Water Rocket#
Author: Bernardo Bahia Monteiro (bbahia@umich.edu)
In this example, we will optimize a water rocket for range and height at the apogee, using design variables that are easily modifiable just before launch: the empty mass, the initial water volume and the launch angle. This example builds on multi-phase cannonball ane is adapted from Optimization of a Water Rocket in OpenMDAO/Dymos [BM20].
Nomenclature#
Symbol |
definition |
---|---|
\(v_\text{out}\) |
water exit speed at the nozzle |
\(A_\text{out}\) |
nozzle area |
\(V_w\) |
water volume in the rocket |
\(p\) |
pressure in the rocket |
\(p_a\) |
ambient pressure |
\(\dot{\,}\) |
time derivative |
\(k\) |
polytropic constant |
\(V_b\) |
internal volume of the rocket |
\(\rho_w\) |
water density |
\(T\) |
thrust |
\(q\) |
dynamic pressure |
\(S\) |
cross sectional area |
\((\cdot)_0\) |
value of \((\cdot)\) at \(t=0\) |
\(t\) |
time |
Problem Formulation#
A natural objective function for a water rocket is the maximum height achieved by the rocket during flight, or the horizontal distance it travels, i.e. its range. The design of a water rocket is somewhat constrained by the soda bottle used as its engine. This means that the volume available for water and air is fixed, the initial launch pressure is limited by the bottle’s strength (since the pressure is directly related to the energy available for the rocket, it is easy to see that it should be as high as possible) and the nozzle throat area is also fixed. Given these manufacturing constraints, the design variables we are left with are the empty mass (it can be easily changed through adding ballast), the water volume at the launch, and the launch angle. With this considerations in mind, a natural formulation for the water rocket problem is
Model#
The water rocket model is divided into three basic components: a water engine, responsible for modelling the fluid dynamics inside the rocket and returning its thrust; the aerodynamics, responsible for calculating the atmospheric drag of the rocket; and the equations of motion, responsible for propagating the rocket’s trajectory in time, using Newton’s laws and the forces provided by the other two components.
In order to integrate these three basic components, some additional interfacing components are necessary: an atmospheric model to provide values of ambient pressure for the water engine and air density to the calculation of the dynamic pressure for the aerodynamic model, and a component that calculates the instantaneous mass of the rocket by summing the water mass with the rocket’s empty mass. The high level layout of this model is shown in below.
atmos
, dynamic_pressure
, aero
and eom
are the same models used in multi-phase cannonball.
The remaining components are discussed below.
Warning
The eom
component has a singularity in the flight path angle derivative when the flight speed is zero.
This happens because the rotational dynamics are not modelled.
This can cause convergence problems if the launch velocity is set to zero or the launch angle is set to \(90^\circ\)
Note
Since the range of altitudes achieved by the water rocket is very small (100m), the air density and pressure are practically constant, thus the use of an atmospheric model is not necessary. However, using it makes it easier to reuse code from [multi-phase cannonball (../multi_phase_cannonball/multi_phase_cannonball.md).
Water engine#
The water engine is modelled by assuming that the air expansion in the rocket follows an adiabatic process and the water flow is incompressible and inviscid, i.e. it follows Bernoulli’s equation. We also make the following simplifying assumptions:
The thrust developed after the water is depleted is negligible
The area inside the bottle is much smaller than the nozzle area
The inertial forces do not affect the fluid dynamics inside the bottle
This simplified modelling can be found in Prusa[@Prusa2000]. A more rigorous formulation, which drops all these simplifying assumptions can be found in Wheeler[@Wheeler2002], Gommes[@Gommes2010], and Barria-Perotti[@BarrioPerotti2010].
The first assumption leads to an underestimation of the rocket performance, since the air left in the bottle after it is out of water is known to generate appreciable thrust[@Thorncroft2009]. This simplified model, however, produces physically meaningful results.
There are two states in this dynamical model, the water volume in the rocket \(V_w\) and the gauge pressure inside the rocket \(p\). The constitutive equations and the N2 diagram showing the model organization are shown below.
Constitutive equations of the water engine model#
Component |
Equation |
---|---|
water_exhaust_speed |
\(v_\text{out} = \sqrt{2(p-p_a)/\rho_w}\) |
water_flow_rate |
\(\dot{V}_w = -v_\text{out} A_\text{out}\) |
pressure_rate |
\(\dot{p} = kp\frac{\dot{V_w}}{(V_b-V_w)}\) |
water_thrust |
\(T = (\rho_w v_\text{out})(v_\text{out}A_\text{out})\) |
import numpy as np
import matplotlib as mpl
import matplotlib.pyplot as plt
import openmdao.api as om
import dymos as dm
class WaterEngine(om.Group):
"""
Computes thrust and water flow for a water.
Simplifications:
- the pressure due to the water column in the non inertial frame (i.e.
under a+g acceleration) is insignificant compared to the air pressure
- the water does not have appreciable speed inside the bottle
"""
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_subsystem(name='water_exhaust_speed',
subsys=_WaterExhaustSpeed(num_nodes=nn),
promotes=['p', 'p_a', 'rho_w'])
self.add_subsystem(name='water_flow_rate',
subsys=_WaterFlowRate(num_nodes=nn),
promotes=['A_out', 'Vdot'])
self.add_subsystem(name='pressure_rate',
subsys=_PressureRate(num_nodes=nn),
promotes=['p', 'k', 'V_b', 'V_w', 'Vdot', 'pdot'])
self.add_subsystem(name='water_thrust',
subsys=_WaterThrust(num_nodes=nn),
promotes=['rho_w', 'A_out', 'F'])
self.connect('water_exhaust_speed.v_out', 'water_flow_rate.v_out')
self.connect('water_exhaust_speed.v_out', 'water_thrust.v_out')
class _WaterExhaustSpeed(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input(name='rho_w', val=1e3*np.ones(nn), desc='water density', units='kg/m**3')
self.add_input(name='p', val=6.5e5*np.ones(nn), desc='air pressure', units='N/m**2') # 5.5bar = 80 psi
self.add_input(name='p_a', val=1.01e5*np.ones(nn), desc='air pressure', units='N/m**2')
self.add_output(name='v_out', shape=(nn,), desc='water exhaust speed', units='m/s')
ar = np.arange(nn)
self.declare_partials(of='*', wrt='*', rows=ar, cols=ar)
def compute(self, inputs, outputs):
p = inputs['p']
p_a = inputs['p_a']
rho_w = inputs['rho_w']
outputs['v_out'] = np.sqrt(2*(p-p_a)/rho_w)
def compute_partials(self, inputs, partials):
p = inputs['p']
p_a = inputs['p_a']
rho_w = inputs['rho_w']
v_out = np.sqrt(2*(p-p_a)/rho_w)
partials['v_out', 'p'] = 1/v_out/rho_w
partials['v_out', 'p_a'] = -1/v_out/rho_w
partials['v_out', 'rho_w'] = dv_outdrho_w = 1/v_out*(-(p-p_a)/rho_w**2)
class _PressureRate(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input(name='p', val=np.ones(nn), desc='air pressure', units='N/m**2')
self.add_input(name='k', val=1.4*np.ones(nn), desc='polytropic coefficient for expansion', units=None)
self.add_input(name='V_b', val=2e-3*np.ones(nn), desc='bottle volume', units='m**3')
self.add_input(name='V_w', val=1e-3*np.ones(nn), desc='water volume', units='m**3')
self.add_input(name='Vdot', shape=(nn,), desc='water flow', units='m**3/s')
self.add_output(name='pdot', shape=(nn,), desc='pressure derivative', units='N/m**2/s')
ar = np.arange(nn)
self.declare_partials(of='*', wrt='*', rows=ar, cols=ar)
def compute(self, inputs, outputs):
p = inputs['p']
k = inputs['k']
V_b = inputs['V_b']
V_w = inputs['V_w']
Vdot = inputs['Vdot']
pdot = p*k*Vdot/(V_b-V_w)
outputs['pdot'] = pdot
def compute_partials(self, inputs, partials):
p = inputs['p']
k = inputs['k']
V_b = inputs['V_b']
V_w = inputs['V_w']
Vdot = inputs['Vdot']
partials['pdot', 'p'] = k*Vdot/(V_b-V_w)
partials['pdot', 'k'] = p*Vdot/(V_b-V_w)
partials['pdot', 'V_b'] = -p*k*Vdot/(V_b-V_w)**2
partials['pdot', 'V_w'] = p*k*Vdot/(V_b-V_w)**2
partials['pdot', 'Vdot'] = p*k/(V_b-V_w)
class _WaterFlowRate(om.ExplicitComponent):
""" Computer water flow rate"""
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input(name='A_out', val=np.ones(nn), desc='nozzle outlet area', units='m**2')
self.add_input(name='v_out', val=np.zeros(nn), desc='water exhaust speed', units='m/s')
self.add_output(name='Vdot', shape=(nn,), desc='water flow', units='m**3/s')
ar = np.arange(nn)
self.declare_partials(of='*', wrt='*', rows=ar, cols=ar)
def compute(self, inputs, outputs):
A_out = inputs['A_out']
v_out = inputs['v_out']
outputs['Vdot'] = -v_out*A_out
def compute_partials(self, inputs, partials):
A_out = inputs['A_out']
v_out = inputs['v_out']
partials['Vdot', 'A_out'] = -v_out
partials['Vdot', 'v_out'] = -A_out
The _MassAdder
component calculates the rocket’s instantaneous mass by
summing the water mass with the rockets empty mass, i.e.
class _MassAdder(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input('m_empty', val=np.zeros(nn), desc='empty mass', units='kg')
self.add_input('V_w', val=1e-3*np.ones(nn), desc='water volume', units='m**3')
self.add_input('rho_w', val=1e3*np.ones(nn), desc="water density", units='kg/m**3')
self.add_output('m', val=np.zeros(nn), desc='total mass', units='kg')
ar = np.arange(nn)
self.declare_partials('*', '*', cols=ar, rows=ar)
def compute(self, inputs, outputs):
outputs['m'] = inputs['m_empty'] + inputs['rho_w']*inputs['V_w']
def compute_partials(self, inputs, jacobian):
jacobian['m', 'm_empty'] = 1
jacobian['m', 'rho_w'] = inputs['V_w']
jacobian['m', 'V_w'] = inputs['rho_w']
Now these components are joined in a single group
display_source('dymos.examples.water_rocket.water_propulsion_ode.WaterPropulsionODE')
class WaterPropulsionODE(om.Group):
def initialize(self):
self.options.declare('num_nodes', types=int)
self.options.declare('ballistic', types=bool, default=False,
desc='If True, neglect propulsion system.')
def setup(self):
nn = self.options['num_nodes']
self.add_subsystem(name='atmos',
subsys=USatm1976Comp(num_nodes=nn))
if not self.options['ballistic']:
self.add_subsystem(name='water_engine',
subsys=WaterEngine(num_nodes=nn),
promotes_inputs=['rho_w'])
self.add_subsystem(name='mass_adder',
subsys=_MassAdder(num_nodes=nn),
promotes_inputs=['rho_w'])
self.add_subsystem(name='dynamic_pressure',
subsys=DynamicPressureComp(num_nodes=nn))
self.add_subsystem(name='aero',
subsys=LiftDragForceComp(num_nodes=nn))
self.add_subsystem(name='eom',
subsys=FlightPathEOM2D(num_nodes=nn))
self.connect('atmos.rho', 'dynamic_pressure.rho')
self.connect('dynamic_pressure.q', 'aero.q')
self.connect('aero.f_drag', 'eom.D')
self.connect('aero.f_lift', 'eom.L')
if not self.options['ballistic']:
self.connect('atmos.pres', 'water_engine.p_a')
self.connect('water_engine.F', 'eom.T')
self.connect('mass_adder.m', 'eom.m')
Phases#
The flight of the water rocket is split in three distinct phases: propelled ascent, ballistic ascent and ballistic descent. If the simplification of no thrust without water were lifted, there would be an extra “air propelled ascent” phase between the propelled ascent and ballistic ascent phases.
Propelled ascent: is the flight phase where the rocket still has water inside, and hence it is producing thrust. The thrust is given by the water engine model, and fed into the flight dynamic equations. It starts at launch and finishes when the water is depleted, i.e. \(V_w=0\).
Ballistic ascent: is the flight phase where the rocket is ascending (\(\gamma>0\)) but produces no thrust. This phase begins at the end of thepropelled ascent phase and ends at the apogee, defined by \(\gamma=0\).
Descent: is the phase where the rocket is descending without thrust. It begins at the end of the ballistic ascent phase and ends with ground impact, i.e. \(h=0\).
def new_propelled_ascent_phase(transcription):
propelled_ascent = dm.Phase(ode_class=WaterPropulsionODE,
transcription=transcription)
# All initial states except flight path angle and water volume are fixed
# Final flight path angle is fixed (we will set it to zero so that the phase ends at apogee)
# Final water volume is fixed (we will set it to zero so that phase ends when bottle empties)
propelled_ascent.set_time_options(
fix_initial=True, duration_bounds=(0.001, 0.5), duration_ref=0.1, units='s')
propelled_ascent.add_state('r', units='m', rate_source='eom.r_dot',
fix_initial=True, fix_final=False, ref=100.0, defect_ref=100.0)
propelled_ascent.add_state('h', units='m', rate_source='eom.h_dot', targets=['atmos.h'],
fix_initial=True, fix_final=False, ref=100.0, defect_ref=100.0)
propelled_ascent.add_state('gam', units='deg', rate_source='eom.gam_dot', targets=['eom.gam'],
fix_initial=False, fix_final=False, lower=0, upper=85.0, ref=90)
propelled_ascent.add_state('v', units='m/s', rate_source='eom.v_dot', targets=['dynamic_pressure.v', 'eom.v'],
fix_initial=True, fix_final=False, ref=100, defect_ref=100, lower=0.01)
propelled_ascent.add_state('p', units='bar', rate_source='water_engine.pdot',
targets=['water_engine.p'], fix_initial=True, fix_final=False,
lower=1.02)
propelled_ascent.add_state('V_w', units='L', rate_source='water_engine.Vdot',
targets=['water_engine.V_w', 'mass_adder.V_w'],
fix_initial=False, fix_final=True, ref=10, defect_ref=10,
lower=0)
propelled_ascent.add_parameter(
'S', targets=['aero.S'], units='m**2')
propelled_ascent.add_parameter(
'm_empty', targets=['mass_adder.m_empty'], units='kg')
propelled_ascent.add_parameter(
'V_b', targets=['water_engine.V_b'], units='m**3')
propelled_ascent.add_timeseries_output('water_engine.F', 'T', units='N')
return propelled_ascent
def new_ballistic_ascent_phase(transcription):
ballistic_ascent = dm.Phase(ode_class=WaterPropulsionODE, transcription=transcription,
ode_init_kwargs={'ballistic': True})
# All initial states are free (they will be linked to the final stages of propelled_ascent).
# Final flight path angle is fixed (we will set it to zero so that the phase ends at apogee)
ballistic_ascent.set_time_options(
fix_initial=False, initial_bounds=(0.001, 1), duration_bounds=(0.001, 10),
duration_ref=1, units='s')
ballistic_ascent.add_state('r', units='m', rate_source='eom.r_dot', fix_initial=False,
fix_final=False, ref=1.0, defect_ref=1.0)
ballistic_ascent.add_state('h', units='m', rate_source='eom.h_dot', targets=['atmos.h'],
fix_initial=False, fix_final=False, ref=1.0)
ballistic_ascent.add_state('gam', units='deg', rate_source='eom.gam_dot', targets=['eom.gam'],
fix_initial=False, fix_final=True, upper=89, ref=90)
ballistic_ascent.add_state('v', units='m/s', rate_source='eom.v_dot',
targets=['dynamic_pressure.v', 'eom.v'], fix_initial=False,
fix_final=False, ref=1.)
ballistic_ascent.add_parameter('S', targets=['aero.S'], units='m**2')
ballistic_ascent.add_parameter('m_empty', targets=['eom.m'], units='kg')
return ballistic_ascent
def new_descent_phase(transcription):
descent = dm.Phase(ode_class=WaterPropulsionODE, transcription=transcription,
ode_init_kwargs={'ballistic': True})
# All initial states and time are free (they will be linked to the final states of ballistic_ascent).
# Final altitude is fixed (we will set it to zero so that the phase ends at ground impact)
descent.set_time_options(initial_bounds=(.5, 100), duration_bounds=(.5, 100),
duration_ref=10, units='s')
descent.add_state('r', units='m', rate_source='eom.r_dot', fix_initial=False, fix_final=False)
descent.add_state('h', units='m', rate_source='eom.h_dot', targets=['atmos.h'],
fix_initial=False, fix_final=True)
descent.add_state('gam', units='deg', rate_source='eom.gam_dot', targets=['eom.gam'],
fix_initial=False, fix_final=False)
descent.add_state('v', units='m/s', rate_source='eom.v_dot',
targets=['dynamic_pressure.v', 'eom.v'],
fix_initial=False, fix_final=False,
ref=1)
descent.add_parameter('S', targets=['aero.S'], units='m**2')
descent.add_parameter('mass', targets=['eom.m'], units='kg')
return descent
Model parameters#
The model requires a few constant parameters. The values used are shown in the following table.
Values for parameters in the water rocket model
Parameter |
Value |
Unit |
Reference |
---|---|---|---|
\(C_D\) |
0.3450 |
- |
|
\(S\) |
\(\pi 106^2/4\) |
\(mm^2\) |
|
\(k\) |
1.2 |
- |
|
\(A_\text{out}\) |
\(\pi22^2/4\) |
\(mm^2\) |
[air13] |
\(V_b\) |
2 |
L |
|
\(\rho_w\) |
1000 |
\(kg/m^3\) |
|
\(p_0\) |
6.5 |
bar |
|
\(v_0\) |
0.1 |
\(m/s\) |
|
\(h_0\) |
0 |
\(m\) |
|
\(r_0\) |
0 |
\(m\) |
Values for the bottle volume \(V_b\), its cross-sectional area \(S\) and the nozzle area \(A_\text{out}\) are determined by the soda bottle that makes the rocket primary structure, and thus are not easily modifiable by the designer. The polytropic coefficient \(k\) is a function of the moist air characteristics inside the rocket. The initial speed \(v_0\) must be set to a value higher than zero, otherwise the flight dynamic equations become singular. This issue arises from the angular dynamics of the rocket not being modelled. The drag coefficient \(C_D\) is sensitive to the aerodynamic design, but can be optimized by a single discipline analysis. The initial pressure \(p_0\) should be maximized in order to obtain the maximum range or height for the rocket. It is limited by the structural properties of the bottle, which are modifiable by the designer, since the bottle needs to be available commercially. Finally, the starting point of the rocket is set to the origin.
Putting it all together#
The different phases must be combined in a single trajectory, and linked in a sequence. Here we also define the design variables.
display_source('dymos.examples.water_rocket.phases.new_water_rocket_trajectory')
def new_water_rocket_trajectory(objective):
tx_prop = dm.Radau(num_segments=50, order=3, compressed=True)
tx_bal = dm.Radau(num_segments=10, order=3, compressed=True)
tx_desc = dm.Radau(num_segments=10, order=3, compressed=True)
traj = dm.Trajectory()
# Add phases to trajectory
propelled_ascent = traj.add_phase('propelled_ascent', new_propelled_ascent_phase(tx_prop))
ballistic_ascent = traj.add_phase('ballistic_ascent', new_ballistic_ascent_phase(tx_bal))
descent = traj.add_phase('descent', new_descent_phase(tx_desc))
# Link phases
traj.link_phases(phases=['propelled_ascent', 'ballistic_ascent'], vars=['*'])
traj.link_phases(phases=['ballistic_ascent', 'descent'], vars=['*'])
# Set objective function
if objective == 'height':
ballistic_ascent.add_objective('h', loc='final', ref=-1.0)
elif objective == 'range':
descent.add_objective('r', loc='final', ref=-100.0)
else:
raise ValueError(f"objective='{objective}' is not defined. Try using 'height' or 'range'")
# Add design parameters to the trajectory.
traj.add_parameter('CD',
targets={'propelled_ascent': ['aero.CD'],
'ballistic_ascent': ['aero.CD'],
'descent': ['aero.CD']},
val=0.3450, units=None, opt=False)
traj.add_parameter('CL',
targets={'propelled_ascent': ['aero.CL'],
'ballistic_ascent': ['aero.CL'],
'descent': ['aero.CL']},
val=0.0, units=None, opt=False)
traj.add_parameter('T',
targets={'ballistic_ascent': ['eom.T'],
'descent': ['eom.T']},
val=0.0, units='N', opt=False)
traj.add_parameter('alpha',
targets={'propelled_ascent': ['eom.alpha'],
'ballistic_ascent': ['eom.alpha'],
'descent': ['eom.alpha']},
val=0.0, units='deg', opt=False)
traj.add_parameter('m_empty', units='kg', val=0.15,
targets={'propelled_ascent': 'm_empty',
'ballistic_ascent': 'm_empty',
'descent': 'mass'},
lower=0.001, upper=1, ref=0.1,
opt=False)
traj.add_parameter('V_b', units='m**3', val=2e-3,
targets={'propelled_ascent': 'V_b'},
opt=False)
traj.add_parameter('S', units='m**2', val=np.pi*106e-3**2/4, opt=False)
traj.add_parameter('A_out', units='m**2', val=np.pi*22e-3**2/4.,
targets={'propelled_ascent': ['water_engine.A_out']},
opt=False)
traj.add_parameter('k', units=None, val=1.2, opt=False,
targets={'propelled_ascent': ['water_engine.k']})
# Make parameter m_empty a design variable
traj.set_parameter_options('m_empty', opt=True)
return traj, {'propelled_ascent': propelled_ascent,
'ballistic_ascent': ballistic_ascent,
'descent': descent}
Helper Functions to Access the Results#
from collections import namedtuple
def summarize_results(water_rocket_problem):
p = water_rocket_problem
Entry = namedtuple('Entry', 'value unit')
summary = {
'Launch angle': Entry(p.get_val('traj.propelled_ascent.timeseries.gam', units='deg')[0, 0], 'deg'),
'Flight angle at end of propulsion': Entry(p.get_val('traj.propelled_ascent.timeseries.gam',
units='deg')[-1, 0], 'deg'),
'Empty mass': Entry(p.get_val('traj.parameters:m_empty', units='kg')[0], 'kg'),
'Water volume': Entry(p.get_val('traj.propelled_ascent.timeseries.V_w', 'L')[0, 0], 'L'),
'Maximum range': Entry(p.get_val('traj.descent.timeseries.r', units='m')[-1, 0], 'm'),
'Maximum height': Entry(p.get_val('traj.ballistic_ascent.timeseries.h', units='m')[-1, 0], 'm'),
'Maximum velocity': Entry(p.get_val('traj.propelled_ascent.timeseries.v', units='m/s')[-1, 0], 'm/s'),
}
return summary
colors={'pa': 'tab:blue', 'ba': 'tab:orange', 'd': 'tab:green'}
def plot_propelled_ascent(p, exp_out):
fig, ax = plt.subplots(2, 2, sharex=True, figsize=(12, 6))
t_imp = p.get_val('traj.propelled_ascent.timeseries.time', 's')
t_exp = exp_out.get_val('traj.propelled_ascent.timeseries.time', 's')
c = colors['pa']
ax[0,0].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.p', 'bar'), '.', color=c)
ax[0,0].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.p', 'bar'), '-', color=c)
ax[0,0].set_ylabel('p (bar)')
ax[0,0].set_ylim(bottom=0)
ax[1,0].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.V_w', 'L'), '.', color=c)
ax[1,0].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.V_w', 'L'), '-', color=c)
ax[1,0].set_ylabel('$V_w$ (L)')
ax[0,1].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.T', 'N'), '.', color=c)
ax[0,1].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.T', 'N'), '-', color=c)
ax[0,1].set_ylabel('T (N)')
ax[0,1].set_ylim(bottom=0)
ax[1,1].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.v', 'm/s'), '.', color=c)
ax[1,1].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.v', 'm/s'), '-', color=c)
ax[1,1].set_ylabel('v (m/s)')
ax[1,1].set_ylim(bottom=0)
ax[1,0].set_xlabel('t (s)')
ax[1,1].set_xlabel('t (s)')
for i in range(4):
ax.ravel()[i].grid(True, alpha=0.2)
fig.tight_layout()
def plot_states(p, exp_out, legend_loc='right', legend_ncol=3):
fig, axes = plt.subplots(nrows=2, ncols=2, figsize=(12, 4), sharex=True)
states = ['r', 'h', 'v', 'gam']
units = ['m', 'm', 'm/s', 'deg']
phases = ['propelled_ascent', 'ballistic_ascent', 'descent']
time_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.time'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.time'),
'descent': p.get_val('traj.descent.timeseries.time')}
time_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.time'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.time'),
'descent': exp_out.get_val('traj.descent.timeseries.time')}
x_imp = {phase: {state: p.get_val(f"traj.{phase}.timeseries.{state}", unit)
for state, unit in zip(states, units)
}
for phase in phases
}
x_exp = {phase: {state: exp_out.get_val(f"traj.{phase}.timeseries.{state}", unit)
for state, unit in zip(states, units)
}
for phase in phases
}
for i, (state, unit) in enumerate(zip(states, units)):
axes.ravel()[i].set_ylabel(f"{state} ({unit})" if state != 'gam' else f'$\gamma$ ({unit})')
axes.ravel()[i].plot(time_imp['propelled_ascent'], x_imp['propelled_ascent'][state], '.', color=colors['pa'])
axes.ravel()[i].plot(time_imp['ballistic_ascent'], x_imp['ballistic_ascent'][state], '.', color=colors['ba'])
axes.ravel()[i].plot(time_imp['descent'], x_imp['descent'][state], '.', color=colors['d'])
h1, = axes.ravel()[i].plot(time_exp['propelled_ascent'], x_exp['propelled_ascent'][state], '-', color=colors['pa'], label='Propelled Ascent')
h2, = axes.ravel()[i].plot(time_exp['ballistic_ascent'], x_exp['ballistic_ascent'][state], '-', color=colors['ba'], label='Ballistic Ascent')
h3, = axes.ravel()[i].plot(time_exp['descent'], x_exp['descent'][state], '-', color=colors['d'], label='Descent')
if state == 'gam':
axes.ravel()[i].yaxis.set_major_locator(mpl.ticker.MaxNLocator(nbins='auto', steps=[1, 1.5, 3, 4.5, 6, 9, 10]))
axes.ravel()[i].set_yticks(np.arange(-90, 91, 45))
axes.ravel()[i].grid(True, alpha=0.2)
axes[1, 0].set_xlabel('t (s)')
axes[1, 1].set_xlabel('t (s)')
plt.figlegend(handles=[h1, h2, h3], loc=legend_loc, ncol=legend_ncol)
fig.tight_layout()
def plot_trajectory(p, exp_out, legend_loc='center right'):
fig, axes = plt.subplots(nrows=1, ncols=1, figsize=(8, 6))
time_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.time'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.time'),
'descent': p.get_val('traj.descent.timeseries.time')}
time_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.time'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.time'),
'descent': exp_out.get_val('traj.descent.timeseries.time')}
r_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.r'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.r'),
'descent': p.get_val('traj.descent.timeseries.r')}
r_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.r'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.r'),
'descent': exp_out.get_val('traj.descent.timeseries.r')}
h_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.h'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.h'),
'descent': p.get_val('traj.descent.timeseries.h')}
h_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.h'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.h'),
'descent': exp_out.get_val('traj.descent.timeseries.h')}
axes.plot(r_imp['propelled_ascent'], h_imp['propelled_ascent'], 'o', color=colors['pa'])
axes.plot(r_imp['ballistic_ascent'], h_imp['ballistic_ascent'], 'o', color=colors['ba'])
axes.plot(r_imp['descent'], h_imp['descent'], 'o', color=colors['d'])
h1, = axes.plot(r_exp['propelled_ascent'], h_exp['propelled_ascent'], '-', color=colors['pa'], label='Propelled Ascent')
h2, = axes.plot(r_exp['ballistic_ascent'], h_exp['ballistic_ascent'], '-', color=colors['ba'], label='Ballistic Ascent')
h3, = axes.plot(r_exp['descent'], h_exp['descent'], '-', color=colors['d'], label='Descent')
axes.set_xlabel('r (m)')
axes.set_ylabel('h (m)')
axes.set_aspect('equal', 'box')
plt.figlegend(handles=[h1, h2, h3], loc=legend_loc)
axes.grid(alpha=0.2)
fig.tight_layout()
Optimizing for Height#
from dymos.examples.water_rocket.phases import new_water_rocket_trajectory, set_sane_initial_guesses
p = om.Problem(model=om.Group())
traj, phases = new_water_rocket_trajectory(objective='height')
traj = p.model.add_subsystem('traj', traj)
p.driver = om.pyOptSparseDriver(optimizer='IPOPT', print_results=False)
p.driver.opt_settings['print_level'] = 4
p.driver.opt_settings['max_iter'] = 1000
p.driver.opt_settings['mu_strategy'] = 'monotone'
p.driver.declare_coloring(tol=1.0E-12)
# Finish Problem Setup
p.model.linear_solver = om.DirectSolver()
p.setup()
set_sane_initial_guesses(p, phases)
dm.run_problem(p, run_driver=True, simulate=True)
summary = summarize_results(p)
for key, entry in summary.items():
print(f'{key}: {entry.value:6.4f} {entry.unit}')
sol_out = om.CaseReader('dymos_solution.db').get_case('final')
sim_out = om.CaseReader('dymos_simulation.db').get_case('final')
--- Constraint Report [traj] ---
--- propelled_ascent ---
None
--- ballistic_ascent ---
None
--- descent ---
None
Model viewer data has already been recorded for Driver.
Model viewer data has already been recorded for Driver.
Full total jacobian was computed 3 times, taking 2.900684 seconds.
Total jacobian shape: (1151, 1153)
Jacobian shape: (1151, 1153) ( 0.67% nonzero)
FWD solves: 20 REV solves: 0
Total colors vs. total size: 20 vs 1153 (98.3% improvement)
Sparsity computed using tolerance: 1e-12
Time to compute sparsity: 2.900684 sec.
Time to compute coloring: 2.978979 sec.
Memory to compute coloring: -19.882812 MB.
List of user-set options:
Name Value used
file_print_level = 5 yes
hessian_approximation = limited-memory yes
linear_solver = mumps yes
max_iter = 1000 yes
mu_strategy = monotone yes
nlp_scaling_method = user-scaling yes
output_file = IPOPT.out yes
print_level = 4 yes
print_user_options = yes yes
sb = yes yes
Total number of variables............................: 1153
variables with only lower bounds: 450
variables with lower and upper bounds: 157
variables with only upper bounds: 30
Total number of equality constraints.................: 1150
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
Number of Iterations....: 61
(scaled) (unscaled)
Objective...............: -5.3408199947708830e+01 -5.3408199947708830e+01
Dual infeasibility......: 5.0610102400738663e-09 5.0610102400738663e-09
Constraint violation....: 2.4745673198978425e-09 2.4745673198978425e-09
Variable bound violation: 9.8625653199846397e-09 9.8625653199846397e-09
Complementarity.........: 2.5059035596800953e-09 2.5059035596800953e-09
Overall NLP error.......: 5.0610102400738663e-09 5.0610102400738663e-09
Number of objective function evaluations = 94
Number of objective gradient evaluations = 62
Number of equality constraint evaluations = 94
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 62
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 0
Total seconds in IPOPT = 10.136
EXIT: Optimal Solution Found.
Simulating trajectory traj
Model viewer data has already been recorded for Driver.
Done simulating trajectory traj
Launch angle: 85.0000 deg
Flight angle at end of propulsion: 84.0887 deg
Empty mass: 0.1449 kg
Water volume: 0.9836 L
Maximum range: 16.0259 m
Maximum height: 53.4082 m
Maximum velocity: 46.1530 m/s
Maximum Height Solution: Propulsive Phase#
plot_propelled_ascent(sol_out, sim_out)
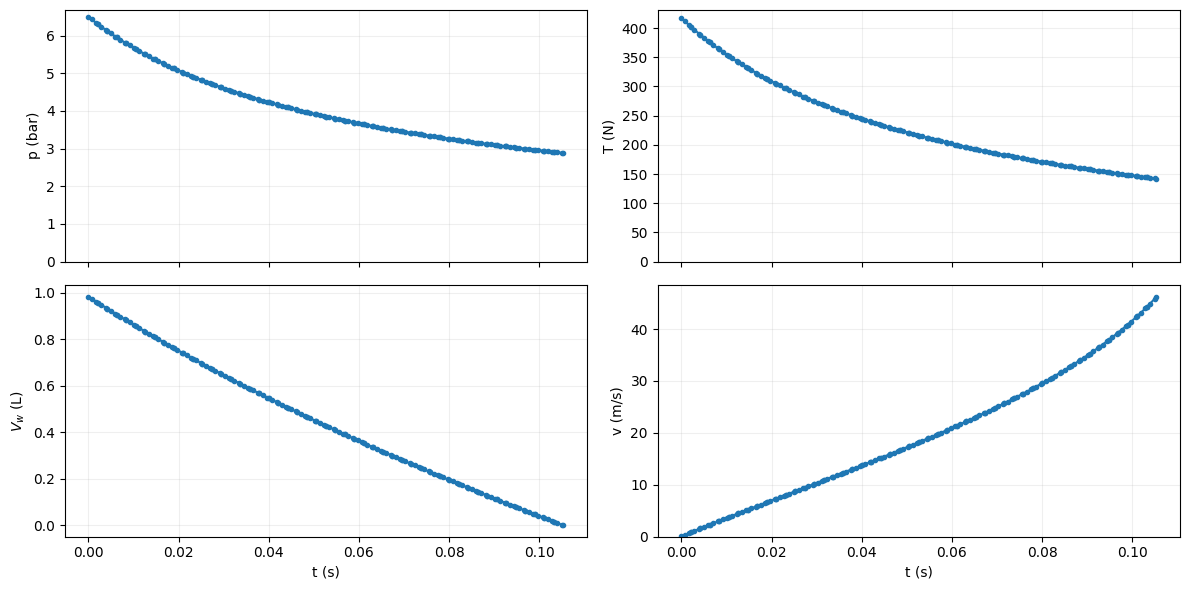
Maximum Height Solution: Height vs. Range#
Note that the equations of motion used here are singular in vertical flight, so the launch angle (the initial flight path angle) was limited to 85 degrees.
plot_trajectory(sol_out, sim_out, legend_loc='center right')
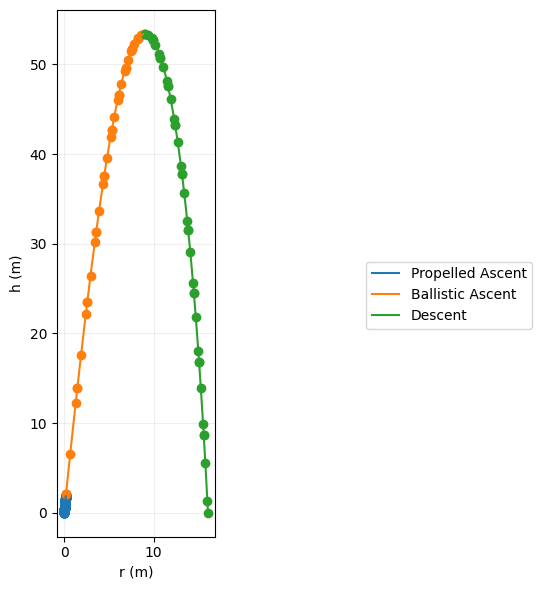
Maximum Height Solution: State History#
plot_states(sol_out, sim_out, legend_loc='lower center', legend_ncol=3)
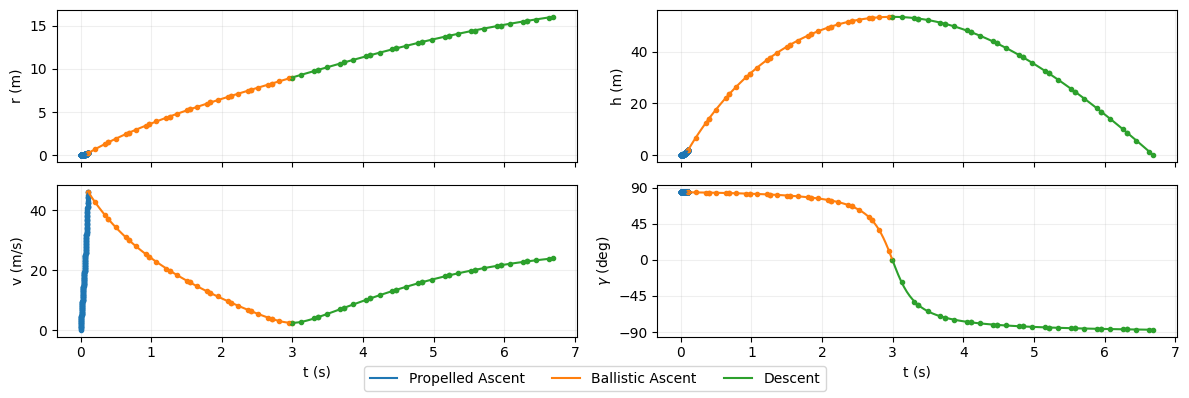
Optimizing for Range#
from dymos.examples.water_rocket.phases import new_water_rocket_trajectory, set_sane_initial_guesses
p = om.Problem(model=om.Group())
traj, phases = new_water_rocket_trajectory(objective='range')
traj = p.model.add_subsystem('traj', traj)
p.driver = om.pyOptSparseDriver(optimizer='IPOPT')
p.driver.opt_settings['print_level'] = 4
p.driver.opt_settings['max_iter'] = 1000
p.driver.opt_settings['mu_strategy'] = 'monotone'
p.driver.declare_coloring(tol=1.0E-12)
# Finish Problem Setup
p.model.linear_solver = om.DirectSolver()
p.setup()
set_sane_initial_guesses(p, phases)
dm.run_problem(p, run_driver=True, simulate=True)
summary = summarize_results(p)
for key, entry in summary.items():
print(f'{key}: {entry.value:6.4f} {entry.unit}')
sol_out = om.CaseReader('dymos_solution.db').get_case('final')
sim_out = om.CaseReader('dymos_simulation.db').get_case('final')
--- Constraint Report [traj] ---
--- propelled_ascent ---
None
--- ballistic_ascent ---
None
--- descent ---
None
/usr/share/miniconda/envs/test/lib/python3.10/site-packages/openmdao/recorders/sqlite_recorder.py:227: UserWarning:The existing case recorder file, dymos_solution.db, is being overwritten.
Model viewer data has already been recorded for Driver.
Model viewer data has already been recorded for Driver.
Full total jacobian was computed 3 times, taking 2.938191 seconds.
Total jacobian shape: (1151, 1153)
Jacobian shape: (1151, 1153) ( 0.67% nonzero)
FWD solves: 20 REV solves: 0
Total colors vs. total size: 20 vs 1153 (98.3% improvement)
Sparsity computed using tolerance: 1e-12
Time to compute sparsity: 2.938191 sec.
Time to compute coloring: 3.010089 sec.
Memory to compute coloring: 0.000000 MB.
List of user-set options:
Name Value used
file_print_level = 5 yes
hessian_approximation = limited-memory yes
linear_solver = mumps yes
max_iter = 1000 yes
mu_strategy = monotone yes
nlp_scaling_method = user-scaling yes
output_file = IPOPT.out yes
print_level = 4 yes
print_user_options = yes yes
sb = yes yes
Total number of variables............................: 1153
variables with only lower bounds: 450
variables with lower and upper bounds: 157
variables with only upper bounds: 30
Total number of equality constraints.................: 1150
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
Number of Iterations....: 48
(scaled) (unscaled)
Objective...............: -8.5111443649844021e-01 -8.5111443649844021e-01
Dual infeasibility......: 5.0864443744323099e-11 5.0864443744323099e-11
Constraint violation....: 5.3436086044157845e-09 5.3436086044157845e-09
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 9.0909090909090931e-10 9.0909090909090931e-10
Overall NLP error.......: 5.3436086044157845e-09 5.3436086044157845e-09
Number of objective function evaluations = 56
Number of objective gradient evaluations = 49
Number of equality constraint evaluations = 56
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 49
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 0
Total seconds in IPOPT = 8.450
EXIT: Optimal Solution Found.
Optimization Problem -- Optimization using pyOpt_sparse
================================================================================
Objective Function: _objfunc
Solution:
--------------------------------------------------------------------------------
Total Time: 8.4514
User Objective Time : 0.2976
User Sensitivity Time : 5.7538
Interface Time : 1.4669
Opt Solver Time: 0.9331
Calls to Objective Function : 56
Calls to Sens Function : 49
Objectives
Index Name Value
0 traj.phases.descent.indep_states.states:r -8.511144E-01
Variables (c - continuous, i - integer, d - discrete)
Index Name Type Lower Bound Value Upper Bound Status
0 traj.parameters:m_empty_0 c 1.000000E-02 1.891010E+00 1.000000E+01
1 traj.ballistic_ascent.t_initial_0 c 1.000000E-03 1.126018E-01 1.000000E+00
2 traj.ballistic_ascent.t_duration_0 c 1.000000E-03 1.928857E+00 1.000000E+01
3 traj.phases.ballistic_ascent.indep_states.states:r_0 c -1.000000E+21 1.608068E+00 1.000000E+21
4 traj.phases.ballistic_ascent.indep_states.states:r_1 c -1.000000E+21 3.795392E+00 1.000000E+21
5 traj.phases.ballistic_ascent.indep_states.states:r_2 c -1.000000E+21 6.718918E+00 1.000000E+21
6 traj.phases.ballistic_ascent.indep_states.states:r_3 c -1.000000E+21 7.622741E+00 1.000000E+21
7 traj.phases.ballistic_ascent.indep_states.states:r_4 c -1.000000E+21 9.655603E+00 1.000000E+21
8 traj.phases.ballistic_ascent.indep_states.states:r_5 c -1.000000E+21 1.238107E+01 1.000000E+21
9 traj.phases.ballistic_ascent.indep_states.states:r_6 c -1.000000E+21 1.322554E+01 1.000000E+21
10 traj.phases.ballistic_ascent.indep_states.states:r_7 c -1.000000E+21 1.512810E+01 1.000000E+21
11 traj.phases.ballistic_ascent.indep_states.states:r_8 c -1.000000E+21 1.768560E+01 1.000000E+21
12 traj.phases.ballistic_ascent.indep_states.states:r_9 c -1.000000E+21 1.847954E+01 1.000000E+21
13 traj.phases.ballistic_ascent.indep_states.states:r_10 c -1.000000E+21 2.027084E+01 1.000000E+21
14 traj.phases.ballistic_ascent.indep_states.states:r_11 c -1.000000E+21 2.268424E+01 1.000000E+21
15 traj.phases.ballistic_ascent.indep_states.states:r_12 c -1.000000E+21 2.343468E+01 1.000000E+21
16 traj.phases.ballistic_ascent.indep_states.states:r_13 c -1.000000E+21 2.512993E+01 1.000000E+21
17 traj.phases.ballistic_ascent.indep_states.states:r_14 c -1.000000E+21 2.741840E+01 1.000000E+21
18 traj.phases.ballistic_ascent.indep_states.states:r_15 c -1.000000E+21 2.813101E+01 1.000000E+21
19 traj.phases.ballistic_ascent.indep_states.states:r_16 c -1.000000E+21 2.974250E+01 1.000000E+21
20 traj.phases.ballistic_ascent.indep_states.states:r_17 c -1.000000E+21 3.192155E+01 1.000000E+21
21 traj.phases.ballistic_ascent.indep_states.states:r_18 c -1.000000E+21 3.260091E+01 1.000000E+21
22 traj.phases.ballistic_ascent.indep_states.states:r_19 c -1.000000E+21 3.413863E+01 1.000000E+21
23 traj.phases.ballistic_ascent.indep_states.states:r_20 c -1.000000E+21 3.622089E+01 1.000000E+21
24 traj.phases.ballistic_ascent.indep_states.states:r_21 c -1.000000E+21 3.687074E+01 1.000000E+21
25 traj.phases.ballistic_ascent.indep_states.states:r_22 c -1.000000E+21 3.834281E+01 1.000000E+21
26 traj.phases.ballistic_ascent.indep_states.states:r_23 c -1.000000E+21 4.033856E+01 1.000000E+21
27 traj.phases.ballistic_ascent.indep_states.states:r_24 c -1.000000E+21 4.096196E+01 1.000000E+21
28 traj.phases.ballistic_ascent.indep_states.states:r_25 c -1.000000E+21 4.237498E+01 1.000000E+21
29 traj.phases.ballistic_ascent.indep_states.states:r_26 c -1.000000E+21 4.429259E+01 1.000000E+21
30 traj.phases.ballistic_ascent.indep_states.states:r_27 c -1.000000E+21 4.489199E+01 1.000000E+21
31 traj.phases.ballistic_ascent.indep_states.states:r_28 c -1.000000E+21 4.625134E+01 1.000000E+21
32 traj.phases.ballistic_ascent.indep_states.states:r_29 c -1.000000E+21 4.809752E+01 1.000000E+21
33 traj.phases.ballistic_ascent.indep_states.states:r_30 c -1.000000E+21 4.867492E+01 1.000000E+21
34 traj.phases.ballistic_ascent.indep_states.states:h_0 c -1.000000E+21 1.304114E+00 1.000000E+21
35 traj.phases.ballistic_ascent.indep_states.states:h_1 c -1.000000E+21 3.014264E+00 1.000000E+21
36 traj.phases.ballistic_ascent.indep_states.states:h_2 c -1.000000E+21 5.225707E+00 1.000000E+21
37 traj.phases.ballistic_ascent.indep_states.states:h_3 c -1.000000E+21 5.891330E+00 1.000000E+21
38 traj.phases.ballistic_ascent.indep_states.states:h_4 c -1.000000E+21 7.355713E+00 1.000000E+21
39 traj.phases.ballistic_ascent.indep_states.states:h_5 c -1.000000E+21 9.244599E+00 1.000000E+21
40 traj.phases.ballistic_ascent.indep_states.states:h_6 c -1.000000E+21 9.811786E+00 1.000000E+21
41 traj.phases.ballistic_ascent.indep_states.states:h_7 c -1.000000E+21 1.105688E+01 1.000000E+21
42 traj.phases.ballistic_ascent.indep_states.states:h_8 c -1.000000E+21 1.265608E+01 1.000000E+21
43 traj.phases.ballistic_ascent.indep_states.states:h_9 c -1.000000E+21 1.313444E+01 1.000000E+21
44 traj.phases.ballistic_ascent.indep_states.states:h_10 c -1.000000E+21 1.418094E+01 1.000000E+21
45 traj.phases.ballistic_ascent.indep_states.states:h_11 c -1.000000E+21 1.551628E+01 1.000000E+21
46 traj.phases.ballistic_ascent.indep_states.states:h_12 c -1.000000E+21 1.591340E+01 1.000000E+21
47 traj.phases.ballistic_ascent.indep_states.states:h_13 c -1.000000E+21 1.677769E+01 1.000000E+21
48 traj.phases.ballistic_ascent.indep_states.states:h_14 c -1.000000E+21 1.786976E+01 1.000000E+21
49 traj.phases.ballistic_ascent.indep_states.states:h_15 c -1.000000E+21 1.819170E+01 1.000000E+21
50 traj.phases.ballistic_ascent.indep_states.states:h_16 c -1.000000E+21 1.888693E+01 1.000000E+21
51 traj.phases.ballistic_ascent.indep_states.states:h_17 c -1.000000E+21 1.975227E+01 1.000000E+21
52 traj.phases.ballistic_ascent.indep_states.states:h_18 c -1.000000E+21 2.000394E+01 1.000000E+21
53 traj.phases.ballistic_ascent.indep_states.states:h_19 c -1.000000E+21 2.054074E+01 1.000000E+21
54 traj.phases.ballistic_ascent.indep_states.states:h_20 c -1.000000E+21 2.119285E+01 1.000000E+21
55 traj.phases.ballistic_ascent.indep_states.states:h_21 c -1.000000E+21 2.137823E+01 1.000000E+21
56 traj.phases.ballistic_ascent.indep_states.states:h_22 c -1.000000E+21 2.176532E+01 1.000000E+21
57 traj.phases.ballistic_ascent.indep_states.states:h_23 c -1.000000E+21 2.221529E+01 1.000000E+21
58 traj.phases.ballistic_ascent.indep_states.states:h_24 c -1.000000E+21 2.233770E+01 1.000000E+21
59 traj.phases.ballistic_ascent.indep_states.states:h_25 c -1.000000E+21 2.258232E+01 1.000000E+21
60 traj.phases.ballistic_ascent.indep_states.states:h_26 c -1.000000E+21 2.283940E+01 1.000000E+21
61 traj.phases.ballistic_ascent.indep_states.states:h_27 c -1.000000E+21 2.290162E+01 1.000000E+21
62 traj.phases.ballistic_ascent.indep_states.states:h_28 c -1.000000E+21 2.300985E+01 1.000000E+21
63 traj.phases.ballistic_ascent.indep_states.states:h_29 c -1.000000E+21 2.308194E+01 1.000000E+21
64 traj.phases.ballistic_ascent.indep_states.states:h_30 c -1.000000E+21 2.308634E+01 1.000000E+21
65 traj.phases.ballistic_ascent.indep_states.states:gam_0 c -1.111111E+19 4.265381E-01 9.888889E-01
66 traj.phases.ballistic_ascent.indep_states.states:gam_1 c -1.111111E+19 4.182287E-01 9.888889E-01
67 traj.phases.ballistic_ascent.indep_states.states:gam_2 c -1.111111E+19 4.060968E-01 9.888889E-01
68 traj.phases.ballistic_ascent.indep_states.states:gam_3 c -1.111111E+19 4.020909E-01 9.888889E-01
69 traj.phases.ballistic_ascent.indep_states.states:gam_4 c -1.111111E+19 3.926065E-01 9.888889E-01
70 traj.phases.ballistic_ascent.indep_states.states:gam_5 c -1.111111E+19 3.787859E-01 9.888889E-01
71 traj.phases.ballistic_ascent.indep_states.states:gam_6 c -1.111111E+19 3.742288E-01 9.888889E-01
72 traj.phases.ballistic_ascent.indep_states.states:gam_7 c -1.111111E+19 3.634519E-01 9.888889E-01
73 traj.phases.ballistic_ascent.indep_states.states:gam_8 c -1.111111E+19 3.477784E-01 9.888889E-01
74 traj.phases.ballistic_ascent.indep_states.states:gam_9 c -1.111111E+19 3.426181E-01 9.888889E-01
75 traj.phases.ballistic_ascent.indep_states.states:gam_10 c -1.111111E+19 3.304299E-01 9.888889E-01
76 traj.phases.ballistic_ascent.indep_states.states:gam_11 c -1.111111E+19 3.127422E-01 9.888889E-01
77 traj.phases.ballistic_ascent.indep_states.states:gam_12 c -1.111111E+19 3.069288E-01 9.888889E-01
78 traj.phases.ballistic_ascent.indep_states.states:gam_13 c -1.111111E+19 2.932186E-01 9.888889E-01
79 traj.phases.ballistic_ascent.indep_states.states:gam_14 c -1.111111E+19 2.733748E-01 9.888889E-01
80 traj.phases.ballistic_ascent.indep_states.states:gam_15 c -1.111111E+19 2.668667E-01 9.888889E-01
81 traj.phases.ballistic_ascent.indep_states.states:gam_16 c -1.111111E+19 2.515467E-01 9.888889E-01
82 traj.phases.ballistic_ascent.indep_states.states:gam_17 c -1.111111E+19 2.294474E-01 9.888889E-01
83 traj.phases.ballistic_ascent.indep_states.states:gam_18 c -1.111111E+19 2.222193E-01 9.888889E-01
84 traj.phases.ballistic_ascent.indep_states.states:gam_19 c -1.111111E+19 2.052451E-01 9.888889E-01
85 traj.phases.ballistic_ascent.indep_states.states:gam_20 c -1.111111E+19 1.808643E-01 9.888889E-01
86 traj.phases.ballistic_ascent.indep_states.states:gam_21 c -1.111111E+19 1.729178E-01 9.888889E-01
87 traj.phases.ballistic_ascent.indep_states.states:gam_22 c -1.111111E+19 1.543129E-01 9.888889E-01
88 traj.phases.ballistic_ascent.indep_states.states:gam_23 c -1.111111E+19 1.277334E-01 9.888889E-01
89 traj.phases.ballistic_ascent.indep_states.states:gam_24 c -1.111111E+19 1.191081E-01 9.888889E-01
90 traj.phases.ballistic_ascent.indep_states.states:gam_25 c -1.111111E+19 9.898934E-02 9.888889E-01
91 traj.phases.ballistic_ascent.indep_states.states:gam_26 c -1.111111E+19 7.043546E-02 9.888889E-01
92 traj.phases.ballistic_ascent.indep_states.states:gam_27 c -1.111111E+19 6.121840E-02 9.888889E-01
93 traj.phases.ballistic_ascent.indep_states.states:gam_28 c -1.111111E+19 3.981478E-02 9.888889E-01
94 traj.phases.ballistic_ascent.indep_states.states:gam_29 c -1.111111E+19 9.670790E-03 9.888889E-01
95 traj.phases.ballistic_ascent.indep_states.states:v_0 c -1.000000E+21 4.131161E+01 1.000000E+21
96 traj.phases.ballistic_ascent.indep_states.states:v_1 c -1.000000E+21 3.978785E+01 1.000000E+21
97 traj.phases.ballistic_ascent.indep_states.states:v_2 c -1.000000E+21 3.782668E+01 1.000000E+21
98 traj.phases.ballistic_ascent.indep_states.states:v_3 c -1.000000E+21 3.723745E+01 1.000000E+21
99 traj.phases.ballistic_ascent.indep_states.states:v_4 c -1.000000E+21 3.594143E+01 1.000000E+21
100 traj.phases.ballistic_ascent.indep_states.states:v_5 c -1.000000E+21 3.426668E+01 1.000000E+21
101 traj.phases.ballistic_ascent.indep_states.states:v_6 c -1.000000E+21 3.376218E+01 1.000000E+21
102 traj.phases.ballistic_ascent.indep_states.states:v_7 c -1.000000E+21 3.265056E+01 1.000000E+21
103 traj.phases.ballistic_ascent.indep_states.states:v_8 c -1.000000E+21 3.121066E+01 1.000000E+21
104 traj.phases.ballistic_ascent.indep_states.states:v_9 c -1.000000E+21 3.077630E+01 1.000000E+21
105 traj.phases.ballistic_ascent.indep_states.states:v_10 c -1.000000E+21 2.981848E+01 1.000000E+21
106 traj.phases.ballistic_ascent.indep_states.states:v_11 c -1.000000E+21 2.857691E+01 1.000000E+21
107 traj.phases.ballistic_ascent.indep_states.states:v_12 c -1.000000E+21 2.820235E+01 1.000000E+21
108 traj.phases.ballistic_ascent.indep_states.states:v_13 c -1.000000E+21 2.737665E+01 1.000000E+21
109 traj.phases.ballistic_ascent.indep_states.states:v_14 c -1.000000E+21 2.630758E+01 1.000000E+21
110 traj.phases.ballistic_ascent.indep_states.states:v_15 c -1.000000E+21 2.598553E+01 1.000000E+21
111 traj.phases.ballistic_ascent.indep_states.states:v_16 c -1.000000E+21 2.527668E+01 1.000000E+21
112 traj.phases.ballistic_ascent.indep_states.states:v_17 c -1.000000E+21 2.436198E+01 1.000000E+21
113 traj.phases.ballistic_ascent.indep_states.states:v_18 c -1.000000E+21 2.408734E+01 1.000000E+21
114 traj.phases.ballistic_ascent.indep_states.states:v_19 c -1.000000E+21 2.348471E+01 1.000000E+21
115 traj.phases.ballistic_ascent.indep_states.states:v_20 c -1.000000E+21 2.271186E+01 1.000000E+21
116 traj.phases.ballistic_ascent.indep_states.states:v_21 c -1.000000E+21 2.248111E+01 1.000000E+21
117 traj.phases.ballistic_ascent.indep_states.states:v_22 c -1.000000E+21 2.197736E+01 1.000000E+21
118 traj.phases.ballistic_ascent.indep_states.states:v_23 c -1.000000E+21 2.133764E+01 1.000000E+21
119 traj.phases.ballistic_ascent.indep_states.states:v_24 c -1.000000E+21 2.114831E+01 1.000000E+21
120 traj.phases.ballistic_ascent.indep_states.states:v_25 c -1.000000E+21 2.073825E+01 1.000000E+21
121 traj.phases.ballistic_ascent.indep_states.states:v_26 c -1.000000E+21 2.022528E+01 1.000000E+21
122 traj.phases.ballistic_ascent.indep_states.states:v_27 c -1.000000E+21 2.007551E+01 1.000000E+21
123 traj.phases.ballistic_ascent.indep_states.states:v_28 c -1.000000E+21 1.975501E+01 1.000000E+21
124 traj.phases.ballistic_ascent.indep_states.states:v_29 c -1.000000E+21 1.936335E+01 1.000000E+21
125 traj.phases.ballistic_ascent.indep_states.states:v_30 c -1.000000E+21 1.925142E+01 1.000000E+21
126 traj.descent.t_initial_0 c 5.000000E-01 2.041459E+00 1.000000E+02
127 traj.descent.t_duration_0 c 5.000000E-02 2.327821E-01 1.000000E+01
128 traj.phases.descent.indep_states.states:r_0 c -1.000000E+21 4.867492E+01 1.000000E+21
129 traj.phases.descent.indep_states.states:r_1 c -1.000000E+21 5.025371E+01 1.000000E+21
130 traj.phases.descent.indep_states.states:r_2 c -1.000000E+21 5.239256E+01 1.000000E+21
131 traj.phases.descent.indep_states.states:r_3 c -1.000000E+21 5.306019E+01 1.000000E+21
132 traj.phases.descent.indep_states.states:r_4 c -1.000000E+21 5.457254E+01 1.000000E+21
133 traj.phases.descent.indep_states.states:r_5 c -1.000000E+21 5.662246E+01 1.000000E+21
134 traj.phases.descent.indep_states.states:r_6 c -1.000000E+21 5.726254E+01 1.000000E+21
135 traj.phases.descent.indep_states.states:r_7 c -1.000000E+21 5.871277E+01 1.000000E+21
136 traj.phases.descent.indep_states.states:r_8 c -1.000000E+21 6.067895E+01 1.000000E+21
137 traj.phases.descent.indep_states.states:r_9 c -1.000000E+21 6.129295E+01 1.000000E+21
138 traj.phases.descent.indep_states.states:r_10 c -1.000000E+21 6.268412E+01 1.000000E+21
139 traj.phases.descent.indep_states.states:r_11 c -1.000000E+21 6.457019E+01 1.000000E+21
140 traj.phases.descent.indep_states.states:r_12 c -1.000000E+21 6.515912E+01 1.000000E+21
141 traj.phases.descent.indep_states.states:r_13 c -1.000000E+21 6.649335E+01 1.000000E+21
142 traj.phases.descent.indep_states.states:r_14 c -1.000000E+21 6.830175E+01 1.000000E+21
143 traj.phases.descent.indep_states.states:r_15 c -1.000000E+21 6.886630E+01 1.000000E+21
144 traj.phases.descent.indep_states.states:r_16 c -1.000000E+21 7.014499E+01 1.000000E+21
145 traj.phases.descent.indep_states.states:r_17 c -1.000000E+21 7.187738E+01 1.000000E+21
146 traj.phases.descent.indep_states.states:r_18 c -1.000000E+21 7.241799E+01 1.000000E+21
147 traj.phases.descent.indep_states.states:r_19 c -1.000000E+21 7.364210E+01 1.000000E+21
148 traj.phases.descent.indep_states.states:r_20 c -1.000000E+21 7.529959E+01 1.000000E+21
149 traj.phases.descent.indep_states.states:r_21 c -1.000000E+21 7.581660E+01 1.000000E+21
150 traj.phases.descent.indep_states.states:r_22 c -1.000000E+21 7.698681E+01 1.000000E+21
151 traj.phases.descent.indep_states.states:r_23 c -1.000000E+21 7.857028E+01 1.000000E+21
152 traj.phases.descent.indep_states.states:r_24 c -1.000000E+21 7.906394E+01 1.000000E+21
153 traj.phases.descent.indep_states.states:r_25 c -1.000000E+21 8.018083E+01 1.000000E+21
154 traj.phases.descent.indep_states.states:r_26 c -1.000000E+21 8.169107E+01 1.000000E+21
155 traj.phases.descent.indep_states.states:r_27 c -1.000000E+21 8.216164E+01 1.000000E+21
156 traj.phases.descent.indep_states.states:r_28 c -1.000000E+21 8.322581E+01 1.000000E+21
157 traj.phases.descent.indep_states.states:r_29 c -1.000000E+21 8.466368E+01 1.000000E+21
158 traj.phases.descent.indep_states.states:r_30 c -1.000000E+21 8.511144E+01 1.000000E+21
159 traj.phases.descent.indep_states.states:h_0 c -1.000000E+21 2.308634E+01 1.000000E+21
160 traj.phases.descent.indep_states.states:h_1 c -1.000000E+21 2.305302E+01 1.000000E+21
161 traj.phases.descent.indep_states.states:h_2 c -1.000000E+21 2.289894E+01 1.000000E+21
162 traj.phases.descent.indep_states.states:h_3 c -1.000000E+21 2.282443E+01 1.000000E+21
163 traj.phases.descent.indep_states.states:h_4 c -1.000000E+21 2.260781E+01 1.000000E+21
164 traj.phases.descent.indep_states.states:h_5 c -1.000000E+21 2.220523E+01 1.000000E+21
165 traj.phases.descent.indep_states.states:h_6 c -1.000000E+21 2.205310E+01 1.000000E+21
166 traj.phases.descent.indep_states.states:h_7 c -1.000000E+21 2.166060E+01 1.000000E+21
167 traj.phases.descent.indep_states.states:h_8 c -1.000000E+21 2.101948E+01 1.000000E+21
168 traj.phases.descent.indep_states.states:h_9 c -1.000000E+21 2.079285E+01 1.000000E+21
169 traj.phases.descent.indep_states.states:h_10 c -1.000000E+21 2.023152E+01 1.000000E+21
170 traj.phases.descent.indep_states.states:h_11 c -1.000000E+21 1.936154E+01 1.000000E+21
171 traj.phases.descent.indep_states.states:h_12 c -1.000000E+21 1.906346E+01 1.000000E+21
172 traj.phases.descent.indep_states.states:h_13 c -1.000000E+21 1.834033E+01 1.000000E+21
173 traj.phases.descent.indep_states.states:h_14 c -1.000000E+21 1.725126E+01 1.000000E+21
174 traj.phases.descent.indep_states.states:h_15 c -1.000000E+21 1.688485E+01 1.000000E+21
175 traj.phases.descent.indep_states.states:h_16 c -1.000000E+21 1.600712E+01 1.000000E+21
176 traj.phases.descent.indep_states.states:h_17 c -1.000000E+21 1.470904E+01 1.000000E+21
177 traj.phases.descent.indep_states.states:h_18 c -1.000000E+21 1.427753E+01 1.000000E+21
178 traj.phases.descent.indep_states.states:h_19 c -1.000000E+21 1.325268E+01 1.000000E+21
179 traj.phases.descent.indep_states.states:h_20 c -1.000000E+21 1.175609E+01 1.000000E+21
180 traj.phases.descent.indep_states.states:h_21 c -1.000000E+21 1.126286E+01 1.000000E+21
181 traj.phases.descent.indep_states.states:h_22 c -1.000000E+21 1.009866E+01 1.000000E+21
182 traj.phases.descent.indep_states.states:h_23 c -1.000000E+21 8.414476E+00 1.000000E+21
183 traj.phases.descent.indep_states.states:h_24 c -1.000000E+21 7.863012E+00 1.000000E+21
184 traj.phases.descent.indep_states.states:h_25 c -1.000000E+21 6.567552E+00 1.000000E+21
185 traj.phases.descent.indep_states.states:h_26 c -1.000000E+21 4.707031E+00 1.000000E+21
186 traj.phases.descent.indep_states.states:h_27 c -1.000000E+21 4.100925E+00 1.000000E+21
187 traj.phases.descent.indep_states.states:h_28 c -1.000000E+21 2.682463E+00 1.000000E+21
188 traj.phases.descent.indep_states.states:h_29 c -1.000000E+21 6.570980E-01 1.000000E+21
189 traj.phases.descent.indep_states.states:gam_0 c -1.000000E+21 5.708043E-29 1.000000E+21
190 traj.phases.descent.indep_states.states:gam_1 c -1.000000E+21 -2.429701E+00 1.000000E+21
191 traj.phases.descent.indep_states.states:gam_2 c -1.000000E+21 -5.827477E+00 1.000000E+21
192 traj.phases.descent.indep_states.states:gam_3 c -1.000000E+21 -6.910356E+00 1.000000E+21
193 traj.phases.descent.indep_states.states:gam_4 c -1.000000E+21 -9.396827E+00 1.000000E+21
194 traj.phases.descent.indep_states.states:gam_5 c -1.000000E+21 -1.282805E+01 1.000000E+21
195 traj.phases.descent.indep_states.states:gam_6 c -1.000000E+21 -1.391041E+01 1.000000E+21
196 traj.phases.descent.indep_states.states:gam_7 c -1.000000E+21 -1.637595E+01 1.000000E+21
197 traj.phases.descent.indep_states.states:gam_8 c -1.000000E+21 -1.973435E+01 1.000000E+21
198 traj.phases.descent.indep_states.states:gam_9 c -1.000000E+21 -2.078346E+01 1.000000E+21
199 traj.phases.descent.indep_states.states:gam_10 c -1.000000E+21 -2.315574E+01 1.000000E+21
200 traj.phases.descent.indep_states.states:gam_11 c -1.000000E+21 -2.634973E+01 1.000000E+21
201 traj.phases.descent.indep_states.states:gam_12 c -1.000000E+21 -2.733906E+01 1.000000E+21
202 traj.phases.descent.indep_states.states:gam_13 c -1.000000E+21 -2.956232E+01 1.000000E+21
203 traj.phases.descent.indep_states.states:gam_14 c -1.000000E+21 -3.252723E+01 1.000000E+21
204 traj.phases.descent.indep_states.states:gam_15 c -1.000000E+21 -3.343943E+01 1.000000E+21
205 traj.phases.descent.indep_states.states:gam_16 c -1.000000E+21 -3.547955E+01 1.000000E+21
206 traj.phases.descent.indep_states.states:gam_17 c -1.000000E+21 -3.818075E+01 1.000000E+21
207 traj.phases.descent.indep_states.states:gam_18 c -1.000000E+21 -3.900775E+01 1.000000E+21
208 traj.phases.descent.indep_states.states:gam_19 c -1.000000E+21 -4.085105E+01 1.000000E+21
209 traj.phases.descent.indep_states.states:gam_20 c -1.000000E+21 -4.327967E+01 1.000000E+21
210 traj.phases.descent.indep_states.states:gam_21 c -1.000000E+21 -4.402083E+01 1.000000E+21
211 traj.phases.descent.indep_states.states:gam_22 c -1.000000E+21 -4.566922E+01 1.000000E+21
212 traj.phases.descent.indep_states.states:gam_23 c -1.000000E+21 -4.783455E+01 1.000000E+21
213 traj.phases.descent.indep_states.states:gam_24 c -1.000000E+21 -4.849415E+01 1.000000E+21
214 traj.phases.descent.indep_states.states:gam_25 c -1.000000E+21 -4.995942E+01 1.000000E+21
215 traj.phases.descent.indep_states.states:gam_26 c -1.000000E+21 -5.188134E+01 1.000000E+21
216 traj.phases.descent.indep_states.states:gam_27 c -1.000000E+21 -5.246633E+01 1.000000E+21
217 traj.phases.descent.indep_states.states:gam_28 c -1.000000E+21 -5.376530E+01 1.000000E+21
218 traj.phases.descent.indep_states.states:gam_29 c -1.000000E+21 -5.546843E+01 1.000000E+21
219 traj.phases.descent.indep_states.states:gam_30 c -1.000000E+21 -5.598681E+01 1.000000E+21
220 traj.phases.descent.indep_states.states:v_0 c -1.000000E+21 1.925142E+01 1.000000E+21
221 traj.phases.descent.indep_states.states:v_1 c -1.000000E+21 1.897161E+01 1.000000E+21
222 traj.phases.descent.indep_states.states:v_2 c -1.000000E+21 1.865510E+01 1.000000E+21
223 traj.phases.descent.indep_states.states:v_3 c -1.000000E+21 1.857132E+01 1.000000E+21
224 traj.phases.descent.indep_states.states:v_4 c -1.000000E+21 1.840830E+01 1.000000E+21
225 traj.phases.descent.indep_states.states:v_5 c -1.000000E+21 1.824708E+01 1.000000E+21
226 traj.phases.descent.indep_states.states:v_6 c -1.000000E+21 1.821090E+01 1.000000E+21
227 traj.phases.descent.indep_states.states:v_7 c -1.000000E+21 1.815382E+01 1.000000E+21
228 traj.phases.descent.indep_states.states:v_8 c -1.000000E+21 1.813124E+01 1.000000E+21
229 traj.phases.descent.indep_states.states:v_9 c -1.000000E+21 1.813699E+01 1.000000E+21
230 traj.phases.descent.indep_states.states:v_10 c -1.000000E+21 1.817219E+01 1.000000E+21
231 traj.phases.descent.indep_states.states:v_11 c -1.000000E+21 1.826804E+01 1.000000E+21
232 traj.phases.descent.indep_states.states:v_12 c -1.000000E+21 1.830905E+01 1.000000E+21
233 traj.phases.descent.indep_states.states:v_13 c -1.000000E+21 1.842085E+01 1.000000E+21
234 traj.phases.descent.indep_states.states:v_14 c -1.000000E+21 1.861287E+01 1.000000E+21
235 traj.phases.descent.indep_states.states:v_15 c -1.000000E+21 1.868199E+01 1.000000E+21
236 traj.phases.descent.indep_states.states:v_16 c -1.000000E+21 1.885401E+01 1.000000E+21
237 traj.phases.descent.indep_states.states:v_17 c -1.000000E+21 1.911976E+01 1.000000E+21
238 traj.phases.descent.indep_states.states:v_18 c -1.000000E+21 1.921000E+01 1.000000E+21
239 traj.phases.descent.indep_states.states:v_19 c -1.000000E+21 1.942649E+01 1.000000E+21
240 traj.phases.descent.indep_states.states:v_20 c -1.000000E+21 1.974502E+01 1.000000E+21
241 traj.phases.descent.indep_states.states:v_21 c -1.000000E+21 1.984997E+01 1.000000E+21
242 traj.phases.descent.indep_states.states:v_22 c -1.000000E+21 2.009674E+01 1.000000E+21
243 traj.phases.descent.indep_states.states:v_23 c -1.000000E+21 2.044963E+01 1.000000E+21
244 traj.phases.descent.indep_states.states:v_24 c -1.000000E+21 2.056377E+01 1.000000E+21
245 traj.phases.descent.indep_states.states:v_25 c -1.000000E+21 2.082870E+01 1.000000E+21
246 traj.phases.descent.indep_states.states:v_26 c -1.000000E+21 2.120052E+01 1.000000E+21
247 traj.phases.descent.indep_states.states:v_27 c -1.000000E+21 2.131927E+01 1.000000E+21
248 traj.phases.descent.indep_states.states:v_28 c -1.000000E+21 2.159246E+01 1.000000E+21
249 traj.phases.descent.indep_states.states:v_29 c -1.000000E+21 2.197079E+01 1.000000E+21
250 traj.phases.descent.indep_states.states:v_30 c -1.000000E+21 2.209052E+01 1.000000E+21
251 traj.propelled_ascent.t_duration_0 c 1.000000E-02 1.126018E+00 5.000000E+00
252 traj.phases.propelled_ascent.indep_states.states:r_0 c -1.000000E+19 1.331346E-06 1.000000E+19
253 traj.phases.propelled_ascent.indep_states.states:r_1 c -1.000000E+19 5.718551E-06 1.000000E+19
254 traj.phases.propelled_ascent.indep_states.states:r_2 c -1.000000E+19 7.723000E-06 1.000000E+19
255 traj.phases.propelled_ascent.indep_states.states:r_3 c -1.000000E+19 1.344992E-05 1.000000E+19
256 traj.phases.propelled_ascent.indep_states.states:r_4 c -1.000000E+19 2.392361E-05 1.000000E+19
257 traj.phases.propelled_ascent.indep_states.states:r_5 c -1.000000E+19 2.785918E-05 1.000000E+19
258 traj.phases.propelled_ascent.indep_states.states:r_6 c -1.000000E+19 3.799366E-05 1.000000E+19
259 traj.phases.propelled_ascent.indep_states.states:r_7 c -1.000000E+19 5.453721E-05 1.000000E+19
260 traj.phases.propelled_ascent.indep_states.states:r_8 c -1.000000E+19 6.039037E-05 1.000000E+19
261 traj.phases.propelled_ascent.indep_states.states:r_9 c -1.000000E+19 7.490885E-05 1.000000E+19
262 traj.phases.propelled_ascent.indep_states.states:r_10 c -1.000000E+19 9.748469E-05 1.000000E+19
263 traj.phases.propelled_ascent.indep_states.states:r_11 c -1.000000E+19 1.052429E-04 1.000000E+19
264 traj.phases.propelled_ascent.indep_states.states:r_12 c -1.000000E+19 1.241161E-04 1.000000E+19
265 traj.phases.propelled_ascent.indep_states.states:r_13 c -1.000000E+19 1.526833E-04 1.000000E+19
266 traj.phases.propelled_ascent.indep_states.states:r_14 c -1.000000E+19 1.623336E-04 1.000000E+19
267 traj.phases.propelled_ascent.indep_states.states:r_15 c -1.000000E+19 1.855322E-04 1.000000E+19
268 traj.phases.propelled_ascent.indep_states.states:r_16 c -1.000000E+19 2.200515E-04 1.000000E+19
269 traj.phases.propelled_ascent.indep_states.states:r_17 c -1.000000E+19 2.315818E-04 1.000000E+19
270 traj.phases.propelled_ascent.indep_states.states:r_18 c -1.000000E+19 2.590789E-04 1.000000E+19
271 traj.phases.propelled_ascent.indep_states.states:r_19 c -1.000000E+19 2.995145E-04 1.000000E+19
272 traj.phases.propelled_ascent.indep_states.states:r_20 c -1.000000E+19 3.129139E-04 1.000000E+19
273 traj.phases.propelled_ascent.indep_states.states:r_21 c -1.000000E+19 3.446853E-04 1.000000E+19
274 traj.phases.propelled_ascent.indep_states.states:r_22 c -1.000000E+19 3.910061E-04 1.000000E+19
275 traj.phases.propelled_ascent.indep_states.states:r_23 c -1.000000E+19 4.062653E-04 1.000000E+19
276 traj.phases.propelled_ascent.indep_states.states:r_24 c -1.000000E+19 4.422904E-04 1.000000E+19
277 traj.phases.propelled_ascent.indep_states.states:r_25 c -1.000000E+19 4.944700E-04 1.000000E+19
278 traj.phases.propelled_ascent.indep_states.states:r_26 c -1.000000E+19 5.115812E-04 1.000000E+19
279 traj.phases.propelled_ascent.indep_states.states:r_27 c -1.000000E+19 5.518432E-04 1.000000E+19
280 traj.phases.propelled_ascent.indep_states.states:r_28 c -1.000000E+19 6.098604E-04 1.000000E+19
281 traj.phases.propelled_ascent.indep_states.states:r_29 c -1.000000E+19 6.288172E-04 1.000000E+19
282 traj.phases.propelled_ascent.indep_states.states:r_30 c -1.000000E+19 6.733027E-04 1.000000E+19
283 traj.phases.propelled_ascent.indep_states.states:r_31 c -1.000000E+19 7.371414E-04 1.000000E+19
284 traj.phases.propelled_ascent.indep_states.states:r_32 c -1.000000E+19 7.579394E-04 1.000000E+19
285 traj.phases.propelled_ascent.indep_states.states:r_33 c -1.000000E+19 8.066389E-04 1.000000E+19
286 traj.phases.propelled_ascent.indep_states.states:r_34 c -1.000000E+19 8.762880E-04 1.000000E+19
287 traj.phases.propelled_ascent.indep_states.states:r_35 c -1.000000E+19 8.989242E-04 1.000000E+19
288 traj.phases.propelled_ascent.indep_states.states:r_36 c -1.000000E+19 9.518318E-04 1.000000E+19
289 traj.phases.propelled_ascent.indep_states.states:r_37 c -1.000000E+19 1.027285E-03 1.000000E+19
290 traj.phases.propelled_ascent.indep_states.states:r_38 c -1.000000E+19 1.051758E-03 1.000000E+19
291 traj.phases.propelled_ascent.indep_states.states:r_39 c -1.000000E+19 1.108872E-03 1.000000E+19
292 traj.phases.propelled_ascent.indep_states.states:r_40 c -1.000000E+19 1.190129E-03 1.000000E+19
293 traj.phases.propelled_ascent.indep_states.states:r_41 c -1.000000E+19 1.216439E-03 1.000000E+19
294 traj.phases.propelled_ascent.indep_states.states:r_42 c -1.000000E+19 1.277760E-03 1.000000E+19
295 traj.phases.propelled_ascent.indep_states.states:r_43 c -1.000000E+19 1.364825E-03 1.000000E+19
296 traj.phases.propelled_ascent.indep_states.states:r_44 c -1.000000E+19 1.392974E-03 1.000000E+19
297 traj.phases.propelled_ascent.indep_states.states:r_45 c -1.000000E+19 1.458507E-03 1.000000E+19
298 traj.phases.propelled_ascent.indep_states.states:r_46 c -1.000000E+19 1.551389E-03 1.000000E+19
299 traj.phases.propelled_ascent.indep_states.states:r_47 c -1.000000E+19 1.581380E-03 1.000000E+19
300 traj.phases.propelled_ascent.indep_states.states:r_48 c -1.000000E+19 1.651135E-03 1.000000E+19
301 traj.phases.propelled_ascent.indep_states.states:r_49 c -1.000000E+19 1.749848E-03 1.000000E+19
302 traj.phases.propelled_ascent.indep_states.states:r_50 c -1.000000E+19 1.781686E-03 1.000000E+19
303 traj.phases.propelled_ascent.indep_states.states:r_51 c -1.000000E+19 1.855675E-03 1.000000E+19
304 traj.phases.propelled_ascent.indep_states.states:r_52 c -1.000000E+19 1.960238E-03 1.000000E+19
305 traj.phases.propelled_ascent.indep_states.states:r_53 c -1.000000E+19 1.993931E-03 1.000000E+19
306 traj.phases.propelled_ascent.indep_states.states:r_54 c -1.000000E+19 2.072169E-03 1.000000E+19
307 traj.phases.propelled_ascent.indep_states.states:r_55 c -1.000000E+19 2.182607E-03 1.000000E+19
308 traj.phases.propelled_ascent.indep_states.states:r_56 c -1.000000E+19 2.218162E-03 1.000000E+19
309 traj.phases.propelled_ascent.indep_states.states:r_57 c -1.000000E+19 2.300670E-03 1.000000E+19
310 traj.phases.propelled_ascent.indep_states.states:r_58 c -1.000000E+19 2.417014E-03 1.000000E+19
311 traj.phases.propelled_ascent.indep_states.states:r_59 c -1.000000E+19 2.454441E-03 1.000000E+19
312 traj.phases.propelled_ascent.indep_states.states:r_60 c -1.000000E+19 2.541242E-03 1.000000E+19
313 traj.phases.propelled_ascent.indep_states.states:r_61 c -1.000000E+19 2.663526E-03 1.000000E+19
314 traj.phases.propelled_ascent.indep_states.states:r_62 c -1.000000E+19 2.702837E-03 1.000000E+19
315 traj.phases.propelled_ascent.indep_states.states:r_63 c -1.000000E+19 2.793960E-03 1.000000E+19
316 traj.phases.propelled_ascent.indep_states.states:r_64 c -1.000000E+19 2.922223E-03 1.000000E+19
317 traj.phases.propelled_ascent.indep_states.states:r_65 c -1.000000E+19 2.963432E-03 1.000000E+19
318 traj.phases.propelled_ascent.indep_states.states:r_66 c -1.000000E+19 3.058908E-03 1.000000E+19
319 traj.phases.propelled_ascent.indep_states.states:r_67 c -1.000000E+19 3.193198E-03 1.000000E+19
320 traj.phases.propelled_ascent.indep_states.states:r_68 c -1.000000E+19 3.236319E-03 1.000000E+19
321 traj.phases.propelled_ascent.indep_states.states:r_69 c -1.000000E+19 3.336185E-03 1.000000E+19
322 traj.phases.propelled_ascent.indep_states.states:r_70 c -1.000000E+19 3.476554E-03 1.000000E+19
323 traj.phases.propelled_ascent.indep_states.states:r_71 c -1.000000E+19 3.521604E-03 1.000000E+19
324 traj.phases.propelled_ascent.indep_states.states:r_72 c -1.000000E+19 3.625899E-03 1.000000E+19
325 traj.phases.propelled_ascent.indep_states.states:r_73 c -1.000000E+19 3.772406E-03 1.000000E+19
326 traj.phases.propelled_ascent.indep_states.states:r_74 c -1.000000E+19 3.819405E-03 1.000000E+19
327 traj.phases.propelled_ascent.indep_states.states:r_75 c -1.000000E+19 3.928175E-03 1.000000E+19
328 traj.phases.propelled_ascent.indep_states.states:r_76 c -1.000000E+19 4.080884E-03 1.000000E+19
329 traj.phases.propelled_ascent.indep_states.states:r_77 c -1.000000E+19 4.129853E-03 1.000000E+19
330 traj.phases.propelled_ascent.indep_states.states:r_78 c -1.000000E+19 4.243147E-03 1.000000E+19
331 traj.phases.propelled_ascent.indep_states.states:r_79 c -1.000000E+19 4.402129E-03 1.000000E+19
332 traj.phases.propelled_ascent.indep_states.states:r_80 c -1.000000E+19 4.453092E-03 1.000000E+19
333 traj.phases.propelled_ascent.indep_states.states:r_81 c -1.000000E+19 4.570965E-03 1.000000E+19
334 traj.phases.propelled_ascent.indep_states.states:r_82 c -1.000000E+19 4.736300E-03 1.000000E+19
335 traj.phases.propelled_ascent.indep_states.states:r_83 c -1.000000E+19 4.789282E-03 1.000000E+19
336 traj.phases.propelled_ascent.indep_states.states:r_84 c -1.000000E+19 4.911794E-03 1.000000E+19
337 traj.phases.propelled_ascent.indep_states.states:r_85 c -1.000000E+19 5.083567E-03 1.000000E+19
338 traj.phases.propelled_ascent.indep_states.states:r_86 c -1.000000E+19 5.138596E-03 1.000000E+19
339 traj.phases.propelled_ascent.indep_states.states:r_87 c -1.000000E+19 5.265814E-03 1.000000E+19
340 traj.phases.propelled_ascent.indep_states.states:r_88 c -1.000000E+19 5.444121E-03 1.000000E+19
341 traj.phases.propelled_ascent.indep_states.states:r_89 c -1.000000E+19 5.501228E-03 1.000000E+19
342 traj.phases.propelled_ascent.indep_states.states:r_90 c -1.000000E+19 5.633222E-03 1.000000E+19
343 traj.phases.propelled_ascent.indep_states.states:r_91 c -1.000000E+19 5.818166E-03 1.000000E+19
344 traj.phases.propelled_ascent.indep_states.states:r_92 c -1.000000E+19 5.877384E-03 1.000000E+19
345 traj.phases.propelled_ascent.indep_states.states:r_93 c -1.000000E+19 6.014235E-03 1.000000E+19
346 traj.phases.propelled_ascent.indep_states.states:r_94 c -1.000000E+19 6.205928E-03 1.000000E+19
347 traj.phases.propelled_ascent.indep_states.states:r_95 c -1.000000E+19 6.267294E-03 1.000000E+19
348 traj.phases.propelled_ascent.indep_states.states:r_96 c -1.000000E+19 6.409087E-03 1.000000E+19
349 traj.phases.propelled_ascent.indep_states.states:r_97 c -1.000000E+19 6.607652E-03 1.000000E+19
350 traj.phases.propelled_ascent.indep_states.states:r_98 c -1.000000E+19 6.671206E-03 1.000000E+19
351 traj.phases.propelled_ascent.indep_states.states:r_99 c -1.000000E+19 6.818035E-03 1.000000E+19
352 traj.phases.propelled_ascent.indep_states.states:r_100 c -1.000000E+19 7.023605E-03 1.000000E+19
353 traj.phases.propelled_ascent.indep_states.states:r_101 c -1.000000E+19 7.089391E-03 1.000000E+19
354 traj.phases.propelled_ascent.indep_states.states:r_102 c -1.000000E+19 7.241357E-03 1.000000E+19
355 traj.phases.propelled_ascent.indep_states.states:r_103 c -1.000000E+19 7.454079E-03 1.000000E+19
356 traj.phases.propelled_ascent.indep_states.states:r_104 c -1.000000E+19 7.522145E-03 1.000000E+19
357 traj.phases.propelled_ascent.indep_states.states:r_105 c -1.000000E+19 7.679359E-03 1.000000E+19
358 traj.phases.propelled_ascent.indep_states.states:r_106 c -1.000000E+19 7.899392E-03 1.000000E+19
359 traj.phases.propelled_ascent.indep_states.states:r_107 c -1.000000E+19 7.969789E-03 1.000000E+19
360 traj.phases.propelled_ascent.indep_states.states:r_108 c -1.000000E+19 8.132373E-03 1.000000E+19
361 traj.phases.propelled_ascent.indep_states.states:r_109 c -1.000000E+19 8.359890E-03 1.000000E+19
362 traj.phases.propelled_ascent.indep_states.states:r_110 c -1.000000E+19 8.432675E-03 1.000000E+19
363 traj.phases.propelled_ascent.indep_states.states:r_111 c -1.000000E+19 8.600761E-03 1.000000E+19
364 traj.phases.propelled_ascent.indep_states.states:r_112 c -1.000000E+19 8.835954E-03 1.000000E+19
365 traj.phases.propelled_ascent.indep_states.states:r_113 c -1.000000E+19 8.911188E-03 1.000000E+19
366 traj.phases.propelled_ascent.indep_states.states:r_114 c -1.000000E+19 9.084922E-03 1.000000E+19
367 traj.phases.propelled_ascent.indep_states.states:r_115 c -1.000000E+19 9.327997E-03 1.000000E+19
368 traj.phases.propelled_ascent.indep_states.states:r_116 c -1.000000E+19 9.405749E-03 1.000000E+19
369 traj.phases.propelled_ascent.indep_states.states:r_117 c -1.000000E+19 9.585290E-03 1.000000E+19
370 traj.phases.propelled_ascent.indep_states.states:r_118 c -1.000000E+19 9.836476E-03 1.000000E+19
371 traj.phases.propelled_ascent.indep_states.states:r_119 c -1.000000E+19 9.916820E-03 1.000000E+19
372 traj.phases.propelled_ascent.indep_states.states:r_120 c -1.000000E+19 1.010234E-02 1.000000E+19
373 traj.phases.propelled_ascent.indep_states.states:r_121 c -1.000000E+19 1.036189E-02 1.000000E+19
374 traj.phases.propelled_ascent.indep_states.states:r_122 c -1.000000E+19 1.044491E-02 1.000000E+19
375 traj.phases.propelled_ascent.indep_states.states:r_123 c -1.000000E+19 1.063660E-02 1.000000E+19
376 traj.phases.propelled_ascent.indep_states.states:r_124 c -1.000000E+19 1.090479E-02 1.000000E+19
377 traj.phases.propelled_ascent.indep_states.states:r_125 c -1.000000E+19 1.099057E-02 1.000000E+19
378 traj.phases.propelled_ascent.indep_states.states:r_126 c -1.000000E+19 1.118866E-02 1.000000E+19
379 traj.phases.propelled_ascent.indep_states.states:r_127 c -1.000000E+19 1.146579E-02 1.000000E+19
380 traj.phases.propelled_ascent.indep_states.states:r_128 c -1.000000E+19 1.155444E-02 1.000000E+19
381 traj.phases.propelled_ascent.indep_states.states:r_129 c -1.000000E+19 1.175914E-02 1.000000E+19
382 traj.phases.propelled_ascent.indep_states.states:r_130 c -1.000000E+19 1.204556E-02 1.000000E+19
383 traj.phases.propelled_ascent.indep_states.states:r_131 c -1.000000E+19 1.213718E-02 1.000000E+19
384 traj.phases.propelled_ascent.indep_states.states:r_132 c -1.000000E+19 1.234877E-02 1.000000E+19
385 traj.phases.propelled_ascent.indep_states.states:r_133 c -1.000000E+19 1.264486E-02 1.000000E+19
386 traj.phases.propelled_ascent.indep_states.states:r_134 c -1.000000E+19 1.273958E-02 1.000000E+19
387 traj.phases.propelled_ascent.indep_states.states:r_135 c -1.000000E+19 1.295835E-02 1.000000E+19
388 traj.phases.propelled_ascent.indep_states.states:r_136 c -1.000000E+19 1.326453E-02 1.000000E+19
389 traj.phases.propelled_ascent.indep_states.states:r_137 c -1.000000E+19 1.336249E-02 1.000000E+19
390 traj.phases.propelled_ascent.indep_states.states:r_138 c -1.000000E+19 1.358877E-02 1.000000E+19
391 traj.phases.propelled_ascent.indep_states.states:r_139 c -1.000000E+19 1.390552E-02 1.000000E+19
392 traj.phases.propelled_ascent.indep_states.states:r_140 c -1.000000E+19 1.400688E-02 1.000000E+19
393 traj.phases.propelled_ascent.indep_states.states:r_141 c -1.000000E+19 1.424104E-02 1.000000E+19
394 traj.phases.propelled_ascent.indep_states.states:r_142 c -1.000000E+19 1.456890E-02 1.000000E+19
395 traj.phases.propelled_ascent.indep_states.states:r_143 c -1.000000E+19 1.467384E-02 1.000000E+19
396 traj.phases.propelled_ascent.indep_states.states:r_144 c -1.000000E+19 1.491631E-02 1.000000E+19
397 traj.phases.propelled_ascent.indep_states.states:r_145 c -1.000000E+19 1.525590E-02 1.000000E+19
398 traj.phases.propelled_ascent.indep_states.states:r_146 c -1.000000E+19 1.536463E-02 1.000000E+19
399 traj.phases.propelled_ascent.indep_states.states:r_147 c -1.000000E+19 1.561589E-02 1.000000E+19
400 traj.phases.propelled_ascent.indep_states.states:r_148 c -1.000000E+19 1.596794E-02 1.000000E+19
401 traj.phases.propelled_ascent.indep_states.states:r_149 c -1.000000E+19 1.608068E-02 1.000000E+19
402 traj.phases.propelled_ascent.indep_states.states:h_0 c -1.000000E+19 1.315706E-06 1.000000E+19
403 traj.phases.propelled_ascent.indep_states.states:h_1 c -1.000000E+19 5.535970E-06 1.000000E+19
404 traj.phases.propelled_ascent.indep_states.states:h_2 c -1.000000E+19 7.445819E-06 1.000000E+19
405 traj.phases.propelled_ascent.indep_states.states:h_3 c -1.000000E+19 1.282391E-05 1.000000E+19
406 traj.phases.propelled_ascent.indep_states.states:h_4 c -1.000000E+19 2.254242E-05 1.000000E+19
407 traj.phases.propelled_ascent.indep_states.states:h_5 c -1.000000E+19 2.616755E-05 1.000000E+19
408 traj.phases.propelled_ascent.indep_states.states:h_6 c -1.000000E+19 3.545444E-05 1.000000E+19
409 traj.phases.propelled_ascent.indep_states.states:h_7 c -1.000000E+19 5.050262E-05 1.000000E+19
410 traj.phases.propelled_ascent.indep_states.states:h_8 c -1.000000E+19 5.580064E-05 1.000000E+19
411 traj.phases.propelled_ascent.indep_states.states:h_9 c -1.000000E+19 6.889508E-05 1.000000E+19
412 traj.phases.propelled_ascent.indep_states.states:h_10 c -1.000000E+19 8.914732E-05 1.000000E+19
413 traj.phases.propelled_ascent.indep_states.states:h_11 c -1.000000E+19 9.608125E-05 1.000000E+19
414 traj.phases.propelled_ascent.indep_states.states:h_12 c -1.000000E+19 1.129027E-04 1.000000E+19
415 traj.phases.propelled_ascent.indep_states.states:h_13 c -1.000000E+19 1.382565E-04 1.000000E+19
416 traj.phases.propelled_ascent.indep_states.states:h_14 c -1.000000E+19 1.467957E-04 1.000000E+19
417 traj.phases.propelled_ascent.indep_states.states:h_15 c -1.000000E+19 1.672772E-04 1.000000E+19
418 traj.phases.propelled_ascent.indep_states.states:h_16 c -1.000000E+19 1.976463E-04 1.000000E+19
419 traj.phases.propelled_ascent.indep_states.states:h_17 c -1.000000E+19 2.077649E-04 1.000000E+19
420 traj.phases.propelled_ascent.indep_states.states:h_18 c -1.000000E+19 2.318493E-04 1.000000E+19
421 traj.phases.propelled_ascent.indep_states.states:h_19 c -1.000000E+19 2.671601E-04 1.000000E+19
422 traj.phases.propelled_ascent.indep_states.states:h_20 c -1.000000E+19 2.788360E-04 1.000000E+19
423 traj.phases.propelled_ascent.indep_states.states:h_21 c -1.000000E+19 3.064747E-04 1.000000E+19
424 traj.phases.propelled_ascent.indep_states.states:h_22 c -1.000000E+19 3.466643E-04 1.000000E+19
425 traj.phases.propelled_ascent.indep_states.states:h_23 c -1.000000E+19 3.598785E-04 1.000000E+19
426 traj.phases.propelled_ascent.indep_states.states:h_24 c -1.000000E+19 3.910297E-04 1.000000E+19
427 traj.phases.propelled_ascent.indep_states.states:h_25 c -1.000000E+19 4.360443E-04 1.000000E+19
428 traj.phases.propelled_ascent.indep_states.states:h_26 c -1.000000E+19 4.507807E-04 1.000000E+19
429 traj.phases.propelled_ascent.indep_states.states:h_27 c -1.000000E+19 4.854090E-04 1.000000E+19
430 traj.phases.propelled_ascent.indep_states.states:h_28 c -1.000000E+19 5.352028E-04 1.000000E+19
431 traj.phases.propelled_ascent.indep_states.states:h_29 c -1.000000E+19 5.514476E-04 1.000000E+19
432 traj.phases.propelled_ascent.indep_states.states:h_30 c -1.000000E+19 5.895229E-04 1.000000E+19
433 traj.phases.propelled_ascent.indep_states.states:h_31 c -1.000000E+19 6.440577E-04 1.000000E+19
434 traj.phases.propelled_ascent.indep_states.states:h_32 c -1.000000E+19 6.617994E-04 1.000000E+19
435 traj.phases.propelled_ascent.indep_states.states:h_33 c -1.000000E+19 7.032969E-04 1.000000E+19
436 traj.phases.propelled_ascent.indep_states.states:h_34 c -1.000000E+19 7.625408E-04 1.000000E+19
437 traj.phases.propelled_ascent.indep_states.states:h_35 c -1.000000E+19 7.817702E-04 1.000000E+19
438 traj.phases.propelled_ascent.indep_states.states:h_36 c -1.000000E+19 8.266694E-04 1.000000E+19
439 traj.phases.propelled_ascent.indep_states.states:h_37 c -1.000000E+19 8.905972E-04 1.000000E+19
440 traj.phases.propelled_ascent.indep_states.states:h_38 c -1.000000E+19 9.113068E-04 1.000000E+19
441 traj.phases.propelled_ascent.indep_states.states:h_39 c -1.000000E+19 9.595919E-04 1.000000E+19
442 traj.phases.propelled_ascent.indep_states.states:h_40 c -1.000000E+19 1.028184E-03 1.000000E+19
443 traj.phases.propelled_ascent.indep_states.states:h_41 c -1.000000E+19 1.050368E-03 1.000000E+19
444 traj.phases.propelled_ascent.indep_states.states:h_42 c -1.000000E+19 1.102027E-03 1.000000E+19
445 traj.phases.propelled_ascent.indep_states.states:h_43 c -1.000000E+19 1.175270E-03 1.000000E+19
446 traj.phases.propelled_ascent.indep_states.states:h_44 c -1.000000E+19 1.198925E-03 1.000000E+19
447 traj.phases.propelled_ascent.indep_states.states:h_45 c -1.000000E+19 1.253950E-03 1.000000E+19
448 traj.phases.propelled_ascent.indep_states.states:h_46 c -1.000000E+19 1.331834E-03 1.000000E+19
449 traj.phases.propelled_ascent.indep_states.states:h_47 c -1.000000E+19 1.356958E-03 1.000000E+19
450 traj.phases.propelled_ascent.indep_states.states:h_48 c -1.000000E+19 1.415346E-03 1.000000E+19
451 traj.phases.propelled_ascent.indep_states.states:h_49 c -1.000000E+19 1.497869E-03 1.000000E+19
452 traj.phases.propelled_ascent.indep_states.states:h_50 c -1.000000E+19 1.524460E-03 1.000000E+19
453 traj.phases.propelled_ascent.indep_states.states:h_51 c -1.000000E+19 1.586210E-03 1.000000E+19
454 traj.phases.propelled_ascent.indep_states.states:h_52 c -1.000000E+19 1.673373E-03 1.000000E+19
455 traj.phases.propelled_ascent.indep_states.states:h_53 c -1.000000E+19 1.701434E-03 1.000000E+19
456 traj.phases.propelled_ascent.indep_states.states:h_54 c -1.000000E+19 1.766549E-03 1.000000E+19
457 traj.phases.propelled_ascent.indep_states.states:h_55 c -1.000000E+19 1.858359E-03 1.000000E+19
458 traj.phases.propelled_ascent.indep_states.states:h_56 c -1.000000E+19 1.887891E-03 1.000000E+19
459 traj.phases.propelled_ascent.indep_states.states:h_57 c -1.000000E+19 1.956378E-03 1.000000E+19
460 traj.phases.propelled_ascent.indep_states.states:h_58 c -1.000000E+19 2.052847E-03 1.000000E+19
461 traj.phases.propelled_ascent.indep_states.states:h_59 c -1.000000E+19 2.083856E-03 1.000000E+19
462 traj.phases.propelled_ascent.indep_states.states:h_60 c -1.000000E+19 2.155726E-03 1.000000E+19
463 traj.phases.propelled_ascent.indep_states.states:h_61 c -1.000000E+19 2.256870E-03 1.000000E+19
464 traj.phases.propelled_ascent.indep_states.states:h_62 c -1.000000E+19 2.289360E-03 1.000000E+19
465 traj.phases.propelled_ascent.indep_states.states:h_63 c -1.000000E+19 2.364628E-03 1.000000E+19
466 traj.phases.propelled_ascent.indep_states.states:h_64 c -1.000000E+19 2.470469E-03 1.000000E+19
467 traj.phases.propelled_ascent.indep_states.states:h_65 c -1.000000E+19 2.504448E-03 1.000000E+19
468 traj.phases.propelled_ascent.indep_states.states:h_66 c -1.000000E+19 2.583131E-03 1.000000E+19
469 traj.phases.propelled_ascent.indep_states.states:h_67 c -1.000000E+19 2.693696E-03 1.000000E+19
470 traj.phases.propelled_ascent.indep_states.states:h_68 c -1.000000E+19 2.729173E-03 1.000000E+19
471 traj.phases.propelled_ascent.indep_states.states:h_69 c -1.000000E+19 2.811292E-03 1.000000E+19
472 traj.phases.propelled_ascent.indep_states.states:h_70 c -1.000000E+19 2.926613E-03 1.000000E+19
473 traj.phases.propelled_ascent.indep_states.states:h_71 c -1.000000E+19 2.963600E-03 1.000000E+19
474 traj.phases.propelled_ascent.indep_states.states:h_72 c -1.000000E+19 3.049181E-03 1.000000E+19
475 traj.phases.propelled_ascent.indep_states.states:h_73 c -1.000000E+19 3.169295E-03 1.000000E+19
476 traj.phases.propelled_ascent.indep_states.states:h_74 c -1.000000E+19 3.207803E-03 1.000000E+19
477 traj.phases.propelled_ascent.indep_states.states:h_75 c -1.000000E+19 3.296875E-03 1.000000E+19
478 traj.phases.propelled_ascent.indep_states.states:h_76 c -1.000000E+19 3.421825E-03 1.000000E+19
479 traj.phases.propelled_ascent.indep_states.states:h_77 c -1.000000E+19 3.461868E-03 1.000000E+19
480 traj.phases.propelled_ascent.indep_states.states:h_78 c -1.000000E+19 3.554465E-03 1.000000E+19
481 traj.phases.propelled_ascent.indep_states.states:h_79 c -1.000000E+19 3.684300E-03 1.000000E+19
482 traj.phases.propelled_ascent.indep_states.states:h_80 c -1.000000E+19 3.725894E-03 1.000000E+19
483 traj.phases.propelled_ascent.indep_states.states:h_81 c -1.000000E+19 3.822054E-03 1.000000E+19
484 traj.phases.propelled_ascent.indep_states.states:h_82 c -1.000000E+19 3.956828E-03 1.000000E+19
485 traj.phases.propelled_ascent.indep_states.states:h_83 c -1.000000E+19 3.999992E-03 1.000000E+19
486 traj.phases.propelled_ascent.indep_states.states:h_84 c -1.000000E+19 4.099756E-03 1.000000E+19
487 traj.phases.propelled_ascent.indep_states.states:h_85 c -1.000000E+19 4.239530E-03 1.000000E+19
488 traj.phases.propelled_ascent.indep_states.states:h_86 c -1.000000E+19 4.284283E-03 1.000000E+19
489 traj.phases.propelled_ascent.indep_states.states:h_87 c -1.000000E+19 4.387699E-03 1.000000E+19
490 traj.phases.propelled_ascent.indep_states.states:h_88 c -1.000000E+19 4.532541E-03 1.000000E+19
491 traj.phases.propelled_ascent.indep_states.states:h_89 c -1.000000E+19 4.578906E-03 1.000000E+19
492 traj.phases.propelled_ascent.indep_states.states:h_90 c -1.000000E+19 4.686025E-03 1.000000E+19
493 traj.phases.propelled_ascent.indep_states.states:h_91 c -1.000000E+19 4.836010E-03 1.000000E+19
494 traj.phases.propelled_ascent.indep_states.states:h_92 c -1.000000E+19 4.884010E-03 1.000000E+19
495 traj.phases.propelled_ascent.indep_states.states:h_93 c -1.000000E+19 4.994891E-03 1.000000E+19
496 traj.phases.propelled_ascent.indep_states.states:h_94 c -1.000000E+19 5.150102E-03 1.000000E+19
497 traj.phases.propelled_ascent.indep_states.states:h_95 c -1.000000E+19 5.199764E-03 1.000000E+19
498 traj.phases.propelled_ascent.indep_states.states:h_96 c -1.000000E+19 5.314469E-03 1.000000E+19
499 traj.phases.propelled_ascent.indep_states.states:h_97 c -1.000000E+19 5.474996E-03 1.000000E+19
500 traj.phases.propelled_ascent.indep_states.states:h_98 c -1.000000E+19 5.526351E-03 1.000000E+19
501 traj.phases.propelled_ascent.indep_states.states:h_99 c -1.000000E+19 5.644949E-03 1.000000E+19
502 traj.phases.propelled_ascent.indep_states.states:h_100 c -1.000000E+19 5.810892E-03 1.000000E+19
503 traj.phases.propelled_ascent.indep_states.states:h_101 c -1.000000E+19 5.863972E-03 1.000000E+19
504 traj.phases.propelled_ascent.indep_states.states:h_102 c -1.000000E+19 5.986541E-03 1.000000E+19
505 traj.phases.propelled_ascent.indep_states.states:h_103 c -1.000000E+19 6.158009E-03 1.000000E+19
506 traj.phases.propelled_ascent.indep_states.states:h_104 c -1.000000E+19 6.212849E-03 1.000000E+19
507 traj.phases.propelled_ascent.indep_states.states:h_105 c -1.000000E+19 6.339471E-03 1.000000E+19
508 traj.phases.propelled_ascent.indep_states.states:h_106 c -1.000000E+19 6.516585E-03 1.000000E+19
509 traj.phases.propelled_ascent.indep_states.states:h_107 c -1.000000E+19 6.573225E-03 1.000000E+19
510 traj.phases.propelled_ascent.indep_states.states:h_108 c -1.000000E+19 6.703992E-03 1.000000E+19
511 traj.phases.propelled_ascent.indep_states.states:h_109 c -1.000000E+19 6.886883E-03 1.000000E+19
512 traj.phases.propelled_ascent.indep_states.states:h_110 c -1.000000E+19 6.945366E-03 1.000000E+19
513 traj.phases.propelled_ascent.indep_states.states:h_111 c -1.000000E+19 7.080379E-03 1.000000E+19
514 traj.phases.propelled_ascent.indep_states.states:h_112 c -1.000000E+19 7.269191E-03 1.000000E+19
515 traj.phases.propelled_ascent.indep_states.states:h_113 c -1.000000E+19 7.329564E-03 1.000000E+19
516 traj.phases.propelled_ascent.indep_states.states:h_114 c -1.000000E+19 7.468934E-03 1.000000E+19
517 traj.phases.propelled_ascent.indep_states.states:h_115 c -1.000000E+19 7.663827E-03 1.000000E+19
518 traj.phases.propelled_ascent.indep_states.states:h_116 c -1.000000E+19 7.726142E-03 1.000000E+19
519 traj.phases.propelled_ascent.indep_states.states:h_117 c -1.000000E+19 7.869990E-03 1.000000E+19
520 traj.phases.propelled_ascent.indep_states.states:h_118 c -1.000000E+19 8.071139E-03 1.000000E+19
521 traj.phases.propelled_ascent.indep_states.states:h_119 c -1.000000E+19 8.135452E-03 1.000000E+19
522 traj.phases.propelled_ascent.indep_states.states:h_120 c -1.000000E+19 8.283914E-03 1.000000E+19
523 traj.phases.propelled_ascent.indep_states.states:h_121 c -1.000000E+19 8.491511E-03 1.000000E+19
524 traj.phases.propelled_ascent.indep_states.states:h_122 c -1.000000E+19 8.557887E-03 1.000000E+19
525 traj.phases.propelled_ascent.indep_states.states:h_123 c -1.000000E+19 8.711110E-03 1.000000E+19
526 traj.phases.propelled_ascent.indep_states.states:h_124 c -1.000000E+19 8.925369E-03 1.000000E+19
527 traj.phases.propelled_ascent.indep_states.states:h_125 c -1.000000E+19 8.993876E-03 1.000000E+19
528 traj.phases.propelled_ascent.indep_states.states:h_126 c -1.000000E+19 9.152024E-03 1.000000E+19
529 traj.phases.propelled_ascent.indep_states.states:h_127 c -1.000000E+19 9.373183E-03 1.000000E+19
530 traj.phases.propelled_ascent.indep_states.states:h_128 c -1.000000E+19 9.443900E-03 1.000000E+19
531 traj.phases.propelled_ascent.indep_states.states:h_129 c -1.000000E+19 9.607156E-03 1.000000E+19
532 traj.phases.propelled_ascent.indep_states.states:h_130 c -1.000000E+19 9.835477E-03 1.000000E+19
533 traj.phases.propelled_ascent.indep_states.states:h_131 c -1.000000E+19 9.908490E-03 1.000000E+19
534 traj.phases.propelled_ascent.indep_states.states:h_132 c -1.000000E+19 1.007706E-02 1.000000E+19
535 traj.phases.propelled_ascent.indep_states.states:h_133 c -1.000000E+19 1.031284E-02 1.000000E+19
536 traj.phases.propelled_ascent.indep_states.states:h_134 c -1.000000E+19 1.038824E-02 1.000000E+19
537 traj.phases.propelled_ascent.indep_states.states:h_135 c -1.000000E+19 1.056235E-02 1.000000E+19
538 traj.phases.propelled_ascent.indep_states.states:h_136 c -1.000000E+19 1.080591E-02 1.000000E+19
539 traj.phases.propelled_ascent.indep_states.states:h_137 c -1.000000E+19 1.088382E-02 1.000000E+19
540 traj.phases.propelled_ascent.indep_states.states:h_138 c -1.000000E+19 1.106372E-02 1.000000E+19
541 traj.phases.propelled_ascent.indep_states.states:h_139 c -1.000000E+19 1.131545E-02 1.000000E+19
542 traj.phases.propelled_ascent.indep_states.states:h_140 c -1.000000E+19 1.139598E-02 1.000000E+19
543 traj.phases.propelled_ascent.indep_states.states:h_141 c -1.000000E+19 1.158197E-02 1.000000E+19
544 traj.phases.propelled_ascent.indep_states.states:h_142 c -1.000000E+19 1.184228E-02 1.000000E+19
545 traj.phases.propelled_ascent.indep_states.states:h_143 c -1.000000E+19 1.192558E-02 1.000000E+19
546 traj.phases.propelled_ascent.indep_states.states:h_144 c -1.000000E+19 1.211799E-02 1.000000E+19
547 traj.phases.propelled_ascent.indep_states.states:h_145 c -1.000000E+19 1.238737E-02 1.000000E+19
548 traj.phases.propelled_ascent.indep_states.states:h_146 c -1.000000E+19 1.247359E-02 1.000000E+19
549 traj.phases.propelled_ascent.indep_states.states:h_147 c -1.000000E+19 1.267280E-02 1.000000E+19
550 traj.phases.propelled_ascent.indep_states.states:h_148 c -1.000000E+19 1.295181E-02 1.000000E+19
551 traj.phases.propelled_ascent.indep_states.states:h_149 c -1.000000E+19 1.304114E-02 1.000000E+19
552 traj.phases.propelled_ascent.indep_states.states:gam_0 c 0.000000E+00 5.121320E-01 9.444444E-01
553 traj.phases.propelled_ascent.indep_states.states:gam_1 c 0.000000E+00 4.914616E-01 9.444444E-01
554 traj.phases.propelled_ascent.indep_states.states:gam_2 c 0.000000E+00 4.853061E-01 9.444444E-01
555 traj.phases.propelled_ascent.indep_states.states:gam_3 c 0.000000E+00 4.820600E-01 9.444444E-01
556 traj.phases.propelled_ascent.indep_states.states:gam_4 c 0.000000E+00 4.783200E-01 9.444444E-01
557 traj.phases.propelled_ascent.indep_states.states:gam_5 c 0.000000E+00 4.744231E-01 9.444444E-01
558 traj.phases.propelled_ascent.indep_states.states:gam_6 c 0.000000E+00 4.733635E-01 9.444444E-01
559 traj.phases.propelled_ascent.indep_states.states:gam_7 c 0.000000E+00 4.712170E-01 9.444444E-01
560 traj.phases.propelled_ascent.indep_states.states:gam_8 c 0.000000E+00 4.687007E-01 9.444444E-01
561 traj.phases.propelled_ascent.indep_states.states:gam_9 c 0.000000E+00 4.679839E-01 9.444444E-01
562 traj.phases.propelled_ascent.indep_states.states:gam_10 c 0.000000E+00 4.664661E-01 9.444444E-01
563 traj.phases.propelled_ascent.indep_states.states:gam_11 c 0.000000E+00 4.645995E-01 9.444444E-01
564 traj.phases.propelled_ascent.indep_states.states:gam_12 c 0.000000E+00 4.640535E-01 9.444444E-01
565 traj.phases.propelled_ascent.indep_states.states:gam_13 c 0.000000E+00 4.628741E-01 9.444444E-01
566 traj.phases.propelled_ascent.indep_states.states:gam_14 c 0.000000E+00 4.613853E-01 9.444444E-01
567 traj.phases.propelled_ascent.indep_states.states:gam_15 c 0.000000E+00 4.609426E-01 9.444444E-01
568 traj.phases.propelled_ascent.indep_states.states:gam_16 c 0.000000E+00 4.599753E-01 9.444444E-01
569 traj.phases.propelled_ascent.indep_states.states:gam_17 c 0.000000E+00 4.587339E-01 9.444444E-01
570 traj.phases.propelled_ascent.indep_states.states:gam_18 c 0.000000E+00 4.583608E-01 9.444444E-01
571 traj.phases.propelled_ascent.indep_states.states:gam_19 c 0.000000E+00 4.575392E-01 9.444444E-01
572 traj.phases.propelled_ascent.indep_states.states:gam_20 c 0.000000E+00 4.564726E-01 9.444444E-01
573 traj.phases.propelled_ascent.indep_states.states:gam_21 c 0.000000E+00 4.561497E-01 9.444444E-01
574 traj.phases.propelled_ascent.indep_states.states:gam_22 c 0.000000E+00 4.554344E-01 9.444444E-01
575 traj.phases.propelled_ascent.indep_states.states:gam_23 c 0.000000E+00 4.544982E-01 9.444444E-01
576 traj.phases.propelled_ascent.indep_states.states:gam_24 c 0.000000E+00 4.542131E-01 9.444444E-01
577 traj.phases.propelled_ascent.indep_states.states:gam_25 c 0.000000E+00 4.535790E-01 9.444444E-01
578 traj.phases.propelled_ascent.indep_states.states:gam_26 c 0.000000E+00 4.527439E-01 9.444444E-01
579 traj.phases.propelled_ascent.indep_states.states:gam_27 c 0.000000E+00 4.524885E-01 9.444444E-01
580 traj.phases.propelled_ascent.indep_states.states:gam_28 c 0.000000E+00 4.519186E-01 9.444444E-01
581 traj.phases.propelled_ascent.indep_states.states:gam_29 c 0.000000E+00 4.511642E-01 9.444444E-01
582 traj.phases.propelled_ascent.indep_states.states:gam_30 c 0.000000E+00 4.509327E-01 9.444444E-01
583 traj.phases.propelled_ascent.indep_states.states:gam_31 c 0.000000E+00 4.504148E-01 9.444444E-01
584 traj.phases.propelled_ascent.indep_states.states:gam_32 c 0.000000E+00 4.497265E-01 9.444444E-01
585 traj.phases.propelled_ascent.indep_states.states:gam_33 c 0.000000E+00 4.495146E-01 9.444444E-01
586 traj.phases.propelled_ascent.indep_states.states:gam_34 c 0.000000E+00 4.490398E-01 9.444444E-01
587 traj.phases.propelled_ascent.indep_states.states:gam_35 c 0.000000E+00 4.484067E-01 9.444444E-01
588 traj.phases.propelled_ascent.indep_states.states:gam_36 c 0.000000E+00 4.482113E-01 9.444444E-01
589 traj.phases.propelled_ascent.indep_states.states:gam_37 c 0.000000E+00 4.477727E-01 9.444444E-01
590 traj.phases.propelled_ascent.indep_states.states:gam_38 c 0.000000E+00 4.471864E-01 9.444444E-01
591 traj.phases.propelled_ascent.indep_states.states:gam_39 c 0.000000E+00 4.470051E-01 9.444444E-01
592 traj.phases.propelled_ascent.indep_states.states:gam_40 c 0.000000E+00 4.465976E-01 9.444444E-01
593 traj.phases.propelled_ascent.indep_states.states:gam_41 c 0.000000E+00 4.460514E-01 9.444444E-01
594 traj.phases.propelled_ascent.indep_states.states:gam_42 c 0.000000E+00 4.458823E-01 9.444444E-01
595 traj.phases.propelled_ascent.indep_states.states:gam_43 c 0.000000E+00 4.455016E-01 9.444444E-01
596 traj.phases.propelled_ascent.indep_states.states:gam_44 c 0.000000E+00 4.449904E-01 9.444444E-01
597 traj.phases.propelled_ascent.indep_states.states:gam_45 c 0.000000E+00 4.448320E-01 9.444444E-01
598 traj.phases.propelled_ascent.indep_states.states:gam_46 c 0.000000E+00 4.444747E-01 9.444444E-01
599 traj.phases.propelled_ascent.indep_states.states:gam_47 c 0.000000E+00 4.439943E-01 9.444444E-01
600 traj.phases.propelled_ascent.indep_states.states:gam_48 c 0.000000E+00 4.438452E-01 9.444444E-01
601 traj.phases.propelled_ascent.indep_states.states:gam_49 c 0.000000E+00 4.435087E-01 9.444444E-01
602 traj.phases.propelled_ascent.indep_states.states:gam_50 c 0.000000E+00 4.430555E-01 9.444444E-01
603 traj.phases.propelled_ascent.indep_states.states:gam_51 c 0.000000E+00 4.429147E-01 9.444444E-01
604 traj.phases.propelled_ascent.indep_states.states:gam_52 c 0.000000E+00 4.425967E-01 9.444444E-01
605 traj.phases.propelled_ascent.indep_states.states:gam_53 c 0.000000E+00 4.421679E-01 9.444444E-01
606 traj.phases.propelled_ascent.indep_states.states:gam_54 c 0.000000E+00 4.420345E-01 9.444444E-01
607 traj.phases.propelled_ascent.indep_states.states:gam_55 c 0.000000E+00 4.417330E-01 9.444444E-01
608 traj.phases.propelled_ascent.indep_states.states:gam_56 c 0.000000E+00 4.413261E-01 9.444444E-01
609 traj.phases.propelled_ascent.indep_states.states:gam_57 c 0.000000E+00 4.411994E-01 9.444444E-01
610 traj.phases.propelled_ascent.indep_states.states:gam_58 c 0.000000E+00 4.409130E-01 9.444444E-01
611 traj.phases.propelled_ascent.indep_states.states:gam_59 c 0.000000E+00 4.405260E-01 9.444444E-01
612 traj.phases.propelled_ascent.indep_states.states:gam_60 c 0.000000E+00 4.404053E-01 9.444444E-01
613 traj.phases.propelled_ascent.indep_states.states:gam_61 c 0.000000E+00 4.401325E-01 9.444444E-01
614 traj.phases.propelled_ascent.indep_states.states:gam_62 c 0.000000E+00 4.397635E-01 9.444444E-01
615 traj.phases.propelled_ascent.indep_states.states:gam_63 c 0.000000E+00 4.396485E-01 9.444444E-01
616 traj.phases.propelled_ascent.indep_states.states:gam_64 c 0.000000E+00 4.393881E-01 9.444444E-01
617 traj.phases.propelled_ascent.indep_states.states:gam_65 c 0.000000E+00 4.390356E-01 9.444444E-01
618 traj.phases.propelled_ascent.indep_states.states:gam_66 c 0.000000E+00 4.389257E-01 9.444444E-01
619 traj.phases.propelled_ascent.indep_states.states:gam_67 c 0.000000E+00 4.386767E-01 9.444444E-01
620 traj.phases.propelled_ascent.indep_states.states:gam_68 c 0.000000E+00 4.383394E-01 9.444444E-01
621 traj.phases.propelled_ascent.indep_states.states:gam_69 c 0.000000E+00 4.382341E-01 9.444444E-01
622 traj.phases.propelled_ascent.indep_states.states:gam_70 c 0.000000E+00 4.379957E-01 9.444444E-01
623 traj.phases.propelled_ascent.indep_states.states:gam_71 c 0.000000E+00 4.376725E-01 9.444444E-01
624 traj.phases.propelled_ascent.indep_states.states:gam_72 c 0.000000E+00 4.375715E-01 9.444444E-01
625 traj.phases.propelled_ascent.indep_states.states:gam_73 c 0.000000E+00 4.373428E-01 9.444444E-01
626 traj.phases.propelled_ascent.indep_states.states:gam_74 c 0.000000E+00 4.370326E-01 9.444444E-01
627 traj.phases.propelled_ascent.indep_states.states:gam_75 c 0.000000E+00 4.369357E-01 9.444444E-01
628 traj.phases.propelled_ascent.indep_states.states:gam_76 c 0.000000E+00 4.367160E-01 9.444444E-01
629 traj.phases.propelled_ascent.indep_states.states:gam_77 c 0.000000E+00 4.364180E-01 9.444444E-01
630 traj.phases.propelled_ascent.indep_states.states:gam_78 c 0.000000E+00 4.363248E-01 9.444444E-01
631 traj.phases.propelled_ascent.indep_states.states:gam_79 c 0.000000E+00 4.361136E-01 9.444444E-01
632 traj.phases.propelled_ascent.indep_states.states:gam_80 c 0.000000E+00 4.358268E-01 9.444444E-01
633 traj.phases.propelled_ascent.indep_states.states:gam_81 c 0.000000E+00 4.357372E-01 9.444444E-01
634 traj.phases.propelled_ascent.indep_states.states:gam_82 c 0.000000E+00 4.355339E-01 9.444444E-01
635 traj.phases.propelled_ascent.indep_states.states:gam_83 c 0.000000E+00 4.352577E-01 9.444444E-01
636 traj.phases.propelled_ascent.indep_states.states:gam_84 c 0.000000E+00 4.351714E-01 9.444444E-01
637 traj.phases.propelled_ascent.indep_states.states:gam_85 c 0.000000E+00 4.349755E-01 9.444444E-01
638 traj.phases.propelled_ascent.indep_states.states:gam_86 c 0.000000E+00 4.347093E-01 9.444444E-01
639 traj.phases.propelled_ascent.indep_states.states:gam_87 c 0.000000E+00 4.346260E-01 9.444444E-01
640 traj.phases.propelled_ascent.indep_states.states:gam_88 c 0.000000E+00 4.344371E-01 9.444444E-01
641 traj.phases.propelled_ascent.indep_states.states:gam_89 c 0.000000E+00 4.341804E-01 9.444444E-01
642 traj.phases.propelled_ascent.indep_states.states:gam_90 c 0.000000E+00 4.341000E-01 9.444444E-01
643 traj.phases.propelled_ascent.indep_states.states:gam_91 c 0.000000E+00 4.339177E-01 9.444444E-01
644 traj.phases.propelled_ascent.indep_states.states:gam_92 c 0.000000E+00 4.336698E-01 9.444444E-01
645 traj.phases.propelled_ascent.indep_states.states:gam_93 c 0.000000E+00 4.335923E-01 9.444444E-01
646 traj.phases.propelled_ascent.indep_states.states:gam_94 c 0.000000E+00 4.334162E-01 9.444444E-01
647 traj.phases.propelled_ascent.indep_states.states:gam_95 c 0.000000E+00 4.331767E-01 9.444444E-01
648 traj.phases.propelled_ascent.indep_states.states:gam_96 c 0.000000E+00 4.331018E-01 9.444444E-01
649 traj.phases.propelled_ascent.indep_states.states:gam_97 c 0.000000E+00 4.329316E-01 9.444444E-01
650 traj.phases.propelled_ascent.indep_states.states:gam_98 c 0.000000E+00 4.327002E-01 9.444444E-01
651 traj.phases.propelled_ascent.indep_states.states:gam_99 c 0.000000E+00 4.326277E-01 9.444444E-01
652 traj.phases.propelled_ascent.indep_states.states:gam_100 c 0.000000E+00 4.324632E-01 9.444444E-01
653 traj.phases.propelled_ascent.indep_states.states:gam_101 c 0.000000E+00 4.322394E-01 9.444444E-01
654 traj.phases.propelled_ascent.indep_states.states:gam_102 c 0.000000E+00 4.321693E-01 9.444444E-01
655 traj.phases.propelled_ascent.indep_states.states:gam_103 c 0.000000E+00 4.320102E-01 9.444444E-01
656 traj.phases.propelled_ascent.indep_states.states:gam_104 c 0.000000E+00 4.317936E-01 9.444444E-01
657 traj.phases.propelled_ascent.indep_states.states:gam_105 c 0.000000E+00 4.317258E-01 9.444444E-01
658 traj.phases.propelled_ascent.indep_states.states:gam_106 c 0.000000E+00 4.315718E-01 9.444444E-01
659 traj.phases.propelled_ascent.indep_states.states:gam_107 c 0.000000E+00 4.313622E-01 9.444444E-01
660 traj.phases.propelled_ascent.indep_states.states:gam_108 c 0.000000E+00 4.312966E-01 9.444444E-01
661 traj.phases.propelled_ascent.indep_states.states:gam_109 c 0.000000E+00 4.311475E-01 9.444444E-01
662 traj.phases.propelled_ascent.indep_states.states:gam_110 c 0.000000E+00 4.309446E-01 9.444444E-01
663 traj.phases.propelled_ascent.indep_states.states:gam_111 c 0.000000E+00 4.308811E-01 9.444444E-01
664 traj.phases.propelled_ascent.indep_states.states:gam_112 c 0.000000E+00 4.307367E-01 9.444444E-01
665 traj.phases.propelled_ascent.indep_states.states:gam_113 c 0.000000E+00 4.305402E-01 9.444444E-01
666 traj.phases.propelled_ascent.indep_states.states:gam_114 c 0.000000E+00 4.304787E-01 9.444444E-01
667 traj.phases.propelled_ascent.indep_states.states:gam_115 c 0.000000E+00 4.303389E-01 9.444444E-01
668 traj.phases.propelled_ascent.indep_states.states:gam_116 c 0.000000E+00 4.301486E-01 9.444444E-01
669 traj.phases.propelled_ascent.indep_states.states:gam_117 c 0.000000E+00 4.300890E-01 9.444444E-01
670 traj.phases.propelled_ascent.indep_states.states:gam_118 c 0.000000E+00 4.299536E-01 9.444444E-01
671 traj.phases.propelled_ascent.indep_states.states:gam_119 c 0.000000E+00 4.297693E-01 9.444444E-01
672 traj.phases.propelled_ascent.indep_states.states:gam_120 c 0.000000E+00 4.297115E-01 9.444444E-01
673 traj.phases.propelled_ascent.indep_states.states:gam_121 c 0.000000E+00 4.295804E-01 9.444444E-01
674 traj.phases.propelled_ascent.indep_states.states:gam_122 c 0.000000E+00 4.294018E-01 9.444444E-01
675 traj.phases.propelled_ascent.indep_states.states:gam_123 c 0.000000E+00 4.293459E-01 9.444444E-01
676 traj.phases.propelled_ascent.indep_states.states:gam_124 c 0.000000E+00 4.292188E-01 9.444444E-01
677 traj.phases.propelled_ascent.indep_states.states:gam_125 c 0.000000E+00 4.290459E-01 9.444444E-01
678 traj.phases.propelled_ascent.indep_states.states:gam_126 c 0.000000E+00 4.289917E-01 9.444444E-01
679 traj.phases.propelled_ascent.indep_states.states:gam_127 c 0.000000E+00 4.288686E-01 9.444444E-01
680 traj.phases.propelled_ascent.indep_states.states:gam_128 c 0.000000E+00 4.287011E-01 9.444444E-01
681 traj.phases.propelled_ascent.indep_states.states:gam_129 c 0.000000E+00 4.286486E-01 9.444444E-01
682 traj.phases.propelled_ascent.indep_states.states:gam_130 c 0.000000E+00 4.285294E-01 9.444444E-01
683 traj.phases.propelled_ascent.indep_states.states:gam_131 c 0.000000E+00 4.283672E-01 9.444444E-01
684 traj.phases.propelled_ascent.indep_states.states:gam_132 c 0.000000E+00 4.283164E-01 9.444444E-01
685 traj.phases.propelled_ascent.indep_states.states:gam_133 c 0.000000E+00 4.282010E-01 9.444444E-01
686 traj.phases.propelled_ascent.indep_states.states:gam_134 c 0.000000E+00 4.280439E-01 9.444444E-01
687 traj.phases.propelled_ascent.indep_states.states:gam_135 c 0.000000E+00 4.279947E-01 9.444444E-01
688 traj.phases.propelled_ascent.indep_states.states:gam_136 c 0.000000E+00 4.278830E-01 9.444444E-01
689 traj.phases.propelled_ascent.indep_states.states:gam_137 c 0.000000E+00 4.277310E-01 9.444444E-01
690 traj.phases.propelled_ascent.indep_states.states:gam_138 c 0.000000E+00 4.276834E-01 9.444444E-01
691 traj.phases.propelled_ascent.indep_states.states:gam_139 c 0.000000E+00 4.275754E-01 9.444444E-01
692 traj.phases.propelled_ascent.indep_states.states:gam_140 c 0.000000E+00 4.274283E-01 9.444444E-01
693 traj.phases.propelled_ascent.indep_states.states:gam_141 c 0.000000E+00 4.273823E-01 9.444444E-01
694 traj.phases.propelled_ascent.indep_states.states:gam_142 c 0.000000E+00 4.272777E-01 9.444444E-01
695 traj.phases.propelled_ascent.indep_states.states:gam_143 c 0.000000E+00 4.271356E-01 9.444444E-01
696 traj.phases.propelled_ascent.indep_states.states:gam_144 c 0.000000E+00 4.270911E-01 9.444444E-01
697 traj.phases.propelled_ascent.indep_states.states:gam_145 c 0.000000E+00 4.269901E-01 9.444444E-01
698 traj.phases.propelled_ascent.indep_states.states:gam_146 c 0.000000E+00 4.268527E-01 9.444444E-01
699 traj.phases.propelled_ascent.indep_states.states:gam_147 c 0.000000E+00 4.268097E-01 9.444444E-01
700 traj.phases.propelled_ascent.indep_states.states:gam_148 c 0.000000E+00 4.267122E-01 9.444444E-01
701 traj.phases.propelled_ascent.indep_states.states:gam_149 c 0.000000E+00 4.265796E-01 9.444444E-01
702 traj.phases.propelled_ascent.indep_states.states:gam_150 c 0.000000E+00 4.265381E-01 9.444444E-01
703 traj.phases.propelled_ascent.indep_states.states:v_0 c 1.000000E-04 3.679528E-03 1.000000E+19
704 traj.phases.propelled_ascent.indep_states.states:v_1 c 1.000000E-04 7.350891E-03 1.000000E+19
705 traj.phases.propelled_ascent.indep_states.states:v_2 c 1.000000E-04 8.506452E-03 1.000000E+19
706 traj.phases.propelled_ascent.indep_states.states:v_3 c 1.000000E-04 1.114203E-02 1.000000E+19
707 traj.phases.propelled_ascent.indep_states.states:v_4 c 1.000000E-04 1.475465E-02 1.000000E+19
708 traj.phases.propelled_ascent.indep_states.states:v_5 c 1.000000E-04 1.589246E-02 1.000000E+19
709 traj.phases.propelled_ascent.indep_states.states:v_6 c 1.000000E-04 1.848823E-02 1.000000E+19
710 traj.phases.propelled_ascent.indep_states.states:v_7 c 1.000000E-04 2.204857E-02 1.000000E+19
711 traj.phases.propelled_ascent.indep_states.states:v_8 c 1.000000E-04 2.317046E-02 1.000000E+19
712 traj.phases.propelled_ascent.indep_states.states:v_9 c 1.000000E-04 2.573091E-02 1.000000E+19
713 traj.phases.propelled_ascent.indep_states.states:v_10 c 1.000000E-04 2.924498E-02 1.000000E+19
714 traj.phases.propelled_ascent.indep_states.states:v_11 c 1.000000E-04 3.035281E-02 1.000000E+19
715 traj.phases.propelled_ascent.indep_states.states:v_12 c 1.000000E-04 3.288210E-02 1.000000E+19
716 traj.phases.propelled_ascent.indep_states.states:v_13 c 1.000000E-04 3.635546E-02 1.000000E+19
717 traj.phases.propelled_ascent.indep_states.states:v_14 c 1.000000E-04 3.745096E-02 1.000000E+19
718 traj.phases.propelled_ascent.indep_states.states:v_15 c 1.000000E-04 3.995293E-02 1.000000E+19
719 traj.phases.propelled_ascent.indep_states.states:v_16 c 1.000000E-04 4.339074E-02 1.000000E+19
720 traj.phases.propelled_ascent.indep_states.states:v_17 c 1.000000E-04 4.447548E-02 1.000000E+19
721 traj.phases.propelled_ascent.indep_states.states:v_18 c 1.000000E-04 4.695370E-02 1.000000E+19
722 traj.phases.propelled_ascent.indep_states.states:v_19 c 1.000000E-04 5.036072E-02 1.000000E+19
723 traj.phases.propelled_ascent.indep_states.states:v_20 c 1.000000E-04 5.143617E-02 1.000000E+19
724 traj.phases.propelled_ascent.indep_states.states:v_21 c 1.000000E-04 5.389397E-02 1.000000E+19
725 traj.phases.propelled_ascent.indep_states.states:v_22 c 1.000000E-04 5.727464E-02 1.000000E+19
726 traj.phases.propelled_ascent.indep_states.states:v_23 c 1.000000E-04 5.834219E-02 1.000000E+19
727 traj.phases.propelled_ascent.indep_states.states:v_24 c 1.000000E-04 6.078266E-02 1.000000E+19
728 traj.phases.propelled_ascent.indep_states.states:v_25 c 1.000000E-04 6.414115E-02 1.000000E+19
729 traj.phases.propelled_ascent.indep_states.states:v_26 c 1.000000E-04 6.520210E-02 1.000000E+19
730 traj.phases.propelled_ascent.indep_states.states:v_27 c 1.000000E-04 6.762817E-02 1.000000E+19
731 traj.phases.propelled_ascent.indep_states.states:v_28 c 1.000000E-04 7.096843E-02 1.000000E+19
732 traj.phases.propelled_ascent.indep_states.states:v_29 c 1.000000E-04 7.202400E-02 1.000000E+19
733 traj.phases.propelled_ascent.indep_states.states:v_30 c 1.000000E-04 7.443844E-02 1.000000E+19
734 traj.phases.propelled_ascent.indep_states.states:v_31 c 1.000000E-04 7.776421E-02 1.000000E+19
735 traj.phases.propelled_ascent.indep_states.states:v_32 c 1.000000E-04 7.881557E-02 1.000000E+19
736 traj.phases.propelled_ascent.indep_states.states:v_33 c 1.000000E-04 8.122103E-02 1.000000E+19
737 traj.phases.propelled_ascent.indep_states.states:v_34 c 1.000000E-04 8.453591E-02 1.000000E+19
738 traj.phases.propelled_ascent.indep_states.states:v_35 c 1.000000E-04 8.558418E-02 1.000000E+19
739 traj.phases.propelled_ascent.indep_states.states:v_36 c 1.000000E-04 8.798320E-02 1.000000E+19
740 traj.phases.propelled_ascent.indep_states.states:v_37 c 1.000000E-04 9.129065E-02 1.000000E+19
741 traj.phases.propelled_ascent.indep_states.states:v_38 c 1.000000E-04 9.233690E-02 1.000000E+19
742 traj.phases.propelled_ascent.indep_states.states:v_39 c 1.000000E-04 9.473195E-02 1.000000E+19
743 traj.phases.propelled_ascent.indep_states.states:v_40 c 1.000000E-04 9.803532E-02 1.000000E+19
744 traj.phases.propelled_ascent.indep_states.states:v_41 c 1.000000E-04 9.908062E-02 1.000000E+19
745 traj.phases.propelled_ascent.indep_states.states:v_42 c 1.000000E-04 1.014741E-01 1.000000E+19
746 traj.phases.propelled_ascent.indep_states.states:v_43 c 1.000000E-04 1.047767E-01 1.000000E+19
747 traj.phases.propelled_ascent.indep_states.states:v_44 c 1.000000E-04 1.058220E-01 1.000000E+19
748 traj.phases.propelled_ascent.indep_states.states:v_45 c 1.000000E-04 1.082163E-01 1.000000E+19
749 traj.phases.propelled_ascent.indep_states.states:v_46 c 1.000000E-04 1.115213E-01 1.000000E+19
750 traj.phases.propelled_ascent.indep_states.states:v_47 c 1.000000E-04 1.125678E-01 1.000000E+19
751 traj.phases.propelled_ascent.indep_states.states:v_48 c 1.000000E-04 1.149651E-01 1.000000E+19
752 traj.phases.propelled_ascent.indep_states.states:v_49 c 1.000000E-04 1.182757E-01 1.000000E+19
753 traj.phases.propelled_ascent.indep_states.states:v_50 c 1.000000E-04 1.193243E-01 1.000000E+19
754 traj.phases.propelled_ascent.indep_states.states:v_51 c 1.000000E-04 1.217270E-01 1.000000E+19
755 traj.phases.propelled_ascent.indep_states.states:v_52 c 1.000000E-04 1.250465E-01 1.000000E+19
756 traj.phases.propelled_ascent.indep_states.states:v_53 c 1.000000E-04 1.260981E-01 1.000000E+19
757 traj.phases.propelled_ascent.indep_states.states:v_54 c 1.000000E-04 1.285086E-01 1.000000E+19
758 traj.phases.propelled_ascent.indep_states.states:v_55 c 1.000000E-04 1.318400E-01 1.000000E+19
759 traj.phases.propelled_ascent.indep_states.states:v_56 c 1.000000E-04 1.328958E-01 1.000000E+19
760 traj.phases.propelled_ascent.indep_states.states:v_57 c 1.000000E-04 1.353163E-01 1.000000E+19
761 traj.phases.propelled_ascent.indep_states.states:v_58 c 1.000000E-04 1.386629E-01 1.000000E+19
762 traj.phases.propelled_ascent.indep_states.states:v_59 c 1.000000E-04 1.397239E-01 1.000000E+19
763 traj.phases.propelled_ascent.indep_states.states:v_60 c 1.000000E-04 1.421567E-01 1.000000E+19
764 traj.phases.propelled_ascent.indep_states.states:v_61 c 1.000000E-04 1.455218E-01 1.000000E+19
765 traj.phases.propelled_ascent.indep_states.states:v_62 c 1.000000E-04 1.465889E-01 1.000000E+19
766 traj.phases.propelled_ascent.indep_states.states:v_63 c 1.000000E-04 1.490366E-01 1.000000E+19
767 traj.phases.propelled_ascent.indep_states.states:v_64 c 1.000000E-04 1.524235E-01 1.000000E+19
768 traj.phases.propelled_ascent.indep_states.states:v_65 c 1.000000E-04 1.534979E-01 1.000000E+19
769 traj.phases.propelled_ascent.indep_states.states:v_66 c 1.000000E-04 1.559628E-01 1.000000E+19
770 traj.phases.propelled_ascent.indep_states.states:v_67 c 1.000000E-04 1.593749E-01 1.000000E+19
771 traj.phases.propelled_ascent.indep_states.states:v_68 c 1.000000E-04 1.604577E-01 1.000000E+19
772 traj.phases.propelled_ascent.indep_states.states:v_69 c 1.000000E-04 1.629423E-01 1.000000E+19
773 traj.phases.propelled_ascent.indep_states.states:v_70 c 1.000000E-04 1.663833E-01 1.000000E+19
774 traj.phases.propelled_ascent.indep_states.states:v_71 c 1.000000E-04 1.674755E-01 1.000000E+19
775 traj.phases.propelled_ascent.indep_states.states:v_72 c 1.000000E-04 1.699825E-01 1.000000E+19
776 traj.phases.propelled_ascent.indep_states.states:v_73 c 1.000000E-04 1.734560E-01 1.000000E+19
777 traj.phases.propelled_ascent.indep_states.states:v_74 c 1.000000E-04 1.745589E-01 1.000000E+19
778 traj.phases.propelled_ascent.indep_states.states:v_75 c 1.000000E-04 1.770911E-01 1.000000E+19
779 traj.phases.propelled_ascent.indep_states.states:v_76 c 1.000000E-04 1.806008E-01 1.000000E+19
780 traj.phases.propelled_ascent.indep_states.states:v_77 c 1.000000E-04 1.817156E-01 1.000000E+19
781 traj.phases.propelled_ascent.indep_states.states:v_78 c 1.000000E-04 1.842758E-01 1.000000E+19
782 traj.phases.propelled_ascent.indep_states.states:v_79 c 1.000000E-04 1.878260E-01 1.000000E+19
783 traj.phases.propelled_ascent.indep_states.states:v_80 c 1.000000E-04 1.889540E-01 1.000000E+19
784 traj.phases.propelled_ascent.indep_states.states:v_81 c 1.000000E-04 1.915452E-01 1.000000E+19
785 traj.phases.propelled_ascent.indep_states.states:v_82 c 1.000000E-04 1.951400E-01 1.000000E+19
786 traj.phases.propelled_ascent.indep_states.states:v_83 c 1.000000E-04 1.962826E-01 1.000000E+19
787 traj.phases.propelled_ascent.indep_states.states:v_84 c 1.000000E-04 1.989081E-01 1.000000E+19
788 traj.phases.propelled_ascent.indep_states.states:v_85 c 1.000000E-04 2.025521E-01 1.000000E+19
789 traj.phases.propelled_ascent.indep_states.states:v_86 c 1.000000E-04 2.037108E-01 1.000000E+19
790 traj.phases.propelled_ascent.indep_states.states:v_87 c 1.000000E-04 2.063739E-01 1.000000E+19
791 traj.phases.propelled_ascent.indep_states.states:v_88 c 1.000000E-04 2.100720E-01 1.000000E+19
792 traj.phases.propelled_ascent.indep_states.states:v_89 c 1.000000E-04 2.112482E-01 1.000000E+19
793 traj.phases.propelled_ascent.indep_states.states:v_90 c 1.000000E-04 2.139527E-01 1.000000E+19
794 traj.phases.propelled_ascent.indep_states.states:v_91 c 1.000000E-04 2.177099E-01 1.000000E+19
795 traj.phases.propelled_ascent.indep_states.states:v_92 c 1.000000E-04 2.189055E-01 1.000000E+19
796 traj.phases.propelled_ascent.indep_states.states:v_93 c 1.000000E-04 2.216552E-01 1.000000E+19
797 traj.phases.propelled_ascent.indep_states.states:v_94 c 1.000000E-04 2.254773E-01 1.000000E+19
798 traj.phases.propelled_ascent.indep_states.states:v_95 c 1.000000E-04 2.266940E-01 1.000000E+19
799 traj.phases.propelled_ascent.indep_states.states:v_96 c 1.000000E-04 2.294931E-01 1.000000E+19
800 traj.phases.propelled_ascent.indep_states.states:v_97 c 1.000000E-04 2.333861E-01 1.000000E+19
801 traj.phases.propelled_ascent.indep_states.states:v_98 c 1.000000E-04 2.346258E-01 1.000000E+19
802 traj.phases.propelled_ascent.indep_states.states:v_99 c 1.000000E-04 2.374791E-01 1.000000E+19
803 traj.phases.propelled_ascent.indep_states.states:v_100 c 1.000000E-04 2.414495E-01 1.000000E+19
804 traj.phases.propelled_ascent.indep_states.states:v_101 c 1.000000E-04 2.427145E-01 1.000000E+19
805 traj.phases.propelled_ascent.indep_states.states:v_102 c 1.000000E-04 2.456268E-01 1.000000E+19
806 traj.phases.propelled_ascent.indep_states.states:v_103 c 1.000000E-04 2.496819E-01 1.000000E+19
807 traj.phases.propelled_ascent.indep_states.states:v_104 c 1.000000E-04 2.509744E-01 1.000000E+19
808 traj.phases.propelled_ascent.indep_states.states:v_105 c 1.000000E-04 2.539513E-01 1.000000E+19
809 traj.phases.propelled_ascent.indep_states.states:v_106 c 1.000000E-04 2.580990E-01 1.000000E+19
810 traj.phases.propelled_ascent.indep_states.states:v_107 c 1.000000E-04 2.594217E-01 1.000000E+19
811 traj.phases.propelled_ascent.indep_states.states:v_108 c 1.000000E-04 2.624693E-01 1.000000E+19
812 traj.phases.propelled_ascent.indep_states.states:v_109 c 1.000000E-04 2.667182E-01 1.000000E+19
813 traj.phases.propelled_ascent.indep_states.states:v_110 c 1.000000E-04 2.680739E-01 1.000000E+19
814 traj.phases.propelled_ascent.indep_states.states:v_111 c 1.000000E-04 2.711989E-01 1.000000E+19
815 traj.phases.propelled_ascent.indep_states.states:v_112 c 1.000000E-04 2.755588E-01 1.000000E+19
816 traj.phases.propelled_ascent.indep_states.states:v_113 c 1.000000E-04 2.769507E-01 1.000000E+19
817 traj.phases.propelled_ascent.indep_states.states:v_114 c 1.000000E-04 2.801605E-01 1.000000E+19
818 traj.phases.propelled_ascent.indep_states.states:v_115 c 1.000000E-04 2.846423E-01 1.000000E+19
819 traj.phases.propelled_ascent.indep_states.states:v_116 c 1.000000E-04 2.860740E-01 1.000000E+19
820 traj.phases.propelled_ascent.indep_states.states:v_117 c 1.000000E-04 2.893770E-01 1.000000E+19
821 traj.phases.propelled_ascent.indep_states.states:v_118 c 1.000000E-04 2.939927E-01 1.000000E+19
822 traj.phases.propelled_ascent.indep_states.states:v_119 c 1.000000E-04 2.954681E-01 1.000000E+19
823 traj.phases.propelled_ascent.indep_states.states:v_120 c 1.000000E-04 2.988738E-01 1.000000E+19
824 traj.phases.propelled_ascent.indep_states.states:v_121 c 1.000000E-04 3.036372E-01 1.000000E+19
825 traj.phases.propelled_ascent.indep_states.states:v_122 c 1.000000E-04 3.051608E-01 1.000000E+19
826 traj.phases.propelled_ascent.indep_states.states:v_123 c 1.000000E-04 3.086799E-01 1.000000E+19
827 traj.phases.propelled_ascent.indep_states.states:v_124 c 1.000000E-04 3.136066E-01 1.000000E+19
828 traj.phases.propelled_ascent.indep_states.states:v_125 c 1.000000E-04 3.151836E-01 1.000000E+19
829 traj.phases.propelled_ascent.indep_states.states:v_126 c 1.000000E-04 3.188283E-01 1.000000E+19
830 traj.phases.propelled_ascent.indep_states.states:v_127 c 1.000000E-04 3.239361E-01 1.000000E+19
831 traj.phases.propelled_ascent.indep_states.states:v_128 c 1.000000E-04 3.255725E-01 1.000000E+19
832 traj.phases.propelled_ascent.indep_states.states:v_129 c 1.000000E-04 3.293567E-01 1.000000E+19
833 traj.phases.propelled_ascent.indep_states.states:v_130 c 1.000000E-04 3.346663E-01 1.000000E+19
834 traj.phases.propelled_ascent.indep_states.states:v_131 c 1.000000E-04 3.363688E-01 1.000000E+19
835 traj.phases.propelled_ascent.indep_states.states:v_132 c 1.000000E-04 3.403089E-01 1.000000E+19
836 traj.phases.propelled_ascent.indep_states.states:v_133 c 1.000000E-04 3.458441E-01 1.000000E+19
837 traj.phases.propelled_ascent.indep_states.states:v_134 c 1.000000E-04 3.476207E-01 1.000000E+19
838 traj.phases.propelled_ascent.indep_states.states:v_135 c 1.000000E-04 3.517356E-01 1.000000E+19
839 traj.phases.propelled_ascent.indep_states.states:v_136 c 1.000000E-04 3.575245E-01 1.000000E+19
840 traj.phases.propelled_ascent.indep_states.states:v_137 c 1.000000E-04 3.593846E-01 1.000000E+19
841 traj.phases.propelled_ascent.indep_states.states:v_138 c 1.000000E-04 3.636968E-01 1.000000E+19
842 traj.phases.propelled_ascent.indep_states.states:v_139 c 1.000000E-04 3.697727E-01 1.000000E+19
843 traj.phases.propelled_ascent.indep_states.states:v_140 c 1.000000E-04 3.717274E-01 1.000000E+19
844 traj.phases.propelled_ascent.indep_states.states:v_141 c 1.000000E-04 3.762635E-01 1.000000E+19
845 traj.phases.propelled_ascent.indep_states.states:v_142 c 1.000000E-04 3.826661E-01 1.000000E+19
846 traj.phases.propelled_ascent.indep_states.states:v_143 c 1.000000E-04 3.847288E-01 1.000000E+19
847 traj.phases.propelled_ascent.indep_states.states:v_144 c 1.000000E-04 3.895210E-01 1.000000E+19
848 traj.phases.propelled_ascent.indep_states.states:v_145 c 1.000000E-04 3.962985E-01 1.000000E+19
849 traj.phases.propelled_ascent.indep_states.states:v_146 c 1.000000E-04 3.984855E-01 1.000000E+19
850 traj.phases.propelled_ascent.indep_states.states:v_147 c 1.000000E-04 4.035730E-01 1.000000E+19
851 traj.phases.propelled_ascent.indep_states.states:v_148 c 1.000000E-04 4.107848E-01 1.000000E+19
852 traj.phases.propelled_ascent.indep_states.states:v_149 c 1.000000E-04 4.131161E-01 1.000000E+19
853 traj.phases.propelled_ascent.indep_states.states:p_0 c 1.020000E+00 6.420517E+00 1.000000E+21
854 traj.phases.propelled_ascent.indep_states.states:p_1 c 1.020000E+00 6.314633E+00 1.000000E+21
855 traj.phases.propelled_ascent.indep_states.states:p_2 c 1.020000E+00 6.281996E+00 1.000000E+21
856 traj.phases.propelled_ascent.indep_states.states:p_3 c 1.020000E+00 6.208781E+00 1.000000E+21
857 traj.phases.propelled_ascent.indep_states.states:p_4 c 1.020000E+00 6.111091E+00 1.000000E+21
858 traj.phases.propelled_ascent.indep_states.states:p_5 c 1.020000E+00 6.080944E+00 1.000000E+21
859 traj.phases.propelled_ascent.indep_states.states:p_6 c 1.020000E+00 6.013254E+00 1.000000E+21
860 traj.phases.propelled_ascent.indep_states.states:p_7 c 1.020000E+00 5.922805E+00 1.000000E+21
861 traj.phases.propelled_ascent.indep_states.states:p_8 c 1.020000E+00 5.894863E+00 1.000000E+21
862 traj.phases.propelled_ascent.indep_states.states:p_9 c 1.020000E+00 5.832071E+00 1.000000E+21
863 traj.phases.propelled_ascent.indep_states.states:p_10 c 1.020000E+00 5.748056E+00 1.000000E+21
864 traj.phases.propelled_ascent.indep_states.states:p_11 c 1.020000E+00 5.722075E+00 1.000000E+21
865 traj.phases.propelled_ascent.indep_states.states:p_12 c 1.020000E+00 5.663647E+00 1.000000E+21
866 traj.phases.propelled_ascent.indep_states.states:p_13 c 1.020000E+00 5.585376E+00 1.000000E+21
867 traj.phases.propelled_ascent.indep_states.states:p_14 c 1.020000E+00 5.561149E+00 1.000000E+21
868 traj.phases.propelled_ascent.indep_states.states:p_15 c 1.020000E+00 5.506628E+00 1.000000E+21
869 traj.phases.propelled_ascent.indep_states.states:p_16 c 1.020000E+00 5.433507E+00 1.000000E+21
870 traj.phases.propelled_ascent.indep_states.states:p_17 c 1.020000E+00 5.410856E+00 1.000000E+21
871 traj.phases.propelled_ascent.indep_states.states:p_18 c 1.020000E+00 5.359847E+00 1.000000E+21
872 traj.phases.propelled_ascent.indep_states.states:p_19 c 1.020000E+00 5.291364E+00 1.000000E+21
873 traj.phases.propelled_ascent.indep_states.states:p_20 c 1.020000E+00 5.270134E+00 1.000000E+21
874 traj.phases.propelled_ascent.indep_states.states:p_21 c 1.020000E+00 5.222294E+00 1.000000E+21
875 traj.phases.propelled_ascent.indep_states.states:p_22 c 1.020000E+00 5.158005E+00 1.000000E+21
876 traj.phases.propelled_ascent.indep_states.states:p_23 c 1.020000E+00 5.138060E+00 1.000000E+21
877 traj.phases.propelled_ascent.indep_states.states:p_24 c 1.020000E+00 5.093092E+00 1.000000E+21
878 traj.phases.propelled_ascent.indep_states.states:p_25 c 1.020000E+00 5.032608E+00 1.000000E+21
879 traj.phases.propelled_ascent.indep_states.states:p_26 c 1.020000E+00 5.013830E+00 1.000000E+21
880 traj.phases.propelled_ascent.indep_states.states:p_27 c 1.020000E+00 4.971473E+00 1.000000E+21
881 traj.phases.propelled_ascent.indep_states.states:p_28 c 1.020000E+00 4.914451E+00 1.000000E+21
882 traj.phases.propelled_ascent.indep_states.states:p_29 c 1.020000E+00 4.896737E+00 1.000000E+21
883 traj.phases.propelled_ascent.indep_states.states:p_30 c 1.020000E+00 4.856760E+00 1.000000E+21
884 traj.phases.propelled_ascent.indep_states.states:p_31 c 1.020000E+00 4.802900E+00 1.000000E+21
885 traj.phases.propelled_ascent.indep_states.states:p_32 c 1.020000E+00 4.786159E+00 1.000000E+21
886 traj.phases.propelled_ascent.indep_states.states:p_33 c 1.020000E+00 4.748359E+00 1.000000E+21
887 traj.phases.propelled_ascent.indep_states.states:p_34 c 1.020000E+00 4.697395E+00 1.000000E+21
888 traj.phases.propelled_ascent.indep_states.states:p_35 c 1.020000E+00 4.681545E+00 1.000000E+21
889 traj.phases.propelled_ascent.indep_states.states:p_36 c 1.020000E+00 4.645743E+00 1.000000E+21
890 traj.phases.propelled_ascent.indep_states.states:p_37 c 1.020000E+00 4.597438E+00 1.000000E+21
891 traj.phases.propelled_ascent.indep_states.states:p_38 c 1.020000E+00 4.582406E+00 1.000000E+21
892 traj.phases.propelled_ascent.indep_states.states:p_39 c 1.020000E+00 4.548440E+00 1.000000E+21
893 traj.phases.propelled_ascent.indep_states.states:p_40 c 1.020000E+00 4.502583E+00 1.000000E+21
894 traj.phases.propelled_ascent.indep_states.states:p_41 c 1.020000E+00 4.488307E+00 1.000000E+21
895 traj.phases.propelled_ascent.indep_states.states:p_42 c 1.020000E+00 4.456034E+00 1.000000E+21
896 traj.phases.propelled_ascent.indep_states.states:p_43 c 1.020000E+00 4.412436E+00 1.000000E+21
897 traj.phases.propelled_ascent.indep_states.states:p_44 c 1.020000E+00 4.398857E+00 1.000000E+21
898 traj.phases.propelled_ascent.indep_states.states:p_45 c 1.020000E+00 4.368149E+00 1.000000E+21
899 traj.phases.propelled_ascent.indep_states.states:p_46 c 1.020000E+00 4.326641E+00 1.000000E+21
900 traj.phases.propelled_ascent.indep_states.states:p_47 c 1.020000E+00 4.313706E+00 1.000000E+21
901 traj.phases.propelled_ascent.indep_states.states:p_48 c 1.020000E+00 4.284447E+00 1.000000E+21
902 traj.phases.propelled_ascent.indep_states.states:p_49 c 1.020000E+00 4.244876E+00 1.000000E+21
903 traj.phases.propelled_ascent.indep_states.states:p_50 c 1.020000E+00 4.232540E+00 1.000000E+21
904 traj.phases.propelled_ascent.indep_states.states:p_51 c 1.020000E+00 4.204626E+00 1.000000E+21
905 traj.phases.propelled_ascent.indep_states.states:p_52 c 1.020000E+00 4.166853E+00 1.000000E+21
906 traj.phases.propelled_ascent.indep_states.states:p_53 c 1.020000E+00 4.155074E+00 1.000000E+21
907 traj.phases.propelled_ascent.indep_states.states:p_54 c 1.020000E+00 4.128410E+00 1.000000E+21
908 traj.phases.propelled_ascent.indep_states.states:p_55 c 1.020000E+00 4.092312E+00 1.000000E+21
909 traj.phases.propelled_ascent.indep_states.states:p_56 c 1.020000E+00 4.081050E+00 1.000000E+21
910 traj.phases.propelled_ascent.indep_states.states:p_57 c 1.020000E+00 4.055552E+00 1.000000E+21
911 traj.phases.propelled_ascent.indep_states.states:p_58 c 1.020000E+00 4.021015E+00 1.000000E+21
912 traj.phases.propelled_ascent.indep_states.states:p_59 c 1.020000E+00 4.010236E+00 1.000000E+21
913 traj.phases.propelled_ascent.indep_states.states:p_60 c 1.020000E+00 3.985825E+00 1.000000E+21
914 traj.phases.propelled_ascent.indep_states.states:p_61 c 1.020000E+00 3.952745E+00 1.000000E+21
915 traj.phases.propelled_ascent.indep_states.states:p_62 c 1.020000E+00 3.942419E+00 1.000000E+21
916 traj.phases.propelled_ascent.indep_states.states:p_63 c 1.020000E+00 3.919024E+00 1.000000E+21
917 traj.phases.propelled_ascent.indep_states.states:p_64 c 1.020000E+00 3.887308E+00 1.000000E+21
918 traj.phases.propelled_ascent.indep_states.states:p_65 c 1.020000E+00 3.877404E+00 1.000000E+21
919 traj.phases.propelled_ascent.indep_states.states:p_66 c 1.020000E+00 3.854961E+00 1.000000E+21
920 traj.phases.propelled_ascent.indep_states.states:p_67 c 1.020000E+00 3.824523E+00 1.000000E+21
921 traj.phases.propelled_ascent.indep_states.states:p_68 c 1.020000E+00 3.815015E+00 1.000000E+21
922 traj.phases.propelled_ascent.indep_states.states:p_69 c 1.020000E+00 3.793465E+00 1.000000E+21
923 traj.phases.propelled_ascent.indep_states.states:p_70 c 1.020000E+00 3.764227E+00 1.000000E+21
924 traj.phases.propelled_ascent.indep_states.states:p_71 c 1.020000E+00 3.755090E+00 1.000000E+21
925 traj.phases.propelled_ascent.indep_states.states:p_72 c 1.020000E+00 3.734378E+00 1.000000E+21
926 traj.phases.propelled_ascent.indep_states.states:p_73 c 1.020000E+00 3.706267E+00 1.000000E+21
927 traj.phases.propelled_ascent.indep_states.states:p_74 c 1.020000E+00 3.697481E+00 1.000000E+21
928 traj.phases.propelled_ascent.indep_states.states:p_75 c 1.020000E+00 3.677557E+00 1.000000E+21
929 traj.phases.propelled_ascent.indep_states.states:p_76 c 1.020000E+00 3.650506E+00 1.000000E+21
930 traj.phases.propelled_ascent.indep_states.states:p_77 c 1.020000E+00 3.642049E+00 1.000000E+21
931 traj.phases.propelled_ascent.indep_states.states:p_78 c 1.020000E+00 3.622868E+00 1.000000E+21
932 traj.phases.propelled_ascent.indep_states.states:p_79 c 1.020000E+00 3.596817E+00 1.000000E+21
933 traj.phases.propelled_ascent.indep_states.states:p_80 c 1.020000E+00 3.588670E+00 1.000000E+21
934 traj.phases.propelled_ascent.indep_states.states:p_81 c 1.020000E+00 3.570189E+00 1.000000E+21
935 traj.phases.propelled_ascent.indep_states.states:p_82 c 1.020000E+00 3.545080E+00 1.000000E+21
936 traj.phases.propelled_ascent.indep_states.states:p_83 c 1.020000E+00 3.537226E+00 1.000000E+21
937 traj.phases.propelled_ascent.indep_states.states:p_84 c 1.020000E+00 3.519406E+00 1.000000E+21
938 traj.phases.propelled_ascent.indep_states.states:p_85 c 1.020000E+00 3.495188E+00 1.000000E+21
939 traj.phases.propelled_ascent.indep_states.states:p_86 c 1.020000E+00 3.487611E+00 1.000000E+21
940 traj.phases.propelled_ascent.indep_states.states:p_87 c 1.020000E+00 3.470416E+00 1.000000E+21
941 traj.phases.propelled_ascent.indep_states.states:p_88 c 1.020000E+00 3.447041E+00 1.000000E+21
942 traj.phases.propelled_ascent.indep_states.states:p_89 c 1.020000E+00 3.439725E+00 1.000000E+21
943 traj.phases.propelled_ascent.indep_states.states:p_90 c 1.020000E+00 3.423122E+00 1.000000E+21
944 traj.phases.propelled_ascent.indep_states.states:p_91 c 1.020000E+00 3.400543E+00 1.000000E+21
945 traj.phases.propelled_ascent.indep_states.states:p_92 c 1.020000E+00 3.393476E+00 1.000000E+21
946 traj.phases.propelled_ascent.indep_states.states:p_93 c 1.020000E+00 3.377433E+00 1.000000E+21
947 traj.phases.propelled_ascent.indep_states.states:p_94 c 1.020000E+00 3.355610E+00 1.000000E+21
948 traj.phases.propelled_ascent.indep_states.states:p_95 c 1.020000E+00 3.348778E+00 1.000000E+21
949 traj.phases.propelled_ascent.indep_states.states:p_96 c 1.020000E+00 3.333266E+00 1.000000E+21
950 traj.phases.propelled_ascent.indep_states.states:p_97 c 1.020000E+00 3.312160E+00 1.000000E+21
951 traj.phases.propelled_ascent.indep_states.states:p_98 c 1.020000E+00 3.305551E+00 1.000000E+21
952 traj.phases.propelled_ascent.indep_states.states:p_99 c 1.020000E+00 3.290543E+00 1.000000E+21
953 traj.phases.propelled_ascent.indep_states.states:p_100 c 1.020000E+00 3.270119E+00 1.000000E+21
954 traj.phases.propelled_ascent.indep_states.states:p_101 c 1.020000E+00 3.263722E+00 1.000000E+21
955 traj.phases.propelled_ascent.indep_states.states:p_102 c 1.020000E+00 3.249193E+00 1.000000E+21
956 traj.phases.propelled_ascent.indep_states.states:p_103 c 1.020000E+00 3.229415E+00 1.000000E+21
957 traj.phases.propelled_ascent.indep_states.states:p_104 c 1.020000E+00 3.223220E+00 1.000000E+21
958 traj.phases.propelled_ascent.indep_states.states:p_105 c 1.020000E+00 3.209147E+00 1.000000E+21
959 traj.phases.propelled_ascent.indep_states.states:p_106 c 1.020000E+00 3.189985E+00 1.000000E+21
960 traj.phases.propelled_ascent.indep_states.states:p_107 c 1.020000E+00 3.183982E+00 1.000000E+21
961 traj.phases.propelled_ascent.indep_states.states:p_108 c 1.020000E+00 3.170343E+00 1.000000E+21
962 traj.phases.propelled_ascent.indep_states.states:p_109 c 1.020000E+00 3.151768E+00 1.000000E+21
963 traj.phases.propelled_ascent.indep_states.states:p_110 c 1.020000E+00 3.145947E+00 1.000000E+21
964 traj.phases.propelled_ascent.indep_states.states:p_111 c 1.020000E+00 3.132721E+00 1.000000E+21
965 traj.phases.propelled_ascent.indep_states.states:p_112 c 1.020000E+00 3.114705E+00 1.000000E+21
966 traj.phases.propelled_ascent.indep_states.states:p_113 c 1.020000E+00 3.109058E+00 1.000000E+21
967 traj.phases.propelled_ascent.indep_states.states:p_114 c 1.020000E+00 3.096227E+00 1.000000E+21
968 traj.phases.propelled_ascent.indep_states.states:p_115 c 1.020000E+00 3.078744E+00 1.000000E+21
969 traj.phases.propelled_ascent.indep_states.states:p_116 c 1.020000E+00 3.073263E+00 1.000000E+21
970 traj.phases.propelled_ascent.indep_states.states:p_117 c 1.020000E+00 3.060808E+00 1.000000E+21
971 traj.phases.propelled_ascent.indep_states.states:p_118 c 1.020000E+00 3.043834E+00 1.000000E+21
972 traj.phases.propelled_ascent.indep_states.states:p_119 c 1.020000E+00 3.038512E+00 1.000000E+21
973 traj.phases.propelled_ascent.indep_states.states:p_120 c 1.020000E+00 3.026416E+00 1.000000E+21
974 traj.phases.propelled_ascent.indep_states.states:p_121 c 1.020000E+00 3.009929E+00 1.000000E+21
975 traj.phases.propelled_ascent.indep_states.states:p_122 c 1.020000E+00 3.004759E+00 1.000000E+21
976 traj.phases.propelled_ascent.indep_states.states:p_123 c 1.020000E+00 2.993006E+00 1.000000E+21
977 traj.phases.propelled_ascent.indep_states.states:p_124 c 1.020000E+00 2.976983E+00 1.000000E+21
978 traj.phases.propelled_ascent.indep_states.states:p_125 c 1.020000E+00 2.971958E+00 1.000000E+21
979 traj.phases.propelled_ascent.indep_states.states:p_126 c 1.020000E+00 2.960534E+00 1.000000E+21
980 traj.phases.propelled_ascent.indep_states.states:p_127 c 1.020000E+00 2.944956E+00 1.000000E+21
981 traj.phases.propelled_ascent.indep_states.states:p_128 c 1.020000E+00 2.940070E+00 1.000000E+21
982 traj.phases.propelled_ascent.indep_states.states:p_129 c 1.020000E+00 2.928960E+00 1.000000E+21
983 traj.phases.propelled_ascent.indep_states.states:p_130 c 1.020000E+00 2.913808E+00 1.000000E+21
984 traj.phases.propelled_ascent.indep_states.states:p_131 c 1.020000E+00 2.909055E+00 1.000000E+21
985 traj.phases.propelled_ascent.indep_states.states:p_132 c 1.020000E+00 2.898246E+00 1.000000E+21
986 traj.phases.propelled_ascent.indep_states.states:p_133 c 1.020000E+00 2.883503E+00 1.000000E+21
987 traj.phases.propelled_ascent.indep_states.states:p_134 c 1.020000E+00 2.878877E+00 1.000000E+21
988 traj.phases.propelled_ascent.indep_states.states:p_135 c 1.020000E+00 2.868357E+00 1.000000E+21
989 traj.phases.propelled_ascent.indep_states.states:p_136 c 1.020000E+00 2.854004E+00 1.000000E+21
990 traj.phases.propelled_ascent.indep_states.states:p_137 c 1.020000E+00 2.849501E+00 1.000000E+21
991 traj.phases.propelled_ascent.indep_states.states:p_138 c 1.020000E+00 2.839258E+00 1.000000E+21
992 traj.phases.propelled_ascent.indep_states.states:p_139 c 1.020000E+00 2.825280E+00 1.000000E+21
993 traj.phases.propelled_ascent.indep_states.states:p_140 c 1.020000E+00 2.820894E+00 1.000000E+21
994 traj.phases.propelled_ascent.indep_states.states:p_141 c 1.020000E+00 2.810917E+00 1.000000E+21
995 traj.phases.propelled_ascent.indep_states.states:p_142 c 1.020000E+00 2.797300E+00 1.000000E+21
996 traj.phases.propelled_ascent.indep_states.states:p_143 c 1.020000E+00 2.793026E+00 1.000000E+21
997 traj.phases.propelled_ascent.indep_states.states:p_144 c 1.020000E+00 2.783304E+00 1.000000E+21
998 traj.phases.propelled_ascent.indep_states.states:p_145 c 1.020000E+00 2.770033E+00 1.000000E+21
999 traj.phases.propelled_ascent.indep_states.states:p_146 c 1.020000E+00 2.765867E+00 1.000000E+21
1000 traj.phases.propelled_ascent.indep_states.states:p_147 c 1.020000E+00 2.756390E+00 1.000000E+21
1001 traj.phases.propelled_ascent.indep_states.states:p_148 c 1.020000E+00 2.743452E+00 1.000000E+21
1002 traj.phases.propelled_ascent.indep_states.states:p_149 c 1.020000E+00 2.739390E+00 1.000000E+21
1003 traj.phases.propelled_ascent.indep_states.states:V_w_0 c 0.000000E+00 1.026550E-01 1.000000E+20
1004 traj.phases.propelled_ascent.indep_states.states:V_w_1 c 0.000000E+00 1.016518E-01 1.000000E+20
1005 traj.phases.propelled_ascent.indep_states.states:V_w_2 c 0.000000E+00 1.002794E-01 1.000000E+20
1006 traj.phases.propelled_ascent.indep_states.states:V_w_3 c 0.000000E+00 9.984787E-02 1.000000E+20
1007 traj.phases.propelled_ascent.indep_states.states:V_w_4 c 0.000000E+00 9.886465E-02 1.000000E+20
1008 traj.phases.propelled_ascent.indep_states.states:V_w_5 c 0.000000E+00 9.751916E-02 1.000000E+20
1009 traj.phases.propelled_ascent.indep_states.states:V_w_6 c 0.000000E+00 9.709596E-02 1.000000E+20
1010 traj.phases.propelled_ascent.indep_states.states:V_w_7 c 0.000000E+00 9.613156E-02 1.000000E+20
1011 traj.phases.propelled_ascent.indep_states.states:V_w_8 c 0.000000E+00 9.481139E-02 1.000000E+20
1012 traj.phases.propelled_ascent.indep_states.states:V_w_9 c 0.000000E+00 9.439605E-02 1.000000E+20
1013 traj.phases.propelled_ascent.indep_states.states:V_w_10 c 0.000000E+00 9.344940E-02 1.000000E+20
1014 traj.phases.propelled_ascent.indep_states.states:V_w_11 c 0.000000E+00 9.215315E-02 1.000000E+20
1015 traj.phases.propelled_ascent.indep_states.states:V_w_12 c 0.000000E+00 9.174525E-02 1.000000E+20
1016 traj.phases.propelled_ascent.indep_states.states:V_w_13 c 0.000000E+00 9.081539E-02 1.000000E+20
1017 traj.phases.propelled_ascent.indep_states.states:V_w_14 c 0.000000E+00 8.954180E-02 1.000000E+20
1018 traj.phases.propelled_ascent.indep_states.states:V_w_15 c 0.000000E+00 8.914095E-02 1.000000E+20
1019 traj.phases.propelled_ascent.indep_states.states:V_w_16 c 0.000000E+00 8.822703E-02 1.000000E+20
1020 traj.phases.propelled_ascent.indep_states.states:V_w_17 c 0.000000E+00 8.697494E-02 1.000000E+20
1021 traj.phases.propelled_ascent.indep_states.states:V_w_18 c 0.000000E+00 8.658079E-02 1.000000E+20
1022 traj.phases.propelled_ascent.indep_states.states:V_w_19 c 0.000000E+00 8.568200E-02 1.000000E+20
1023 traj.phases.propelled_ascent.indep_states.states:V_w_20 c 0.000000E+00 8.445038E-02 1.000000E+20
1024 traj.phases.propelled_ascent.indep_states.states:V_w_21 c 0.000000E+00 8.406260E-02 1.000000E+20
1025 traj.phases.propelled_ascent.indep_states.states:V_w_22 c 0.000000E+00 8.317823E-02 1.000000E+20
1026 traj.phases.propelled_ascent.indep_states.states:V_w_23 c 0.000000E+00 8.196610E-02 1.000000E+20
1027 traj.phases.propelled_ascent.indep_states.states:V_w_24 c 0.000000E+00 8.158439E-02 1.000000E+20
1028 traj.phases.propelled_ascent.indep_states.states:V_w_25 c 0.000000E+00 8.071378E-02 1.000000E+20
1029 traj.phases.propelled_ascent.indep_states.states:V_w_26 c 0.000000E+00 7.952026E-02 1.000000E+20
1030 traj.phases.propelled_ascent.indep_states.states:V_w_27 c 0.000000E+00 7.914436E-02 1.000000E+20
1031 traj.phases.propelled_ascent.indep_states.states:V_w_28 c 0.000000E+00 7.828688E-02 1.000000E+20
1032 traj.phases.propelled_ascent.indep_states.states:V_w_29 c 0.000000E+00 7.711117E-02 1.000000E+20
1033 traj.phases.propelled_ascent.indep_states.states:V_w_30 c 0.000000E+00 7.674082E-02 1.000000E+20
1034 traj.phases.propelled_ascent.indep_states.states:V_w_31 c 0.000000E+00 7.589592E-02 1.000000E+20
1035 traj.phases.propelled_ascent.indep_states.states:V_w_32 c 0.000000E+00 7.473725E-02 1.000000E+20
1036 traj.phases.propelled_ascent.indep_states.states:V_w_33 c 0.000000E+00 7.437223E-02 1.000000E+20
1037 traj.phases.propelled_ascent.indep_states.states:V_w_34 c 0.000000E+00 7.353939E-02 1.000000E+20
1038 traj.phases.propelled_ascent.indep_states.states:V_w_35 c 0.000000E+00 7.239706E-02 1.000000E+20
1039 traj.phases.propelled_ascent.indep_states.states:V_w_36 c 0.000000E+00 7.203715E-02 1.000000E+20
1040 traj.phases.propelled_ascent.indep_states.states:V_w_37 c 0.000000E+00 7.121588E-02 1.000000E+20
1041 traj.phases.propelled_ascent.indep_states.states:V_w_38 c 0.000000E+00 7.008926E-02 1.000000E+20
1042 traj.phases.propelled_ascent.indep_states.states:V_w_39 c 0.000000E+00 6.973425E-02 1.000000E+20
1043 traj.phases.propelled_ascent.indep_states.states:V_w_40 c 0.000000E+00 6.892411E-02 1.000000E+20
1044 traj.phases.propelled_ascent.indep_states.states:V_w_41 c 0.000000E+00 6.781258E-02 1.000000E+20
1045 traj.phases.propelled_ascent.indep_states.states:V_w_42 c 0.000000E+00 6.746230E-02 1.000000E+20
1046 traj.phases.propelled_ascent.indep_states.states:V_w_43 c 0.000000E+00 6.666286E-02 1.000000E+20
1047 traj.phases.propelled_ascent.indep_states.states:V_w_44 c 0.000000E+00 6.556587E-02 1.000000E+20
1048 traj.phases.propelled_ascent.indep_states.states:V_w_45 c 0.000000E+00 6.522013E-02 1.000000E+20
1049 traj.phases.propelled_ascent.indep_states.states:V_w_46 c 0.000000E+00 6.443100E-02 1.000000E+20
1050 traj.phases.propelled_ascent.indep_states.states:V_w_47 c 0.000000E+00 6.334803E-02 1.000000E+20
1051 traj.phases.propelled_ascent.indep_states.states:V_w_48 c 0.000000E+00 6.300667E-02 1.000000E+20
1052 traj.phases.propelled_ascent.indep_states.states:V_w_49 c 0.000000E+00 6.222749E-02 1.000000E+20
1053 traj.phases.propelled_ascent.indep_states.states:V_w_50 c 0.000000E+00 6.115803E-02 1.000000E+20
1054 traj.phases.propelled_ascent.indep_states.states:V_w_51 c 0.000000E+00 6.082090E-02 1.000000E+20
1055 traj.phases.propelled_ascent.indep_states.states:V_w_52 c 0.000000E+00 6.005133E-02 1.000000E+20
1056 traj.phases.propelled_ascent.indep_states.states:V_w_53 c 0.000000E+00 5.899493E-02 1.000000E+20
1057 traj.phases.propelled_ascent.indep_states.states:V_w_54 c 0.000000E+00 5.866189E-02 1.000000E+20
1058 traj.phases.propelled_ascent.indep_states.states:V_w_55 c 0.000000E+00 5.790159E-02 1.000000E+20
1059 traj.phases.propelled_ascent.indep_states.states:V_w_56 c 0.000000E+00 5.685782E-02 1.000000E+20
1060 traj.phases.propelled_ascent.indep_states.states:V_w_57 c 0.000000E+00 5.652873E-02 1.000000E+20
1061 traj.phases.propelled_ascent.indep_states.states:V_w_58 c 0.000000E+00 5.577741E-02 1.000000E+20
1062 traj.phases.propelled_ascent.indep_states.states:V_w_59 c 0.000000E+00 5.474585E-02 1.000000E+20
1063 traj.phases.propelled_ascent.indep_states.states:V_w_60 c 0.000000E+00 5.442059E-02 1.000000E+20
1064 traj.phases.propelled_ascent.indep_states.states:V_w_61 c 0.000000E+00 5.367796E-02 1.000000E+20
1065 traj.phases.propelled_ascent.indep_states.states:V_w_62 c 0.000000E+00 5.265823E-02 1.000000E+20
1066 traj.phases.propelled_ascent.indep_states.states:V_w_63 c 0.000000E+00 5.233667E-02 1.000000E+20
1067 traj.phases.propelled_ascent.indep_states.states:V_w_64 c 0.000000E+00 5.160247E-02 1.000000E+20
1068 traj.phases.propelled_ascent.indep_states.states:V_w_65 c 0.000000E+00 5.059421E-02 1.000000E+20
1069 traj.phases.propelled_ascent.indep_states.states:V_w_66 c 0.000000E+00 5.027624E-02 1.000000E+20
1070 traj.phases.propelled_ascent.indep_states.states:V_w_67 c 0.000000E+00 4.955020E-02 1.000000E+20
1071 traj.phases.propelled_ascent.indep_states.states:V_w_68 c 0.000000E+00 4.855307E-02 1.000000E+20
1072 traj.phases.propelled_ascent.indep_states.states:V_w_69 c 0.000000E+00 4.823859E-02 1.000000E+20
1073 traj.phases.propelled_ascent.indep_states.states:V_w_70 c 0.000000E+00 4.752047E-02 1.000000E+20
1074 traj.phases.propelled_ascent.indep_states.states:V_w_71 c 0.000000E+00 4.653414E-02 1.000000E+20
1075 traj.phases.propelled_ascent.indep_states.states:V_w_72 c 0.000000E+00 4.622305E-02 1.000000E+20
1076 traj.phases.propelled_ascent.indep_states.states:V_w_73 c 0.000000E+00 4.551263E-02 1.000000E+20
1077 traj.phases.propelled_ascent.indep_states.states:V_w_74 c 0.000000E+00 4.453678E-02 1.000000E+20
1078 traj.phases.propelled_ascent.indep_states.states:V_w_75 c 0.000000E+00 4.422898E-02 1.000000E+20
1079 traj.phases.propelled_ascent.indep_states.states:V_w_76 c 0.000000E+00 4.352604E-02 1.000000E+20
1080 traj.phases.propelled_ascent.indep_states.states:V_w_77 c 0.000000E+00 4.256039E-02 1.000000E+20
1081 traj.phases.propelled_ascent.indep_states.states:V_w_78 c 0.000000E+00 4.225579E-02 1.000000E+20
1082 traj.phases.propelled_ascent.indep_states.states:V_w_79 c 0.000000E+00 4.156012E-02 1.000000E+20
1083 traj.phases.propelled_ascent.indep_states.states:V_w_80 c 0.000000E+00 4.060440E-02 1.000000E+20
1084 traj.phases.propelled_ascent.indep_states.states:V_w_81 c 0.000000E+00 4.030291E-02 1.000000E+20
1085 traj.phases.propelled_ascent.indep_states.states:V_w_82 c 0.000000E+00 3.961431E-02 1.000000E+20
1086 traj.phases.propelled_ascent.indep_states.states:V_w_83 c 0.000000E+00 3.866824E-02 1.000000E+20
1087 traj.phases.propelled_ascent.indep_states.states:V_w_84 c 0.000000E+00 3.836979E-02 1.000000E+20
1088 traj.phases.propelled_ascent.indep_states.states:V_w_85 c 0.000000E+00 3.768808E-02 1.000000E+20
1089 traj.phases.propelled_ascent.indep_states.states:V_w_86 c 0.000000E+00 3.675142E-02 1.000000E+20
1090 traj.phases.propelled_ascent.indep_states.states:V_w_87 c 0.000000E+00 3.645591E-02 1.000000E+20
1091 traj.phases.propelled_ascent.indep_states.states:V_w_88 c 0.000000E+00 3.578092E-02 1.000000E+20
1092 traj.phases.propelled_ascent.indep_states.states:V_w_89 c 0.000000E+00 3.485342E-02 1.000000E+20
1093 traj.phases.propelled_ascent.indep_states.states:V_w_90 c 0.000000E+00 3.456079E-02 1.000000E+20
1094 traj.phases.propelled_ascent.indep_states.states:V_w_91 c 0.000000E+00 3.389235E-02 1.000000E+20
1095 traj.phases.propelled_ascent.indep_states.states:V_w_92 c 0.000000E+00 3.297378E-02 1.000000E+20
1096 traj.phases.propelled_ascent.indep_states.states:V_w_93 c 0.000000E+00 3.268396E-02 1.000000E+20
1097 traj.phases.propelled_ascent.indep_states.states:V_w_94 c 0.000000E+00 3.202190E-02 1.000000E+20
1098 traj.phases.propelled_ascent.indep_states.states:V_w_95 c 0.000000E+00 3.111205E-02 1.000000E+20
1099 traj.phases.propelled_ascent.indep_states.states:V_w_96 c 0.000000E+00 3.082496E-02 1.000000E+20
1100 traj.phases.propelled_ascent.indep_states.states:V_w_97 c 0.000000E+00 3.016913E-02 1.000000E+20
1101 traj.phases.propelled_ascent.indep_states.states:V_w_98 c 0.000000E+00 2.926778E-02 1.000000E+20
1102 traj.phases.propelled_ascent.indep_states.states:V_w_99 c 0.000000E+00 2.898337E-02 1.000000E+20
1103 traj.phases.propelled_ascent.indep_states.states:V_w_100 c 0.000000E+00 2.833362E-02 1.000000E+20
1104 traj.phases.propelled_ascent.indep_states.states:V_w_101 c 0.000000E+00 2.744058E-02 1.000000E+20
1105 traj.phases.propelled_ascent.indep_states.states:V_w_102 c 0.000000E+00 2.715878E-02 1.000000E+20
1106 traj.phases.propelled_ascent.indep_states.states:V_w_103 c 0.000000E+00 2.651497E-02 1.000000E+20
1107 traj.phases.propelled_ascent.indep_states.states:V_w_104 c 0.000000E+00 2.563005E-02 1.000000E+20
1108 traj.phases.propelled_ascent.indep_states.states:V_w_105 c 0.000000E+00 2.535079E-02 1.000000E+20
1109 traj.phases.propelled_ascent.indep_states.states:V_w_106 c 0.000000E+00 2.471279E-02 1.000000E+20
1110 traj.phases.propelled_ascent.indep_states.states:V_w_107 c 0.000000E+00 2.383580E-02 1.000000E+20
1111 traj.phases.propelled_ascent.indep_states.states:V_w_108 c 0.000000E+00 2.355903E-02 1.000000E+20
1112 traj.phases.propelled_ascent.indep_states.states:V_w_109 c 0.000000E+00 2.292670E-02 1.000000E+20
1113 traj.phases.propelled_ascent.indep_states.states:V_w_110 c 0.000000E+00 2.205747E-02 1.000000E+20
1114 traj.phases.propelled_ascent.indep_states.states:V_w_111 c 0.000000E+00 2.178315E-02 1.000000E+20
1115 traj.phases.propelled_ascent.indep_states.states:V_w_112 c 0.000000E+00 2.115637E-02 1.000000E+20
1116 traj.phases.propelled_ascent.indep_states.states:V_w_113 c 0.000000E+00 2.029473E-02 1.000000E+20
1117 traj.phases.propelled_ascent.indep_states.states:V_w_114 c 0.000000E+00 2.002278E-02 1.000000E+20
1118 traj.phases.propelled_ascent.indep_states.states:V_w_115 c 0.000000E+00 1.940144E-02 1.000000E+20
1119 traj.phases.propelled_ascent.indep_states.states:V_w_116 c 0.000000E+00 1.854722E-02 1.000000E+20
1120 traj.phases.propelled_ascent.indep_states.states:V_w_117 c 0.000000E+00 1.827761E-02 1.000000E+20
1121 traj.phases.propelled_ascent.indep_states.states:V_w_118 c 0.000000E+00 1.766158E-02 1.000000E+20
1122 traj.phases.propelled_ascent.indep_states.states:V_w_119 c 0.000000E+00 1.681463E-02 1.000000E+20
1123 traj.phases.propelled_ascent.indep_states.states:V_w_120 c 0.000000E+00 1.654731E-02 1.000000E+20
1124 traj.phases.propelled_ascent.indep_states.states:V_w_121 c 0.000000E+00 1.593648E-02 1.000000E+20
1125 traj.phases.propelled_ascent.indep_states.states:V_w_122 c 0.000000E+00 1.509666E-02 1.000000E+20
1126 traj.phases.propelled_ascent.indep_states.states:V_w_123 c 0.000000E+00 1.483157E-02 1.000000E+20
1127 traj.phases.propelled_ascent.indep_states.states:V_w_124 c 0.000000E+00 1.422585E-02 1.000000E+20
1128 traj.phases.propelled_ascent.indep_states.states:V_w_125 c 0.000000E+00 1.339300E-02 1.000000E+20
1129 traj.phases.propelled_ascent.indep_states.states:V_w_126 c 0.000000E+00 1.313011E-02 1.000000E+20
1130 traj.phases.propelled_ascent.indep_states.states:V_w_127 c 0.000000E+00 1.252938E-02 1.000000E+20
1131 traj.phases.propelled_ascent.indep_states.states:V_w_128 c 0.000000E+00 1.170337E-02 1.000000E+20
1132 traj.phases.propelled_ascent.indep_states.states:V_w_129 c 0.000000E+00 1.144263E-02 1.000000E+20
1133 traj.phases.propelled_ascent.indep_states.states:V_w_130 c 0.000000E+00 1.084680E-02 1.000000E+20
1134 traj.phases.propelled_ascent.indep_states.states:V_w_131 c 0.000000E+00 1.002749E-02 1.000000E+20
1135 traj.phases.propelled_ascent.indep_states.states:V_w_132 c 0.000000E+00 9.768857E-03 1.000000E+20
1136 traj.phases.propelled_ascent.indep_states.states:V_w_133 c 0.000000E+00 9.177831E-03 1.000000E+20
1137 traj.phases.propelled_ascent.indep_states.states:V_w_134 c 0.000000E+00 8.365097E-03 1.000000E+20
1138 traj.phases.propelled_ascent.indep_states.states:V_w_135 c 0.000000E+00 8.108533E-03 1.000000E+20
1139 traj.phases.propelled_ascent.indep_states.states:V_w_136 c 0.000000E+00 7.522220E-03 1.000000E+20
1140 traj.phases.propelled_ascent.indep_states.states:V_w_137 c 0.000000E+00 6.715937E-03 1.000000E+20
1141 traj.phases.propelled_ascent.indep_states.states:V_w_138 c 0.000000E+00 6.461401E-03 1.000000E+20
1142 traj.phases.propelled_ascent.indep_states.states:V_w_139 c 0.000000E+00 5.879712E-03 1.000000E+20
1143 traj.phases.propelled_ascent.indep_states.states:V_w_140 c 0.000000E+00 5.079759E-03 1.000000E+20
1144 traj.phases.propelled_ascent.indep_states.states:V_w_141 c 0.000000E+00 4.827216E-03 1.000000E+20
1145 traj.phases.propelled_ascent.indep_states.states:V_w_142 c 0.000000E+00 4.250065E-03 1.000000E+20
1146 traj.phases.propelled_ascent.indep_states.states:V_w_143 c 0.000000E+00 3.456327E-03 1.000000E+20
1147 traj.phases.propelled_ascent.indep_states.states:V_w_144 c 0.000000E+00 3.205738E-03 1.000000E+20
1148 traj.phases.propelled_ascent.indep_states.states:V_w_145 c 0.000000E+00 2.633045E-03 1.000000E+20
1149 traj.phases.propelled_ascent.indep_states.states:V_w_146 c 0.000000E+00 1.845409E-03 1.000000E+20
1150 traj.phases.propelled_ascent.indep_states.states:V_w_147 c 0.000000E+00 1.596741E-03 1.000000E+20
1151 traj.phases.propelled_ascent.indep_states.states:V_w_148 c 0.000000E+00 1.028424E-03 1.000000E+20
1152 traj.phases.propelled_ascent.indep_states.states:V_w_149 c 0.000000E+00 2.467821E-04 1.000000E+20
Constraints (i - inequality, e - equality)
Index Name Type Lower Value Upper Status Lagrange Multiplier (N/A)
0 traj.linkages.propelled_ascent:time_final|ballistic_ascent:time_initial e 0.000000E+00 -1.387779E-17 0.000000E+00 9.00000E+100
1 traj.linkages.propelled_ascent:r_final|ballistic_ascent:r_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
2 traj.linkages.propelled_ascent:h_final|ballistic_ascent:h_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
3 traj.linkages.propelled_ascent:gam_final|ballistic_ascent:gam_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
4 traj.linkages.propelled_ascent:v_final|ballistic_ascent:v_initial e 0.000000E+00 -7.105427E-15 0.000000E+00 9.00000E+100
5 traj.linkages.ballistic_ascent:time_final|descent:time_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
6 traj.linkages.ballistic_ascent:r_final|descent:r_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
7 traj.linkages.ballistic_ascent:h_final|descent:h_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
8 traj.linkages.ballistic_ascent:gam_final|descent:gam_initial e 0.000000E+00 -5.708043E-29 0.000000E+00 9.00000E+100
9 traj.linkages.ballistic_ascent:v_final|descent:v_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
10 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.426338E-15 0.000000E+00 9.00000E+100
11 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.027901E-15 0.000000E+00 9.00000E+100
12 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.824775E-15 0.000000E+00 9.00000E+100
13 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.824775E-15 0.000000E+00 9.00000E+100
14 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.537944E-15 0.000000E+00 9.00000E+100
15 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.426338E-15 0.000000E+00 9.00000E+100
16 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.062165E-14 0.000000E+00 9.00000E+100
17 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.426338E-15 0.000000E+00 9.00000E+100
18 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.768972E-15 0.000000E+00 9.00000E+100
19 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.426338E-16 0.000000E+00 9.00000E+100
20 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.482141E-15 0.000000E+00 9.00000E+100
21 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 6.167409E-15 0.000000E+00 9.00000E+100
22 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.083704E-15 0.000000E+00 9.00000E+100
23 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 2.741071E-15 0.000000E+00 9.00000E+100
24 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 6.510043E-15 0.000000E+00 9.00000E+100
25 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
26 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.111606E-15 0.000000E+00 9.00000E+100
27 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 1.850223E-14 0.000000E+00 9.00000E+100
28 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 1.678906E-14 0.000000E+00 9.00000E+100
29 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -9.593747E-15 0.000000E+00 9.00000E+100
30 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.884486E-14 0.000000E+00 9.00000E+100
31 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.267745E-14 0.000000E+00 9.00000E+100
32 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.083704E-15 0.000000E+00 9.00000E+100
33 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.768972E-15 0.000000E+00 9.00000E+100
34 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.843861E-14 0.000000E+00 9.00000E+100
35 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.130692E-14 0.000000E+00 9.00000E+100
36 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 1.027901E-15 0.000000E+00 9.00000E+100
37 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.027901E-15 0.000000E+00 9.00000E+100
38 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.083704E-15 0.000000E+00 9.00000E+100
39 traj.phases.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 6.510043E-15 0.000000E+00 9.00000E+100
40 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.370535E-15 0.000000E+00 9.00000E+100
41 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.055803E-15 0.000000E+00 9.00000E+100
42 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.852676E-16 0.000000E+00 9.00000E+100
43 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.199218E-14 0.000000E+00 9.00000E+100
44 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 2.055803E-15 0.000000E+00 9.00000E+100
45 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.454240E-15 0.000000E+00 9.00000E+100
46 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.852676E-16 0.000000E+00 9.00000E+100
47 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.027901E-15 0.000000E+00 9.00000E+100
48 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.852676E-16 0.000000E+00 9.00000E+100
49 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.681359E-15 0.000000E+00 9.00000E+100
50 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.713169E-16 0.000000E+00 9.00000E+100
51 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.597655E-15 0.000000E+00 9.00000E+100
52 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.569754E-15 0.000000E+00 9.00000E+100
53 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.852676E-16 0.000000E+00 9.00000E+100
54 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.199218E-15 0.000000E+00 9.00000E+100
55 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.426338E-15 0.000000E+00 9.00000E+100
56 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.027901E-14 0.000000E+00 9.00000E+100
57 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 5.824775E-15 0.000000E+00 9.00000E+100
58 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.597655E-15 0.000000E+00 9.00000E+100
59 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 6.852676E-16 0.000000E+00 9.00000E+100
60 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 6.338726E-15 0.000000E+00 9.00000E+100
61 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 5.910433E-15 0.000000E+00 9.00000E+100
62 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.282923E-16 0.000000E+00 9.00000E+100
63 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.940289E-15 0.000000E+00 9.00000E+100
64 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 7.794919E-15 0.000000E+00 9.00000E+100
65 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -7.623602E-15 0.000000E+00 9.00000E+100
66 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.344838E-14 0.000000E+00 9.00000E+100
67 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.638530E-16 0.000000E+00 9.00000E+100
68 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 4.154435E-15 0.000000E+00 9.00000E+100
69 traj.phases.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.525791E-15 0.000000E+00 9.00000E+100
70 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.903521E-16 0.000000E+00 9.00000E+100
71 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.043028E-17 0.000000E+00 9.00000E+100
72 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.637028E-16 0.000000E+00 9.00000E+100
73 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.710564E-17 0.000000E+00 9.00000E+100
74 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -7.614085E-18 0.000000E+00 9.00000E+100
75 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.694134E-16 0.000000E+00 9.00000E+100
76 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.807042E-17 0.000000E+00 9.00000E+100
77 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.045634E-17 0.000000E+00 9.00000E+100
78 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.008866E-16 0.000000E+00 9.00000E+100
79 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.865451E-16 0.000000E+00 9.00000E+100
80 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.994789E-17 0.000000E+00 9.00000E+100
81 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.360366E-16 0.000000E+00 9.00000E+100
82 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.517606E-17 0.000000E+00 9.00000E+100
83 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
84 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.568451E-17 0.000000E+00 9.00000E+100
85 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.898310E-17 0.000000E+00 9.00000E+100
86 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.807042E-18 0.000000E+00 9.00000E+100
87 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.664930E-17 0.000000E+00 9.00000E+100
88 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.329859E-17 0.000000E+00 9.00000E+100
89 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.471972E-17 0.000000E+00 9.00000E+100
90 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.471972E-17 0.000000E+00 9.00000E+100
91 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.664930E-17 0.000000E+00 9.00000E+100
92 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.426338E-17 0.000000E+00 9.00000E+100
93 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.522817E-17 0.000000E+00 9.00000E+100
94 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.756198E-17 0.000000E+00 9.00000E+100
95 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -7.614085E-18 0.000000E+00 9.00000E+100
96 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -7.614085E-18 0.000000E+00 9.00000E+100
97 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.568451E-17 0.000000E+00 9.00000E+100
98 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.807042E-18 0.000000E+00 9.00000E+100
99 traj.phases.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
100 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.713169E-15 0.000000E+00 9.00000E+100
101 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.565845E-15 0.000000E+00 9.00000E+100
102 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.741071E-15 0.000000E+00 9.00000E+100
103 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.255021E-14 0.000000E+00 9.00000E+100
104 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.164955E-14 0.000000E+00 9.00000E+100
105 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.021540E-14 0.000000E+00 9.00000E+100
106 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.936381E-15 0.000000E+00 9.00000E+100
107 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 5.824775E-15 0.000000E+00 9.00000E+100
108 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.083704E-15 0.000000E+00 9.00000E+100
109 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.282923E-15 0.000000E+00 9.00000E+100
110 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.824775E-15 0.000000E+00 9.00000E+100
111 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 7.709261E-15 0.000000E+00 9.00000E+100
112 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.490457E-14 0.000000E+00 9.00000E+100
113 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 8.737162E-15 0.000000E+00 9.00000E+100
114 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.741071E-15 0.000000E+00 9.00000E+100
115 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 1.284877E-14 0.000000E+00 9.00000E+100
116 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.741071E-15 0.000000E+00 9.00000E+100
117 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.055803E-15 0.000000E+00 9.00000E+100
118 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 4.454240E-15 0.000000E+00 9.00000E+100
119 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 5.139507E-16 0.000000E+00 9.00000E+100
120 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 4.539898E-15 0.000000E+00 9.00000E+100
121 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.282923E-16 0.000000E+00 9.00000E+100
122 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.597655E-15 0.000000E+00 9.00000E+100
123 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 1.456194E-15 0.000000E+00 9.00000E+100
124 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.227120E-15 0.000000E+00 9.00000E+100
125 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 9.422430E-16 0.000000E+00 9.00000E+100
126 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.884486E-15 0.000000E+00 9.00000E+100
127 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 7.195310E-15 0.000000E+00 9.00000E+100
128 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.079796E-15 0.000000E+00 9.00000E+100
129 traj.phases.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 8.608675E-15 0.000000E+00 9.00000E+100
130 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -2.191571E-14 0.000000E+00 9.00000E+100
131 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -9.510593E-15 0.000000E+00 9.00000E+100
132 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -8.683585E-15 0.000000E+00 9.00000E+100
133 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -4.135040E-16 0.000000E+00 9.00000E+100
134 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 4.962048E-15 0.000000E+00 9.00000E+100
135 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -1.488614E-14 0.000000E+00 9.00000E+100
136 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 1.075110E-14 0.000000E+00 9.00000E+100
137 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -2.439674E-14 0.000000E+00 9.00000E+100
138 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -1.240512E-14 0.000000E+00 9.00000E+100
139 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -4.672595E-14 0.000000E+00 9.00000E+100
140 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 7.443072E-15 0.000000E+00 9.00000E+100
141 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 1.281862E-14 0.000000E+00 9.00000E+100
142 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 8.270080E-16 0.000000E+00 9.00000E+100
143 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -1.075110E-14 0.000000E+00 9.00000E+100
144 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 1.385238E-14 0.000000E+00 9.00000E+100
145 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -2.212247E-14 0.000000E+00 9.00000E+100
146 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 1.199162E-14 0.000000E+00 9.00000E+100
147 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -2.315623E-14 0.000000E+00 9.00000E+100
148 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -3.700861E-14 0.000000E+00 9.00000E+100
149 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -6.202560E-16 0.000000E+00 9.00000E+100
150 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -1.447264E-14 0.000000E+00 9.00000E+100
151 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -7.856576E-15 0.000000E+00 9.00000E+100
152 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -1.943469E-14 0.000000E+00 9.00000E+100
153 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 2.356973E-14 0.000000E+00 9.00000E+100
154 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 1.343888E-14 0.000000E+00 9.00000E+100
155 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -3.928288E-15 0.000000E+00 9.00000E+100
156 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -2.356973E-14 0.000000E+00 9.00000E+100
157 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -2.274272E-14 0.000000E+00 9.00000E+100
158 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 1.095786E-14 0.000000E+00 9.00000E+100
159 traj.phases.descent.collocation_constraint.defects:r e 0.000000E+00 -1.385238E-14 0.000000E+00 9.00000E+100
160 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -1.421085E-14 0.000000E+00 9.00000E+100
161 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -3.385564E-15 0.000000E+00 9.00000E+100
162 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 4.160884E-15 0.000000E+00 9.00000E+100
163 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 1.188824E-14 0.000000E+00 9.00000E+100
164 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -9.820721E-16 0.000000E+00 9.00000E+100
165 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 2.377648E-15 0.000000E+00 9.00000E+100
166 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -8.993713E-15 0.000000E+00 9.00000E+100
167 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 6.616064E-15 0.000000E+00 9.00000E+100
168 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -6.202560E-15 0.000000E+00 9.00000E+100
169 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -9.303841E-16 0.000000E+00 9.00000E+100
170 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -2.584400E-15 0.000000E+00 9.00000E+100
171 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -1.261187E-14 0.000000E+00 9.00000E+100
172 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -8.270080E-15 0.000000E+00 9.00000E+100
173 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 3.928288E-15 0.000000E+00 9.00000E+100
174 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -9.303841E-15 0.000000E+00 9.00000E+100
175 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 2.481024E-15 0.000000E+00 9.00000E+100
176 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 2.067520E-16 0.000000E+00 9.00000E+100
177 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -1.240512E-15 0.000000E+00 9.00000E+100
178 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -2.894528E-15 0.000000E+00 9.00000E+100
179 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 1.447264E-15 0.000000E+00 9.00000E+100
180 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -1.033760E-15 0.000000E+00 9.00000E+100
181 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 -2.481024E-15 0.000000E+00 9.00000E+100
182 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 3.928288E-15 0.000000E+00 9.00000E+100
183 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 2.481024E-15 0.000000E+00 9.00000E+100
184 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 7.236320E-15 0.000000E+00 9.00000E+100
185 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 2.894528E-15 0.000000E+00 9.00000E+100
186 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 4.962048E-15 0.000000E+00 9.00000E+100
187 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 3.721536E-15 0.000000E+00 9.00000E+100
188 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 1.240512E-15 0.000000E+00 9.00000E+100
189 traj.phases.descent.collocation_constraint.defects:h e 0.000000E+00 1.240512E-15 0.000000E+00 9.00000E+100
190 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 2.894528E-15 0.000000E+00 9.00000E+100
191 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -4.135040E-16 0.000000E+00 9.00000E+100
192 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -4.135040E-16 0.000000E+00 9.00000E+100
193 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 1.240512E-15 0.000000E+00 9.00000E+100
194 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 2.067520E-15 0.000000E+00 9.00000E+100
195 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 4.548544E-15 0.000000E+00 9.00000E+100
196 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 4.135040E-16 0.000000E+00 9.00000E+100
197 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 8.270080E-15 0.000000E+00 9.00000E+100
198 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 6.616064E-15 0.000000E+00 9.00000E+100
199 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 9.097089E-15 0.000000E+00 9.00000E+100
200 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -8.270080E-16 0.000000E+00 9.00000E+100
201 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.240512E-15 0.000000E+00 9.00000E+100
202 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 8.270080E-15 0.000000E+00 9.00000E+100
203 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -2.894528E-15 0.000000E+00 9.00000E+100
204 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 9.510593E-15 0.000000E+00 9.00000E+100
205 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.778067E-14 0.000000E+00 9.00000E+100
206 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -8.270080E-16 0.000000E+00 9.00000E+100
207 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -6.202560E-15 0.000000E+00 9.00000E+100
208 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 8.270080E-15 0.000000E+00 9.00000E+100
209 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.240512E-15 0.000000E+00 9.00000E+100
210 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.695366E-14 0.000000E+00 9.00000E+100
211 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -2.935879E-14 0.000000E+00 9.00000E+100
212 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 9.924097E-15 0.000000E+00 9.00000E+100
213 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -9.924097E-15 0.000000E+00 9.00000E+100
214 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.695366E-14 0.000000E+00 9.00000E+100
215 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -8.683585E-15 0.000000E+00 9.00000E+100
216 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 1.778067E-14 0.000000E+00 9.00000E+100
217 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -4.548544E-15 0.000000E+00 9.00000E+100
218 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 9.097089E-15 0.000000E+00 9.00000E+100
219 traj.phases.descent.collocation_constraint.defects:gam e 0.000000E+00 -7.856576E-15 0.000000E+00 9.00000E+100
220 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 1.891781E-14 0.000000E+00 9.00000E+100
221 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 4.135040E-15 0.000000E+00 9.00000E+100
222 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -3.101280E-15 0.000000E+00 9.00000E+100
223 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -5.995808E-15 0.000000E+00 9.00000E+100
224 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -4.341792E-15 0.000000E+00 9.00000E+100
225 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 7.029568E-15 0.000000E+00 9.00000E+100
226 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 3.333876E-15 0.000000E+00 9.00000E+100
227 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -1.912456E-15 0.000000E+00 9.00000E+100
228 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 4.026818E-15 0.000000E+00 9.00000E+100
229 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -8.021332E-15 0.000000E+00 9.00000E+100
230 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 2.196740E-16 0.000000E+00 9.00000E+100
231 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -4.858672E-15 0.000000E+00 9.00000E+100
232 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -7.262164E-15 0.000000E+00 9.00000E+100
233 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 3.644004E-15 0.000000E+00 9.00000E+100
234 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -5.168800E-16 0.000000E+00 9.00000E+100
235 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -3.411408E-15 0.000000E+00 9.00000E+100
236 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -4.445168E-15 0.000000E+00 9.00000E+100
237 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 5.427240E-15 0.000000E+00 9.00000E+100
238 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -1.498952E-15 0.000000E+00 9.00000E+100
239 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 4.703608E-15 0.000000E+00 9.00000E+100
240 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 2.274272E-15 0.000000E+00 9.00000E+100
241 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 6.150872E-15 0.000000E+00 9.00000E+100
242 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 1.602328E-15 0.000000E+00 9.00000E+100
243 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 4.755296E-15 0.000000E+00 9.00000E+100
244 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -1.493783E-14 0.000000E+00 9.00000E+100
245 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 1.033760E-15 0.000000E+00 9.00000E+100
246 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -5.633992E-15 0.000000E+00 9.00000E+100
247 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 -4.445168E-15 0.000000E+00 9.00000E+100
248 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 5.272176E-15 0.000000E+00 9.00000E+100
249 traj.phases.descent.collocation_constraint.defects:v e 0.000000E+00 3.514784E-15 0.000000E+00 9.00000E+100
250 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
251 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
252 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
253 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.000526E-21 0.000000E+00 9.00000E+100
254 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.000526E-21 0.000000E+00 9.00000E+100
255 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.000105E-20 0.000000E+00 9.00000E+100
256 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.000526E-21 0.000000E+00 9.00000E+100
257 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.500789E-21 0.000000E+00 9.00000E+100
258 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.000526E-21 0.000000E+00 9.00000E+100
259 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.500263E-20 0.000000E+00 9.00000E+100
260 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.500263E-21 0.000000E+00 9.00000E+100
261 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
262 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
263 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.000526E-21 0.000000E+00 9.00000E+100
264 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.500158E-20 0.000000E+00 9.00000E+100
265 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.000211E-20 0.000000E+00 9.00000E+100
266 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.000526E-21 0.000000E+00 9.00000E+100
267 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.000211E-20 0.000000E+00 9.00000E+100
268 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.200232E-19 0.000000E+00 9.00000E+100
269 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.000105E-19 0.000000E+00 9.00000E+100
270 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.600168E-19 0.000000E+00 9.00000E+100
271 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.500368E-20 0.000000E+00 9.00000E+100
272 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.000211E-20 0.000000E+00 9.00000E+100
273 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.800189E-19 0.000000E+00 9.00000E+100
274 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.500158E-19 0.000000E+00 9.00000E+100
275 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.300137E-19 0.000000E+00 9.00000E+100
276 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.000211E-19 0.000000E+00 9.00000E+100
277 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.200232E-19 0.000000E+00 9.00000E+100
278 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.000421E-20 0.000000E+00 9.00000E+100
279 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.100116E-19 0.000000E+00 9.00000E+100
280 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.000526E-20 0.000000E+00 9.00000E+100
281 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.300242E-19 0.000000E+00 9.00000E+100
282 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 4.000421E-20 0.000000E+00 9.00000E+100
283 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 6.200653E-19 0.000000E+00 9.00000E+100
284 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.900200E-19 0.000000E+00 9.00000E+100
285 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.100326E-19 0.000000E+00 9.00000E+100
286 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.000105E-19 0.000000E+00 9.00000E+100
287 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.200232E-19 0.000000E+00 9.00000E+100
288 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.500158E-19 0.000000E+00 9.00000E+100
289 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.200232E-19 0.000000E+00 9.00000E+100
290 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.000316E-20 0.000000E+00 9.00000E+100
291 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.100642E-19 0.000000E+00 9.00000E+100
292 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.500158E-19 0.000000E+00 9.00000E+100
293 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.400253E-19 0.000000E+00 9.00000E+100
294 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.200337E-19 0.000000E+00 9.00000E+100
295 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.000316E-19 0.000000E+00 9.00000E+100
296 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.200442E-19 0.000000E+00 9.00000E+100
297 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.600379E-19 0.000000E+00 9.00000E+100
298 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.080114E-18 0.000000E+00 9.00000E+100
299 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
300 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 6.600695E-19 0.000000E+00 9.00000E+100
301 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.660175E-18 0.000000E+00 9.00000E+100
302 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.600168E-19 0.000000E+00 9.00000E+100
303 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 9.601010E-19 0.000000E+00 9.00000E+100
304 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.760185E-18 0.000000E+00 9.00000E+100
305 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.200758E-19 0.000000E+00 9.00000E+100
306 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.640173E-18 0.000000E+00 9.00000E+100
307 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.800189E-19 0.000000E+00 9.00000E+100
308 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
309 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.400463E-19 0.000000E+00 9.00000E+100
310 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.160543E-18 0.000000E+00 9.00000E+100
311 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.160122E-18 0.000000E+00 9.00000E+100
312 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 9.801031E-19 0.000000E+00 9.00000E+100
313 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.680177E-18 0.000000E+00 9.00000E+100
314 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.200442E-19 0.000000E+00 9.00000E+100
315 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.200337E-19 0.000000E+00 9.00000E+100
316 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.060217E-18 0.000000E+00 9.00000E+100
317 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.400568E-19 0.000000E+00 9.00000E+100
318 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.800821E-19 0.000000E+00 9.00000E+100
319 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.200126E-18 0.000000E+00 9.00000E+100
320 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.000526E-19 0.000000E+00 9.00000E+100
321 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.400253E-19 0.000000E+00 9.00000E+100
322 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.320244E-18 0.000000E+00 9.00000E+100
323 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.000211E-19 0.000000E+00 9.00000E+100
324 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.120223E-18 0.000000E+00 9.00000E+100
325 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 4.000421E-20 0.000000E+00 9.00000E+100
326 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.800821E-19 0.000000E+00 9.00000E+100
327 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.180124E-18 0.000000E+00 9.00000E+100
328 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.240341E-18 0.000000E+00 9.00000E+100
329 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.200758E-19 0.000000E+00 9.00000E+100
330 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.160122E-18 0.000000E+00 9.00000E+100
331 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.360354E-18 0.000000E+00 9.00000E+100
332 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.400147E-19 0.000000E+00 9.00000E+100
333 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.200337E-19 0.000000E+00 9.00000E+100
334 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.580693E-18 0.000000E+00 9.00000E+100
335 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.320139E-18 0.000000E+00 9.00000E+100
336 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.600589E-19 0.000000E+00 9.00000E+100
337 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.082114E-17 0.000000E+00 9.00000E+100
338 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 4.600484E-18 0.000000E+00 9.00000E+100
339 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.560480E-18 0.000000E+00 9.00000E+100
340 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.600274E-18 0.000000E+00 9.00000E+100
341 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.440257E-18 0.000000E+00 9.00000E+100
342 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.080535E-18 0.000000E+00 9.00000E+100
343 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.600379E-18 0.000000E+00 9.00000E+100
344 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 8.400884E-19 0.000000E+00 9.00000E+100
345 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.680493E-18 0.000000E+00 9.00000E+100
346 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.080114E-18 0.000000E+00 9.00000E+100
347 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.200547E-19 0.000000E+00 9.00000E+100
348 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 4.000421E-20 0.000000E+00 9.00000E+100
349 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.640278E-18 0.000000E+00 9.00000E+100
350 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.600800E-19 0.000000E+00 9.00000E+100
351 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.320139E-18 0.000000E+00 9.00000E+100
352 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.160333E-18 0.000000E+00 9.00000E+100
353 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.000421E-19 0.000000E+00 9.00000E+100
354 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.800189E-18 0.000000E+00 9.00000E+100
355 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.800400E-18 0.000000E+00 9.00000E+100
356 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.600168E-18 0.000000E+00 9.00000E+100
357 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.600168E-19 0.000000E+00 9.00000E+100
358 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.520686E-18 0.000000E+00 9.00000E+100
359 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.800716E-19 0.000000E+00 9.00000E+100
360 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.120118E-18 0.000000E+00 9.00000E+100
361 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 9.601010E-19 0.000000E+00 9.00000E+100
362 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.200758E-19 0.000000E+00 9.00000E+100
363 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.480156E-18 0.000000E+00 9.00000E+100
364 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.800189E-18 0.000000E+00 9.00000E+100
365 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.600800E-19 0.000000E+00 9.00000E+100
366 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.360248E-18 0.000000E+00 9.00000E+100
367 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.000737E-18 0.000000E+00 9.00000E+100
368 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.400358E-18 0.000000E+00 9.00000E+100
369 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.920834E-18 0.000000E+00 9.00000E+100
370 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 8.960943E-18 0.000000E+00 9.00000E+100
371 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.200758E-19 0.000000E+00 9.00000E+100
372 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.400358E-18 0.000000E+00 9.00000E+100
373 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.600379E-18 0.000000E+00 9.00000E+100
374 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.200547E-19 0.000000E+00 9.00000E+100
375 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.080535E-18 0.000000E+00 9.00000E+100
376 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.680387E-18 0.000000E+00 9.00000E+100
377 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.520792E-18 0.000000E+00 9.00000E+100
378 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 9.601010E-19 0.000000E+00 9.00000E+100
379 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.680282E-18 0.000000E+00 9.00000E+100
380 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.280345E-18 0.000000E+00 9.00000E+100
381 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.920307E-18 0.000000E+00 9.00000E+100
382 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.176124E-17 0.000000E+00 9.00000E+100
383 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.480156E-18 0.000000E+00 9.00000E+100
384 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.000211E-19 0.000000E+00 9.00000E+100
385 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.600589E-19 0.000000E+00 9.00000E+100
386 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.400674E-18 0.000000E+00 9.00000E+100
387 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.200547E-18 0.000000E+00 9.00000E+100
388 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.592168E-17 0.000000E+00 9.00000E+100
389 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.400253E-19 0.000000E+00 9.00000E+100
390 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.280240E-18 0.000000E+00 9.00000E+100
391 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.000526E-18 0.000000E+00 9.00000E+100
392 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.800716E-19 0.000000E+00 9.00000E+100
393 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.600274E-18 0.000000E+00 9.00000E+100
394 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.520792E-18 0.000000E+00 9.00000E+100
395 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.320139E-18 0.000000E+00 9.00000E+100
396 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.320349E-18 0.000000E+00 9.00000E+100
397 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -9.320981E-18 0.000000E+00 9.00000E+100
398 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.920623E-18 0.000000E+00 9.00000E+100
399 traj.phases.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.600379E-18 0.000000E+00 9.00000E+100
400 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.969062E-21 0.000000E+00 9.00000E+100
401 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.875197E-21 0.000000E+00 9.00000E+100
402 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.250132E-21 0.000000E+00 9.00000E+100
403 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.250132E-20 0.000000E+00 9.00000E+100
404 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.375145E-20 0.000000E+00 9.00000E+100
405 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.000105E-20 0.000000E+00 9.00000E+100
406 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.000316E-20 0.000000E+00 9.00000E+100
407 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.500158E-20 0.000000E+00 9.00000E+100
408 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.000105E-20 0.000000E+00 9.00000E+100
409 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -5.000526E-20 0.000000E+00 9.00000E+100
410 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.500474E-20 0.000000E+00 9.00000E+100
411 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.000842E-20 0.000000E+00 9.00000E+100
412 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -5.500579E-20 0.000000E+00 9.00000E+100
413 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.000211E-20 0.000000E+00 9.00000E+100
414 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.500263E-20 0.000000E+00 9.00000E+100
415 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.000632E-20 0.000000E+00 9.00000E+100
416 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.000211E-20 0.000000E+00 9.00000E+100
417 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.000526E-20 0.000000E+00 9.00000E+100
418 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.250132E-19 0.000000E+00 9.00000E+100
419 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.500789E-20 0.000000E+00 9.00000E+100
420 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.500474E-20 0.000000E+00 9.00000E+100
421 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.000526E-20 0.000000E+00 9.00000E+100
422 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.000105E-20 0.000000E+00 9.00000E+100
423 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.500474E-20 0.000000E+00 9.00000E+100
424 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.650384E-19 0.000000E+00 9.00000E+100
425 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -5.000526E-20 0.000000E+00 9.00000E+100
426 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.000526E-20 0.000000E+00 9.00000E+100
427 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.100221E-19 0.000000E+00 9.00000E+100
428 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.400147E-19 0.000000E+00 9.00000E+100
429 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.000211E-20 0.000000E+00 9.00000E+100
430 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.000105E-20 0.000000E+00 9.00000E+100
431 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.900200E-19 0.000000E+00 9.00000E+100
432 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.200126E-19 0.000000E+00 9.00000E+100
433 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.000316E-19 0.000000E+00 9.00000E+100
434 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.100326E-19 0.000000E+00 9.00000E+100
435 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.800189E-19 0.000000E+00 9.00000E+100
436 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -9.000947E-20 0.000000E+00 9.00000E+100
437 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.400147E-19 0.000000E+00 9.00000E+100
438 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.100326E-19 0.000000E+00 9.00000E+100
439 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.500158E-19 0.000000E+00 9.00000E+100
440 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.700179E-19 0.000000E+00 9.00000E+100
441 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.500158E-19 0.000000E+00 9.00000E+100
442 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.000316E-20 0.000000E+00 9.00000E+100
443 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.000737E-20 0.000000E+00 9.00000E+100
444 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.600274E-19 0.000000E+00 9.00000E+100
445 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.000211E-20 0.000000E+00 9.00000E+100
446 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.300242E-19 0.000000E+00 9.00000E+100
447 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.800189E-19 0.000000E+00 9.00000E+100
448 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.500368E-19 0.000000E+00 9.00000E+100
449 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.700389E-19 0.000000E+00 9.00000E+100
450 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.000632E-20 0.000000E+00 9.00000E+100
451 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.100642E-19 0.000000E+00 9.00000E+100
452 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.000316E-20 0.000000E+00 9.00000E+100
453 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.800716E-19 0.000000E+00 9.00000E+100
454 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.801031E-19 0.000000E+00 9.00000E+100
455 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.600274E-19 0.000000E+00 9.00000E+100
456 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
457 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.800295E-19 0.000000E+00 9.00000E+100
458 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -7.000737E-19 0.000000E+00 9.00000E+100
459 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.200653E-19 0.000000E+00 9.00000E+100
460 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.800505E-19 0.000000E+00 9.00000E+100
461 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.601010E-19 0.000000E+00 9.00000E+100
462 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.680177E-18 0.000000E+00 9.00000E+100
463 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.200758E-19 0.000000E+00 9.00000E+100
464 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.801031E-19 0.000000E+00 9.00000E+100
465 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.400147E-19 0.000000E+00 9.00000E+100
466 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.620171E-18 0.000000E+00 9.00000E+100
467 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.400358E-19 0.000000E+00 9.00000E+100
468 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.400674E-19 0.000000E+00 9.00000E+100
469 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.440257E-18 0.000000E+00 9.00000E+100
470 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -5.000526E-19 0.000000E+00 9.00000E+100
471 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.800926E-19 0.000000E+00 9.00000E+100
472 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.000632E-19 0.000000E+00 9.00000E+100
473 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.200126E-18 0.000000E+00 9.00000E+100
474 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.200758E-19 0.000000E+00 9.00000E+100
475 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.880198E-18 0.000000E+00 9.00000E+100
476 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.100116E-18 0.000000E+00 9.00000E+100
477 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.000105E-19 0.000000E+00 9.00000E+100
478 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.920307E-18 0.000000E+00 9.00000E+100
479 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -7.000737E-19 0.000000E+00 9.00000E+100
480 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.600484E-19 0.000000E+00 9.00000E+100
481 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.200126E-18 0.000000E+00 9.00000E+100
482 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.800821E-19 0.000000E+00 9.00000E+100
483 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.600484E-19 0.000000E+00 9.00000E+100
484 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.480366E-18 0.000000E+00 9.00000E+100
485 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.080114E-18 0.000000E+00 9.00000E+100
486 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.400884E-19 0.000000E+00 9.00000E+100
487 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.360248E-18 0.000000E+00 9.00000E+100
488 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.400147E-19 0.000000E+00 9.00000E+100
489 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -7.400779E-19 0.000000E+00 9.00000E+100
490 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.000632E-19 0.000000E+00 9.00000E+100
491 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.520265E-18 0.000000E+00 9.00000E+100
492 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.200126E-19 0.000000E+00 9.00000E+100
493 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.000211E-18 0.000000E+00 9.00000E+100
494 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.200337E-19 0.000000E+00 9.00000E+100
495 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.600905E-19 0.000000E+00 9.00000E+100
496 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.140330E-18 0.000000E+00 9.00000E+100
497 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.500158E-18 0.000000E+00 9.00000E+100
498 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.220444E-18 0.000000E+00 9.00000E+100
499 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.520370E-18 0.000000E+00 9.00000E+100
500 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.120223E-18 0.000000E+00 9.00000E+100
501 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.000632E-19 0.000000E+00 9.00000E+100
502 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.720181E-18 0.000000E+00 9.00000E+100
503 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.340141E-18 0.000000E+00 9.00000E+100
504 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.000632E-19 0.000000E+00 9.00000E+100
505 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.380672E-18 0.000000E+00 9.00000E+100
506 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.700389E-18 0.000000E+00 9.00000E+100
507 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.280135E-18 0.000000E+00 9.00000E+100
508 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.400674E-19 0.000000E+00 9.00000E+100
509 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.640278E-18 0.000000E+00 9.00000E+100
510 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.920413E-18 0.000000E+00 9.00000E+100
511 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.601010E-19 0.000000E+00 9.00000E+100
512 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.200337E-19 0.000000E+00 9.00000E+100
513 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.320244E-18 0.000000E+00 9.00000E+100
514 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.640383E-18 0.000000E+00 9.00000E+100
515 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.440467E-18 0.000000E+00 9.00000E+100
516 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.480261E-18 0.000000E+00 9.00000E+100
517 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.240131E-18 0.000000E+00 9.00000E+100
518 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.000842E-20 0.000000E+00 9.00000E+100
519 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.400253E-19 0.000000E+00 9.00000E+100
520 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.800295E-18 0.000000E+00 9.00000E+100
521 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.160122E-18 0.000000E+00 9.00000E+100
522 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.800716E-19 0.000000E+00 9.00000E+100
523 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.560269E-18 0.000000E+00 9.00000E+100
524 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.360354E-18 0.000000E+00 9.00000E+100
525 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.000842E-19 0.000000E+00 9.00000E+100
526 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.440152E-18 0.000000E+00 9.00000E+100
527 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.720286E-18 0.000000E+00 9.00000E+100
528 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.400674E-19 0.000000E+00 9.00000E+100
529 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.800926E-19 0.000000E+00 9.00000E+100
530 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.000211E-18 0.000000E+00 9.00000E+100
531 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.720286E-18 0.000000E+00 9.00000E+100
532 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.280661E-18 0.000000E+00 9.00000E+100
533 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.960312E-18 0.000000E+00 9.00000E+100
534 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.360143E-18 0.000000E+00 9.00000E+100
535 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.720181E-18 0.000000E+00 9.00000E+100
536 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.160122E-18 0.000000E+00 9.00000E+100
537 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.000842E-20 0.000000E+00 9.00000E+100
538 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.000421E-18 0.000000E+00 9.00000E+100
539 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.120855E-18 0.000000E+00 9.00000E+100
540 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.000316E-18 0.000000E+00 9.00000E+100
541 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.080219E-18 0.000000E+00 9.00000E+100
542 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.200758E-19 0.000000E+00 9.00000E+100
543 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.880724E-18 0.000000E+00 9.00000E+100
544 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.000211E-18 0.000000E+00 9.00000E+100
545 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.000421E-19 0.000000E+00 9.00000E+100
546 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.480261E-18 0.000000E+00 9.00000E+100
547 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -7.600800E-18 0.000000E+00 9.00000E+100
548 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.320244E-18 0.000000E+00 9.00000E+100
549 traj.phases.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.280135E-18 0.000000E+00 9.00000E+100
550 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.365477E-16 0.000000E+00 9.00000E+100
551 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.249757E-17 0.000000E+00 9.00000E+100
552 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.507714E-16 0.000000E+00 9.00000E+100
553 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.813524E-16 0.000000E+00 9.00000E+100
554 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.689488E-18 0.000000E+00 9.00000E+100
555 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.877531E-16 0.000000E+00 9.00000E+100
556 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.400674E-17 0.000000E+00 9.00000E+100
557 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.640909E-17 0.000000E+00 9.00000E+100
558 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.308582E-16 0.000000E+00 9.00000E+100
559 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.200337E-16 0.000000E+00 9.00000E+100
560 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.230352E-16 0.000000E+00 9.00000E+100
561 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.792189E-16 0.000000E+00 9.00000E+100
562 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.307015E-17 0.000000E+00 9.00000E+100
563 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.467032E-17 0.000000E+00 9.00000E+100
564 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.921196E-16 0.000000E+00 9.00000E+100
565 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.110655E-16 0.000000E+00 9.00000E+100
566 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.574130E-17 0.000000E+00 9.00000E+100
567 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -7.111860E-17 0.000000E+00 9.00000E+100
568 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.939970E-16 0.000000E+00 9.00000E+100
569 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.569791E-17 0.000000E+00 9.00000E+100
570 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.564609E-16 0.000000E+00 9.00000E+100
571 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.302675E-17 0.000000E+00 9.00000E+100
572 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.386813E-17 0.000000E+00 9.00000E+100
573 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.153115E-16 0.000000E+00 9.00000E+100
574 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.268683E-16 0.000000E+00 9.00000E+100
575 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.986981E-17 0.000000E+00 9.00000E+100
576 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.777965E-17 0.000000E+00 9.00000E+100
577 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.475711E-17 0.000000E+00 9.00000E+100
578 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.636570E-17 0.000000E+00 9.00000E+100
579 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.848195E-16 0.000000E+00 9.00000E+100
580 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.350364E-16 0.000000E+00 9.00000E+100
581 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.057889E-17 0.000000E+00 9.00000E+100
582 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.915757E-16 0.000000E+00 9.00000E+100
583 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.164567E-17 0.000000E+00 9.00000E+100
584 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.213461E-16 0.000000E+00 9.00000E+100
585 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.978512E-17 0.000000E+00 9.00000E+100
586 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.866863E-18 0.000000E+00 9.00000E+100
587 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.022330E-17 0.000000E+00 9.00000E+100
588 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.289025E-17 0.000000E+00 9.00000E+100
589 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.777181E-16 0.000000E+00 9.00000E+100
590 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.665953E-16 0.000000E+00 9.00000E+100
591 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.947082E-17 0.000000E+00 9.00000E+100
592 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.879625E-16 0.000000E+00 9.00000E+100
593 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.267689E-16 0.000000E+00 9.00000E+100
594 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.646606E-16 0.000000E+00 9.00000E+100
595 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.739739E-16 0.000000E+00 9.00000E+100
596 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.115989E-17 0.000000E+00 9.00000E+100
597 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.561053E-16 0.000000E+00 9.00000E+100
598 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.369033E-17 0.000000E+00 9.00000E+100
599 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.289656E-17 0.000000E+00 9.00000E+100
600 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.712028E-18 0.000000E+00 9.00000E+100
601 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.827385E-17 0.000000E+00 9.00000E+100
602 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.654139E-17 0.000000E+00 9.00000E+100
603 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.164988E-17 0.000000E+00 9.00000E+100
604 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.198348E-16 0.000000E+00 9.00000E+100
605 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.498251E-17 0.000000E+00 9.00000E+100
606 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.302886E-17 0.000000E+00 9.00000E+100
607 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.724836E-17 0.000000E+00 9.00000E+100
608 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.586834E-16 0.000000E+00 9.00000E+100
609 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.548607E-16 0.000000E+00 9.00000E+100
610 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.196102E-17 0.000000E+00 9.00000E+100
611 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.818414E-16 0.000000E+00 9.00000E+100
612 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.445123E-18 0.000000E+00 9.00000E+100
613 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.810074E-16 0.000000E+00 9.00000E+100
614 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.240552E-17 0.000000E+00 9.00000E+100
615 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.247687E-16 0.000000E+00 9.00000E+100
616 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.124668E-16 0.000000E+00 9.00000E+100
617 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.721070E-16 0.000000E+00 9.00000E+100
618 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.062755E-17 0.000000E+00 9.00000E+100
619 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.853739E-17 0.000000E+00 9.00000E+100
620 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.000211E-17 0.000000E+00 9.00000E+100
621 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.138213E-17 0.000000E+00 9.00000E+100
622 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.269911E-16 0.000000E+00 9.00000E+100
623 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.765367E-17 0.000000E+00 9.00000E+100
624 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.622498E-17 0.000000E+00 9.00000E+100
625 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.831854E-16 0.000000E+00 9.00000E+100
626 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.169456E-16 0.000000E+00 9.00000E+100
627 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.247687E-16 0.000000E+00 9.00000E+100
628 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.309916E-16 0.000000E+00 9.00000E+100
629 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.538150E-17 0.000000E+00 9.00000E+100
630 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.413587E-17 0.000000E+00 9.00000E+100
631 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.804190E-16 0.000000E+00 9.00000E+100
632 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.934274E-18 0.000000E+00 9.00000E+100
633 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.818074E-17 0.000000E+00 9.00000E+100
634 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.889193E-18 0.000000E+00 9.00000E+100
635 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.449778E-17 0.000000E+00 9.00000E+100
636 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.102865E-17 0.000000E+00 9.00000E+100
637 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.291013E-16 0.000000E+00 9.00000E+100
638 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.911523E-17 0.000000E+00 9.00000E+100
639 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.968652E-16 0.000000E+00 9.00000E+100
640 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -7.120749E-17 0.000000E+00 9.00000E+100
641 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.876279E-17 0.000000E+00 9.00000E+100
642 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.182768E-17 0.000000E+00 9.00000E+100
643 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.841527E-16 0.000000E+00 9.00000E+100
644 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.367255E-16 0.000000E+00 9.00000E+100
645 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.298020E-17 0.000000E+00 9.00000E+100
646 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.115989E-17 0.000000E+00 9.00000E+100
647 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.312138E-16 0.000000E+00 9.00000E+100
648 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.377923E-16 0.000000E+00 9.00000E+100
649 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.111778E-16 0.000000E+00 9.00000E+100
650 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.235896E-17 0.000000E+00 9.00000E+100
651 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.881087E-16 0.000000E+00 9.00000E+100
652 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.587044E-16 0.000000E+00 9.00000E+100
653 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.745962E-16 0.000000E+00 9.00000E+100
654 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.237674E-16 0.000000E+00 9.00000E+100
655 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.025079E-16 0.000000E+00 9.00000E+100
656 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.823046E-17 0.000000E+00 9.00000E+100
657 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.955867E-17 0.000000E+00 9.00000E+100
658 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.411260E-16 0.000000E+00 9.00000E+100
659 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.240131E-17 0.000000E+00 9.00000E+100
660 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.194792E-16 0.000000E+00 9.00000E+100
661 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.360588E-16 0.000000E+00 9.00000E+100
662 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.029991E-16 0.000000E+00 9.00000E+100
663 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.427133E-17 0.000000E+00 9.00000E+100
664 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.368027E-16 0.000000E+00 9.00000E+100
665 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.645729E-16 0.000000E+00 9.00000E+100
666 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.212128E-16 0.000000E+00 9.00000E+100
667 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.854417E-16 0.000000E+00 9.00000E+100
668 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.145407E-17 0.000000E+00 9.00000E+100
669 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.097999E-17 0.000000E+00 9.00000E+100
670 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.843305E-16 0.000000E+00 9.00000E+100
671 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.925384E-17 0.000000E+00 9.00000E+100
672 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.924425E-16 0.000000E+00 9.00000E+100
673 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.769601E-17 0.000000E+00 9.00000E+100
674 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.253781E-17 0.000000E+00 9.00000E+100
675 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.078733E-17 0.000000E+00 9.00000E+100
676 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.257466E-16 0.000000E+00 9.00000E+100
677 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.273690E-16 0.000000E+00 9.00000E+100
678 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.780293E-17 0.000000E+00 9.00000E+100
679 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.148788E-16 0.000000E+00 9.00000E+100
680 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.689488E-17 0.000000E+00 9.00000E+100
681 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.565241E-17 0.000000E+00 9.00000E+100
682 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.784632E-16 0.000000E+00 9.00000E+100
683 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.526827E-17 0.000000E+00 9.00000E+100
684 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.599724E-16 0.000000E+00 9.00000E+100
685 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.527143E-16 0.000000E+00 9.00000E+100
686 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.538255E-17 0.000000E+00 9.00000E+100
687 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.309354E-16 0.000000E+00 9.00000E+100
688 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.200968E-18 0.000000E+00 9.00000E+100
689 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.509469E-17 0.000000E+00 9.00000E+100
690 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.185014E-16 0.000000E+00 9.00000E+100
691 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.929829E-17 0.000000E+00 9.00000E+100
692 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.574166E-16 0.000000E+00 9.00000E+100
693 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.412371E-16 0.000000E+00 9.00000E+100
694 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.187107E-17 0.000000E+00 9.00000E+100
695 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.940730E-17 0.000000E+00 9.00000E+100
696 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.489151E-18 0.000000E+00 9.00000E+100
697 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -9.449883E-17 0.000000E+00 9.00000E+100
698 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.134342E-16 0.000000E+00 9.00000E+100
699 traj.phases.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.634616E-16 0.000000E+00 9.00000E+100
700 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.400674E-19 0.000000E+00 9.00000E+100
701 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.760606E-18 0.000000E+00 9.00000E+100
702 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -7.040741E-18 0.000000E+00 9.00000E+100
703 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.960943E-18 0.000000E+00 9.00000E+100
704 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.320876E-18 0.000000E+00 9.00000E+100
705 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.408148E-17 0.000000E+00 9.00000E+100
706 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.024108E-17 0.000000E+00 9.00000E+100
707 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -7.680808E-18 0.000000E+00 9.00000E+100
708 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.120539E-18 0.000000E+00 9.00000E+100
709 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.601010E-18 0.000000E+00 9.00000E+100
710 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.400674E-18 0.000000E+00 9.00000E+100
711 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.152121E-17 0.000000E+00 9.00000E+100
712 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.176229E-17 0.000000E+00 9.00000E+100
713 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.200337E-18 0.000000E+00 9.00000E+100
714 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.120539E-18 0.000000E+00 9.00000E+100
715 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.728182E-17 0.000000E+00 9.00000E+100
716 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.840404E-18 0.000000E+00 9.00000E+100
717 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.320876E-18 0.000000E+00 9.00000E+100
718 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.880303E-17 0.000000E+00 9.00000E+100
719 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.920202E-17 0.000000E+00 9.00000E+100
720 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.560269E-18 0.000000E+00 9.00000E+100
721 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.536162E-17 0.000000E+00 9.00000E+100
722 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.320876E-18 0.000000E+00 9.00000E+100
723 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.024108E-17 0.000000E+00 9.00000E+100
724 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.584377E-17 0.000000E+00 9.00000E+100
725 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.344141E-17 0.000000E+00 9.00000E+100
726 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.904411E-17 0.000000E+00 9.00000E+100
727 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.200337E-17 0.000000E+00 9.00000E+100
728 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.560269E-17 0.000000E+00 9.00000E+100
729 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.304243E-17 0.000000E+00 9.00000E+100
730 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.640909E-17 0.000000E+00 9.00000E+100
731 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.480472E-18 0.000000E+00 9.00000E+100
732 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.920202E-17 0.000000E+00 9.00000E+100
733 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.448889E-17 0.000000E+00 9.00000E+100
734 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.328350E-17 0.000000E+00 9.00000E+100
735 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.672492E-17 0.000000E+00 9.00000E+100
736 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.928519E-17 0.000000E+00 9.00000E+100
737 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.264344E-17 0.000000E+00 9.00000E+100
738 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.432256E-17 0.000000E+00 9.00000E+100
739 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.744815E-17 0.000000E+00 9.00000E+100
740 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.792189E-17 0.000000E+00 9.00000E+100
741 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.544478E-17 0.000000E+00 9.00000E+100
742 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.432256E-17 0.000000E+00 9.00000E+100
743 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.024108E-17 0.000000E+00 9.00000E+100
744 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.240236E-17 0.000000E+00 9.00000E+100
745 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.800505E-17 0.000000E+00 9.00000E+100
746 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.280135E-18 0.000000E+00 9.00000E+100
747 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.176229E-17 0.000000E+00 9.00000E+100
748 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.336667E-17 0.000000E+00 9.00000E+100
749 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.920202E-18 0.000000E+00 9.00000E+100
750 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.368249E-17 0.000000E+00 9.00000E+100
751 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.656701E-17 0.000000E+00 9.00000E+100
752 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.280135E-17 0.000000E+00 9.00000E+100
753 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.280135E-18 0.000000E+00 9.00000E+100
754 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -7.552795E-17 0.000000E+00 9.00000E+100
755 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.928519E-17 0.000000E+00 9.00000E+100
756 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.680808E-18 0.000000E+00 9.00000E+100
757 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.536162E-16 0.000000E+00 9.00000E+100
758 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.328350E-17 0.000000E+00 9.00000E+100
759 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.400674E-19 0.000000E+00 9.00000E+100
760 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.280135E-17 0.000000E+00 9.00000E+100
761 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.368249E-17 0.000000E+00 9.00000E+100
762 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.096431E-17 0.000000E+00 9.00000E+100
763 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.401748E-16 0.000000E+00 9.00000E+100
764 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.536162E-17 0.000000E+00 9.00000E+100
765 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.088115E-17 0.000000E+00 9.00000E+100
766 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.760606E-18 0.000000E+00 9.00000E+100
767 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.112222E-17 0.000000E+00 9.00000E+100
768 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.736498E-17 0.000000E+00 9.00000E+100
769 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.472155E-17 0.000000E+00 9.00000E+100
770 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.040741E-18 0.000000E+00 9.00000E+100
771 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.496263E-17 0.000000E+00 9.00000E+100
772 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.048216E-16 0.000000E+00 9.00000E+100
773 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.328350E-17 0.000000E+00 9.00000E+100
774 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.304243E-17 0.000000E+00 9.00000E+100
775 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.285040E-16 0.000000E+00 9.00000E+100
776 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.120539E-17 0.000000E+00 9.00000E+100
777 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.016633E-17 0.000000E+00 9.00000E+100
778 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.816296E-17 0.000000E+00 9.00000E+100
779 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 5.568586E-17 0.000000E+00 9.00000E+100
780 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.824613E-17 0.000000E+00 9.00000E+100
781 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.496263E-17 0.000000E+00 9.00000E+100
782 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.912728E-17 0.000000E+00 9.00000E+100
783 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.864512E-17 0.000000E+00 9.00000E+100
784 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.848721E-17 0.000000E+00 9.00000E+100
785 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.100916E-16 0.000000E+00 9.00000E+100
786 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -7.424781E-17 0.000000E+00 9.00000E+100
787 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.232761E-17 0.000000E+00 9.00000E+100
788 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.760606E-18 0.000000E+00 9.00000E+100
789 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.968418E-17 0.000000E+00 9.00000E+100
790 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.776397E-17 0.000000E+00 9.00000E+100
791 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.008317E-17 0.000000E+00 9.00000E+100
792 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.960943E-17 0.000000E+00 9.00000E+100
793 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.388946E-16 0.000000E+00 9.00000E+100
794 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.664175E-17 0.000000E+00 9.00000E+100
795 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.408148E-16 0.000000E+00 9.00000E+100
796 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.369744E-16 0.000000E+00 9.00000E+100
797 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.336667E-17 0.000000E+00 9.00000E+100
798 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.651374E-16 0.000000E+00 9.00000E+100
799 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.804990E-16 0.000000E+00 9.00000E+100
800 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.648384E-17 0.000000E+00 9.00000E+100
801 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.880303E-17 0.000000E+00 9.00000E+100
802 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.912728E-17 0.000000E+00 9.00000E+100
803 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.601010E-18 0.000000E+00 9.00000E+100
804 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.088115E-16 0.000000E+00 9.00000E+100
805 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.152963E-17 0.000000E+00 9.00000E+100
806 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.792189E-17 0.000000E+00 9.00000E+100
807 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.056532E-17 0.000000E+00 9.00000E+100
808 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.416465E-17 0.000000E+00 9.00000E+100
809 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 9.537004E-17 0.000000E+00 9.00000E+100
810 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.728182E-17 0.000000E+00 9.00000E+100
811 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.587367E-16 0.000000E+00 9.00000E+100
812 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.224445E-17 0.000000E+00 9.00000E+100
813 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.792189E-17 0.000000E+00 9.00000E+100
814 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.832930E-17 0.000000E+00 9.00000E+100
815 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.288451E-17 0.000000E+00 9.00000E+100
816 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.944310E-17 0.000000E+00 9.00000E+100
817 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.496263E-16 0.000000E+00 9.00000E+100
818 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.608485E-17 0.000000E+00 9.00000E+100
819 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.552795E-17 0.000000E+00 9.00000E+100
820 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.088957E-17 0.000000E+00 9.00000E+100
821 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.139320E-16 0.000000E+00 9.00000E+100
822 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.320876E-18 0.000000E+00 9.00000E+100
823 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.944310E-16 0.000000E+00 9.00000E+100
824 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.472997E-17 0.000000E+00 9.00000E+100
825 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.720707E-17 0.000000E+00 9.00000E+100
826 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.680808E-17 0.000000E+00 9.00000E+100
827 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.784714E-17 0.000000E+00 9.00000E+100
828 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.976734E-17 0.000000E+00 9.00000E+100
829 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.213138E-16 0.000000E+00 9.00000E+100
830 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.920202E-17 0.000000E+00 9.00000E+100
831 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.702579E-16 0.000000E+00 9.00000E+100
832 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.612970E-16 0.000000E+00 9.00000E+100
833 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.208653E-17 0.000000E+00 9.00000E+100
834 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
835 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.344141E-16 0.000000E+00 9.00000E+100
836 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.536162E-16 0.000000E+00 9.00000E+100
837 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.265838E-16 0.000000E+00 9.00000E+100
838 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.816296E-17 0.000000E+00 9.00000E+100
839 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.664175E-17 0.000000E+00 9.00000E+100
840 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.664175E-16 0.000000E+00 9.00000E+100
841 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.251542E-16 0.000000E+00 9.00000E+100
842 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.305737E-16 0.000000E+00 9.00000E+100
843 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 5.760606E-17 0.000000E+00 9.00000E+100
844 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.328350E-17 0.000000E+00 9.00000E+100
845 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.214633E-16 0.000000E+00 9.00000E+100
846 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.305737E-16 0.000000E+00 9.00000E+100
847 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.765091E-16 0.000000E+00 9.00000E+100
848 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.456364E-17 0.000000E+00 9.00000E+100
849 traj.phases.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.497758E-16 0.000000E+00 9.00000E+100
850 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.343609E-09 0.000000E+00 9.00000E+100
851 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.129766E-09 0.000000E+00 9.00000E+100
852 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.850614E-09 0.000000E+00 9.00000E+100
853 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.765927E-09 0.000000E+00 9.00000E+100
854 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.578329E-09 0.000000E+00 9.00000E+100
855 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.333265E-09 0.000000E+00 9.00000E+100
856 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.258871E-09 0.000000E+00 9.00000E+100
857 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.094001E-09 0.000000E+00 9.00000E+100
858 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.878440E-09 0.000000E+00 9.00000E+100
859 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.812956E-09 0.000000E+00 9.00000E+100
860 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.667758E-09 0.000000E+00 9.00000E+100
861 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.477737E-09 0.000000E+00 9.00000E+100
862 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.419975E-09 0.000000E+00 9.00000E+100
863 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.291813E-09 0.000000E+00 9.00000E+100
864 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.123929E-09 0.000000E+00 9.00000E+100
865 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.072854E-09 0.000000E+00 9.00000E+100
866 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.959469E-09 0.000000E+00 9.00000E+100
867 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.810798E-09 0.000000E+00 9.00000E+100
868 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.765542E-09 0.000000E+00 9.00000E+100
869 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.664996E-09 0.000000E+00 9.00000E+100
870 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.533032E-09 0.000000E+00 9.00000E+100
871 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.492826E-09 0.000000E+00 9.00000E+100
872 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.403471E-09 0.000000E+00 9.00000E+100
873 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.286073E-09 0.000000E+00 9.00000E+100
874 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.250281E-09 0.000000E+00 9.00000E+100
875 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.170681E-09 0.000000E+00 9.00000E+100
876 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.066008E-09 0.000000E+00 9.00000E+100
877 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.034072E-09 0.000000E+00 9.00000E+100
878 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.963009E-09 0.000000E+00 9.00000E+100
879 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.869480E-09 0.000000E+00 9.00000E+100
880 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.840920E-09 0.000000E+00 9.00000E+100
881 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.777350E-09 0.000000E+00 9.00000E+100
882 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.693597E-09 0.000000E+00 9.00000E+100
883 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.668012E-09 0.000000E+00 9.00000E+100
884 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.611018E-09 0.000000E+00 9.00000E+100
885 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.535876E-09 0.000000E+00 9.00000E+100
886 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.512897E-09 0.000000E+00 9.00000E+100
887 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.461705E-09 0.000000E+00 9.00000E+100
888 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.394154E-09 0.000000E+00 9.00000E+100
889 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.373493E-09 0.000000E+00 9.00000E+100
890 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.327411E-09 0.000000E+00 9.00000E+100
891 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.266567E-09 0.000000E+00 9.00000E+100
892 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.247946E-09 0.000000E+00 9.00000E+100
893 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.206403E-09 0.000000E+00 9.00000E+100
894 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.151503E-09 0.000000E+00 9.00000E+100
895 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.134690E-09 0.000000E+00 9.00000E+100
896 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.097171E-09 0.000000E+00 9.00000E+100
897 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.047549E-09 0.000000E+00 9.00000E+100
898 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.032347E-09 0.000000E+00 9.00000E+100
899 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.984016E-10 0.000000E+00 9.00000E+100
900 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.534782E-10 0.000000E+00 9.00000E+100
901 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.397087E-10 0.000000E+00 9.00000E+100
902 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.089447E-10 0.000000E+00 9.00000E+100
903 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.682114E-10 0.000000E+00 9.00000E+100
904 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.557201E-10 0.000000E+00 9.00000E+100
905 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.278011E-10 0.000000E+00 9.00000E+100
906 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.908120E-10 0.000000E+00 9.00000E+100
907 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.794599E-10 0.000000E+00 9.00000E+100
908 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.540900E-10 0.000000E+00 9.00000E+100
909 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.204480E-10 0.000000E+00 9.00000E+100
910 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.101184E-10 0.000000E+00 9.00000E+100
911 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.870301E-10 0.000000E+00 9.00000E+100
912 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.563945E-10 0.000000E+00 9.00000E+100
913 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.469843E-10 0.000000E+00 9.00000E+100
914 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.259420E-10 0.000000E+00 9.00000E+100
915 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.980067E-10 0.000000E+00 9.00000E+100
916 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.894248E-10 0.000000E+00 9.00000E+100
917 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.702220E-10 0.000000E+00 9.00000E+100
918 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.447196E-10 0.000000E+00 9.00000E+100
919 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.368805E-10 0.000000E+00 9.00000E+100
920 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.193364E-10 0.000000E+00 9.00000E+100
921 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.960267E-10 0.000000E+00 9.00000E+100
922 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.888583E-10 0.000000E+00 9.00000E+100
923 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.728115E-10 0.000000E+00 9.00000E+100
924 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.514800E-10 0.000000E+00 9.00000E+100
925 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.449182E-10 0.000000E+00 9.00000E+100
926 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.302255E-10 0.000000E+00 9.00000E+100
927 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.106841E-10 0.000000E+00 9.00000E+100
928 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.046728E-10 0.000000E+00 9.00000E+100
929 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.912039E-10 0.000000E+00 9.00000E+100
930 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.732857E-10 0.000000E+00 9.00000E+100
931 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.677740E-10 0.000000E+00 9.00000E+100
932 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.554130E-10 0.000000E+00 9.00000E+100
933 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.389668E-10 0.000000E+00 9.00000E+100
934 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.339041E-10 0.000000E+00 9.00000E+100
935 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.225558E-10 0.000000E+00 9.00000E+100
936 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.074460E-10 0.000000E+00 9.00000E+100
937 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.027907E-10 0.000000E+00 9.00000E+100
938 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.923607E-10 0.000000E+00 9.00000E+100
939 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.784674E-10 0.000000E+00 9.00000E+100
940 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.741847E-10 0.000000E+00 9.00000E+100
941 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.645912E-10 0.000000E+00 9.00000E+100
942 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.518059E-10 0.000000E+00 9.00000E+100
943 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.478644E-10 0.000000E+00 9.00000E+100
944 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.390301E-10 0.000000E+00 9.00000E+100
945 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.272553E-10 0.000000E+00 9.00000E+100
946 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.236257E-10 0.000000E+00 9.00000E+100
947 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.154863E-10 0.000000E+00 9.00000E+100
948 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.046338E-10 0.000000E+00 9.00000E+100
949 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.012904E-10 0.000000E+00 9.00000E+100
950 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.937827E-10 0.000000E+00 9.00000E+100
951 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.837742E-10 0.000000E+00 9.00000E+100
952 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.806895E-10 0.000000E+00 9.00000E+100
953 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.737638E-10 0.000000E+00 9.00000E+100
954 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.645269E-10 0.000000E+00 9.00000E+100
955 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.616756E-10 0.000000E+00 9.00000E+100
956 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.552866E-10 0.000000E+00 9.00000E+100
957 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.467573E-10 0.000000E+00 9.00000E+100
958 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.441261E-10 0.000000E+00 9.00000E+100
959 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.382221E-10 0.000000E+00 9.00000E+100
960 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.303420E-10 0.000000E+00 9.00000E+100
961 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.279107E-10 0.000000E+00 9.00000E+100
962 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.224530E-10 0.000000E+00 9.00000E+100
963 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.151691E-10 0.000000E+00 9.00000E+100
964 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.129207E-10 0.000000E+00 9.00000E+100
965 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.078757E-10 0.000000E+00 9.00000E+100
966 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.011378E-10 0.000000E+00 9.00000E+100
967 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.905852E-11 0.000000E+00 9.00000E+100
968 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.439203E-11 0.000000E+00 9.00000E+100
969 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.815833E-11 0.000000E+00 9.00000E+100
970 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.623440E-11 0.000000E+00 9.00000E+100
971 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.191597E-11 0.000000E+00 9.00000E+100
972 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.614612E-11 0.000000E+00 9.00000E+100
973 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.436696E-11 0.000000E+00 9.00000E+100
974 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.036697E-11 0.000000E+00 9.00000E+100
975 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.502583E-11 0.000000E+00 9.00000E+100
976 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.337651E-11 0.000000E+00 9.00000E+100
977 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.967373E-11 0.000000E+00 9.00000E+100
978 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.472387E-11 0.000000E+00 9.00000E+100
979 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.319936E-11 0.000000E+00 9.00000E+100
980 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.976754E-11 0.000000E+00 9.00000E+100
981 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.518408E-11 0.000000E+00 9.00000E+100
982 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.377007E-11 0.000000E+00 9.00000E+100
983 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.059016E-11 0.000000E+00 9.00000E+100
984 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.634398E-11 0.000000E+00 9.00000E+100
985 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.503103E-11 0.000000E+00 9.00000E+100
986 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.208659E-11 0.000000E+00 9.00000E+100
987 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.815067E-11 0.000000E+00 9.00000E+100
988 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 1.420045E-09 0.000000E+00 9.00000E+100
989 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.420120E-11 0.000000E+00 9.00000E+100
990 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.054580E-11 0.000000E+00 9.00000E+100
991 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.941677E-11 0.000000E+00 9.00000E+100
992 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.688252E-11 0.000000E+00 9.00000E+100
993 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.349543E-11 0.000000E+00 9.00000E+100
994 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.244836E-11 0.000000E+00 9.00000E+100
995 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.009904E-11 0.000000E+00 9.00000E+100
996 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.958558E-12 0.000000E+00 9.00000E+100
997 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.990176E-12 0.000000E+00 9.00000E+100
998 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.810161E-12 0.000000E+00 9.00000E+100
999 traj.phases.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.991066E-13 0.000000E+00 9.00000E+100
1000 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.380356E-17 0.000000E+00 9.00000E+100
1001 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.000947E-18 0.000000E+00 9.00000E+100
1002 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.480156E-17 0.000000E+00 9.00000E+100
1003 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.400147E-18 0.000000E+00 9.00000E+100
1004 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.400779E-18 0.000000E+00 9.00000E+100
1005 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.220655E-17 0.000000E+00 9.00000E+100
1006 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.400253E-18 0.000000E+00 9.00000E+100
1007 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.800189E-18 0.000000E+00 9.00000E+100
1008 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.960312E-17 0.000000E+00 9.00000E+100
1009 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.440152E-17 0.000000E+00 9.00000E+100
1010 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -7.400779E-18 0.000000E+00 9.00000E+100
1011 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.720286E-17 0.000000E+00 9.00000E+100
1012 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.000947E-18 0.000000E+00 9.00000E+100
1013 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.000316E-18 0.000000E+00 9.00000E+100
1014 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -7.200758E-18 0.000000E+00 9.00000E+100
1015 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.920518E-17 0.000000E+00 9.00000E+100
1016 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.380251E-17 0.000000E+00 9.00000E+100
1017 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.760291E-17 0.000000E+00 9.00000E+100
1018 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.100221E-17 0.000000E+00 9.00000E+100
1019 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 6.000632E-19 0.000000E+00 9.00000E+100
1020 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.140120E-17 0.000000E+00 9.00000E+100
1021 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.801031E-18 0.000000E+00 9.00000E+100
1022 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.400463E-18 0.000000E+00 9.00000E+100
1023 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.300347E-17 0.000000E+00 9.00000E+100
1024 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.740288E-17 0.000000E+00 9.00000E+100
1025 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -7.000737E-18 0.000000E+00 9.00000E+100
1026 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.200232E-17 0.000000E+00 9.00000E+100
1027 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 8.000842E-18 0.000000E+00 9.00000E+100
1028 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.600168E-18 0.000000E+00 9.00000E+100
1029 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.200547E-18 0.000000E+00 9.00000E+100
1030 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.680808E-17 0.000000E+00 9.00000E+100
1031 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.600274E-18 0.000000E+00 9.00000E+100
1032 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.740604E-17 0.000000E+00 9.00000E+100
1033 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.040425E-17 0.000000E+00 9.00000E+100
1034 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.840194E-17 0.000000E+00 9.00000E+100
1035 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.460154E-17 0.000000E+00 9.00000E+100
1036 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.220444E-17 0.000000E+00 9.00000E+100
1037 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.200968E-18 0.000000E+00 9.00000E+100
1038 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.000316E-18 0.000000E+00 9.00000E+100
1039 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 4.260448E-17 0.000000E+00 9.00000E+100
1040 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 9.000947E-18 0.000000E+00 9.00000E+100
1041 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.600168E-18 0.000000E+00 9.00000E+100
1042 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 3.000316E-18 0.000000E+00 9.00000E+100
1043 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.800295E-17 0.000000E+00 9.00000E+100
1044 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.200653E-18 0.000000E+00 9.00000E+100
1045 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.820297E-17 0.000000E+00 9.00000E+100
1046 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.540162E-17 0.000000E+00 9.00000E+100
1047 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.380145E-17 0.000000E+00 9.00000E+100
1048 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.960627E-17 0.000000E+00 9.00000E+100
1049 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.400147E-18 0.000000E+00 9.00000E+100
1050 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.400253E-18 0.000000E+00 9.00000E+100
1051 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.700284E-17 0.000000E+00 9.00000E+100
1052 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.220234E-17 0.000000E+00 9.00000E+100
1053 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 4.500474E-17 0.000000E+00 9.00000E+100
1054 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.900726E-17 0.000000E+00 9.00000E+100
1055 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.020213E-17 0.000000E+00 9.00000E+100
1056 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-17 0.000000E+00 9.00000E+100
1057 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.060112E-17 0.000000E+00 9.00000E+100
1058 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 5.600589E-18 0.000000E+00 9.00000E+100
1059 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.800295E-18 0.000000E+00 9.00000E+100
1060 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -8.200863E-18 0.000000E+00 9.00000E+100
1061 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.400147E-17 0.000000E+00 9.00000E+100
1062 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.600168E-17 0.000000E+00 9.00000E+100
1063 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.060217E-17 0.000000E+00 9.00000E+100
1064 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.540162E-17 0.000000E+00 9.00000E+100
1065 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.200442E-18 0.000000E+00 9.00000E+100
1066 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.760185E-17 0.000000E+00 9.00000E+100
1067 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.820192E-17 0.000000E+00 9.00000E+100
1068 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 5.200547E-18 0.000000E+00 9.00000E+100
1069 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.260554E-17 0.000000E+00 9.00000E+100
1070 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.200758E-18 0.000000E+00 9.00000E+100
1071 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.800716E-18 0.000000E+00 9.00000E+100
1072 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 5.000526E-18 0.000000E+00 9.00000E+100
1073 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.000421E-19 0.000000E+00 9.00000E+100
1074 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.820297E-17 0.000000E+00 9.00000E+100
1075 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.840194E-17 0.000000E+00 9.00000E+100
1076 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.200232E-18 0.000000E+00 9.00000E+100
1077 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-17 0.000000E+00 9.00000E+100
1078 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.800189E-18 0.000000E+00 9.00000E+100
1079 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.400253E-18 0.000000E+00 9.00000E+100
1080 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-17 0.000000E+00 9.00000E+100
1081 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.340141E-17 0.000000E+00 9.00000E+100
1082 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.800505E-18 0.000000E+00 9.00000E+100
1083 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.000211E-18 0.000000E+00 9.00000E+100
1084 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.600589E-18 0.000000E+00 9.00000E+100
1085 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.200758E-18 0.000000E+00 9.00000E+100
1086 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.600695E-18 0.000000E+00 9.00000E+100
1087 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.000632E-18 0.000000E+00 9.00000E+100
1088 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.000632E-19 0.000000E+00 9.00000E+100
1089 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.700179E-17 0.000000E+00 9.00000E+100
1090 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 3.800400E-18 0.000000E+00 9.00000E+100
1091 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-18 0.000000E+00 9.00000E+100
1092 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.801031E-18 0.000000E+00 9.00000E+100
1093 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.400779E-18 0.000000E+00 9.00000E+100
1094 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-18 0.000000E+00 9.00000E+100
1095 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.040109E-17 0.000000E+00 9.00000E+100
1096 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 8.800926E-18 0.000000E+00 9.00000E+100
1097 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.400147E-18 0.000000E+00 9.00000E+100
1098 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.200547E-18 0.000000E+00 9.00000E+100
1099 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.340141E-17 0.000000E+00 9.00000E+100
1100 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.220128E-17 0.000000E+00 9.00000E+100
1101 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.200758E-18 0.000000E+00 9.00000E+100
1102 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.400253E-18 0.000000E+00 9.00000E+100
1103 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -8.400884E-18 0.000000E+00 9.00000E+100
1104 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.120118E-17 0.000000E+00 9.00000E+100
1105 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.570271E-17 0.000000E+00 9.00000E+100
1106 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-19 0.000000E+00 9.00000E+100
1107 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.600695E-18 0.000000E+00 9.00000E+100
1108 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.690178E-17 0.000000E+00 9.00000E+100
1109 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.000632E-18 0.000000E+00 9.00000E+100
1110 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.700705E-18 0.000000E+00 9.00000E+100
1111 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.600589E-18 0.000000E+00 9.00000E+100
1112 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.800295E-18 0.000000E+00 9.00000E+100
1113 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.800610E-18 0.000000E+00 9.00000E+100
1114 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.501000E-18 0.000000E+00 9.00000E+100
1115 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.600484E-18 0.000000E+00 9.00000E+100
1116 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.200337E-18 0.000000E+00 9.00000E+100
1117 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.350142E-17 0.000000E+00 9.00000E+100
1118 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 4.500474E-18 0.000000E+00 9.00000E+100
1119 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -8.500895E-18 0.000000E+00 9.00000E+100
1120 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.501000E-18 0.000000E+00 9.00000E+100
1121 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.800295E-18 0.000000E+00 9.00000E+100
1122 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
1123 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.350142E-17 0.000000E+00 9.00000E+100
1124 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.500368E-18 0.000000E+00 9.00000E+100
1125 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.400147E-18 0.000000E+00 9.00000E+100
1126 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.600589E-18 0.000000E+00 9.00000E+100
1127 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.500368E-18 0.000000E+00 9.00000E+100
1128 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.000211E-19 0.000000E+00 9.00000E+100
1129 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 8.000842E-19 0.000000E+00 9.00000E+100
1130 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.900410E-18 0.000000E+00 9.00000E+100
1131 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -8.000842E-19 0.000000E+00 9.00000E+100
1132 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 3.400358E-18 0.000000E+00 9.00000E+100
1133 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.000421E-18 0.000000E+00 9.00000E+100
1134 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.300137E-18 0.000000E+00 9.00000E+100
1135 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.100116E-18 0.000000E+00 9.00000E+100
1136 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.800189E-18 0.000000E+00 9.00000E+100
1137 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.500579E-18 0.000000E+00 9.00000E+100
1138 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.500158E-18 0.000000E+00 9.00000E+100
1139 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.000211E-19 0.000000E+00 9.00000E+100
1140 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.000421E-19 0.000000E+00 9.00000E+100
1141 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.000105E-19 0.000000E+00 9.00000E+100
1142 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.700179E-18 0.000000E+00 9.00000E+100
1143 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
1144 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.600168E-18 0.000000E+00 9.00000E+100
1145 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.000421E-19 0.000000E+00 9.00000E+100
1146 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -9.000947E-19 0.000000E+00 9.00000E+100
1147 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.100116E-18 0.000000E+00 9.00000E+100
1148 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.300137E-18 0.000000E+00 9.00000E+100
1149 traj.phases.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.100116E-18 0.000000E+00 9.00000E+100
Exit Status
Inform Description
0 Solve Succeeded
--------------------------------------------------------------------------------
/usr/share/miniconda/envs/test/lib/python3.10/site-packages/openmdao/recorders/sqlite_recorder.py:227: UserWarning:The existing case recorder file, dymos_simulation.db, is being overwritten.
Simulating trajectory traj
Model viewer data has already been recorded for Driver.
Done simulating trajectory traj
Launch angle: 46.0919 deg
Flight angle at end of propulsion: 38.3884 deg
Empty mass: 0.1891 kg
Water volume: 1.0265 L
Maximum range: 85.1114 m
Maximum height: 23.0863 m
Maximum velocity: 41.3116 m/s
Maximum Range Solution: Propulsive Phase#
plot_propelled_ascent(sol_out, sim_out)
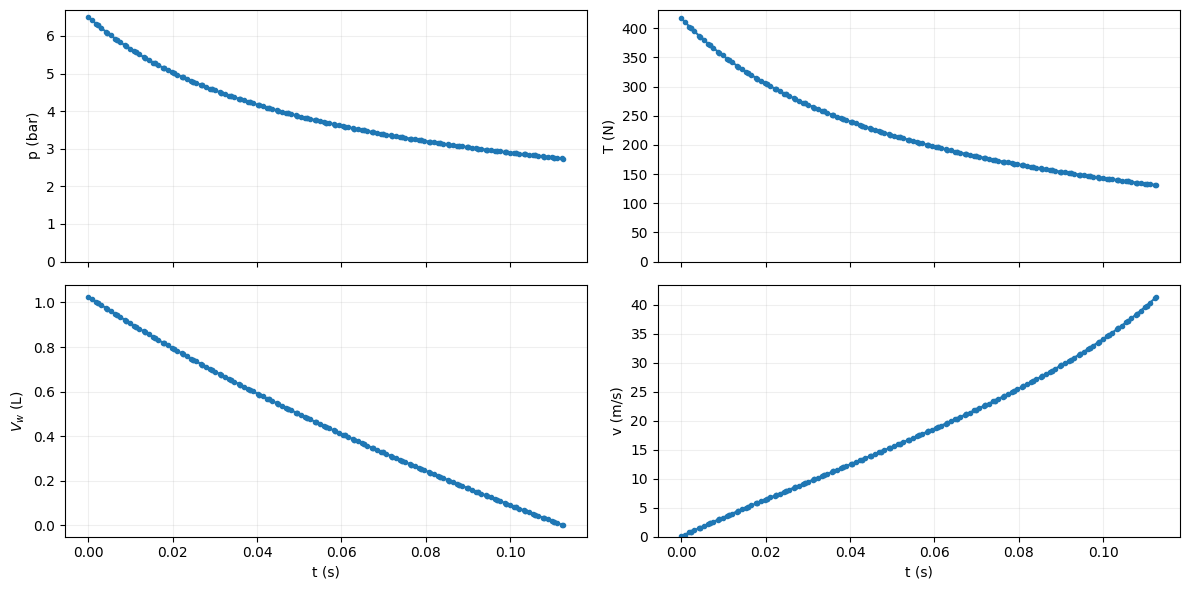
Maximum Range Solution: Height vs. Range#
plot_trajectory(sol_out, sim_out, legend_loc='center')
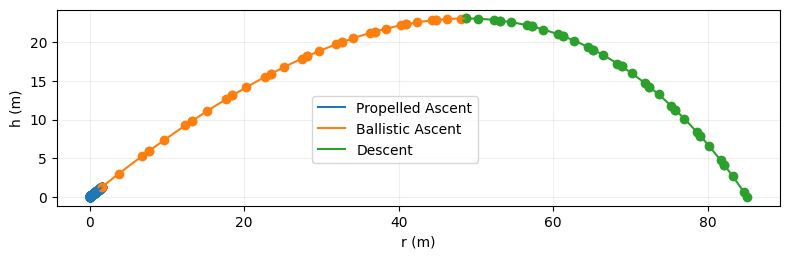
Maximum Range Solution: State History#
plot_states(sol_out, sim_out, legend_loc='lower center')
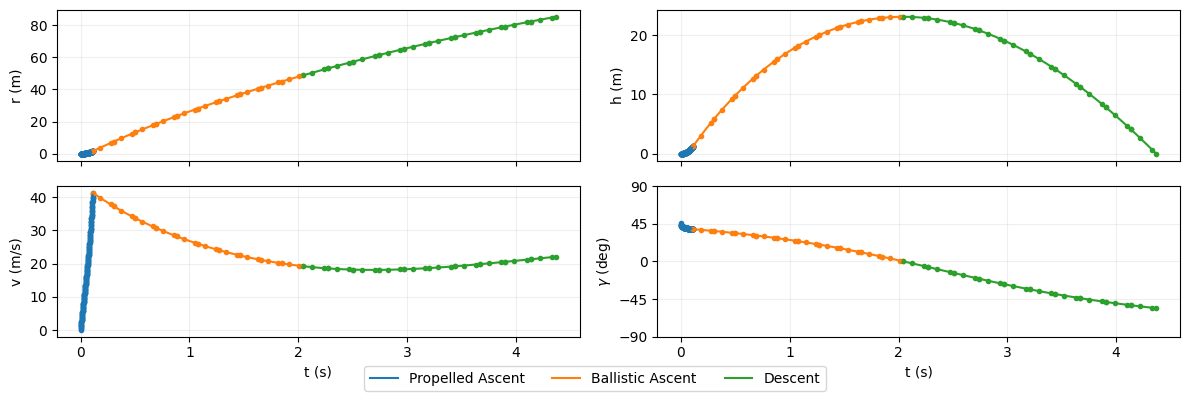
References#
Nozzles. 2013. URL: http://www.aircommandrockets.com/nozzles.htm.
Bernardo Bahia Monteiro. Optimization of a water rocket in openmdao/dymos. Aug 2020. URL: engrxiv.org/nqta8, doi:10.31224/osf.io/nqta8.
R Barrio-Perotti, E Blanco-Marigorta, K Argüelles-D\'ıaz, and J Fernández-Oro. Experimental evaluation of the drag coefficient of water rockets by a simple free-fall test. European Journal of Physics, 30(5):1039–1048, jul 2009. doi:10.1088/0143-0807/30/5/012.
Laura Fischer, Thomas Günther, Linda Herzig, Tobias Jarzina, Frank Klinker, Sabine Knipper, Franz-Georg Schürmann, and Marvin Wollek. On the approximation of D.I.Y. water rocket dynamics including air drag. arXiv:2001.08828, 2020. arXiv:2001.08828.
Alejandro Romanelli, Italo Bove, and Federico González Madina. Air expansion in a water rocket. American Journal of Physics, 81(10):762–766, oct 2013. doi:10.1119/1.4811116.
Glen E. Thorncroft, John R. Ridgely, and Christopher C. Pascual. Hydrodynamics and thrust characteristics of a water-propelled rocket. International Journal of Mechanical Engineering Education, 37(3):241–261, jul 2009. doi:10.7227/ijmee.37.3.9.